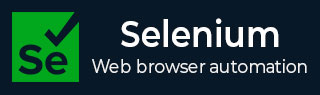
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver 与 RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 Iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 多选
Selenium Webdriver 可以使用 Select 类在网页上的下拉菜单中进行多选操作。网页上的下拉菜单可以分为两种类型 - 单选和多选。
多选下拉菜单由名为 select 的标签名标识。此外,其每个选项都由名为 option 的标签名标识。除此之外,多选下拉菜单应具有一个名为 multiple 的属性。
HTML 中多选下拉菜单的识别
右键单击网页,然后在 Chrome 浏览器中单击“检查”按钮,结果将显示整个页面的 HTML 代码。要检查页面上的多选下拉菜单,请单击可见 HTML 代码顶部可用的向左向上箭头,如下所示。
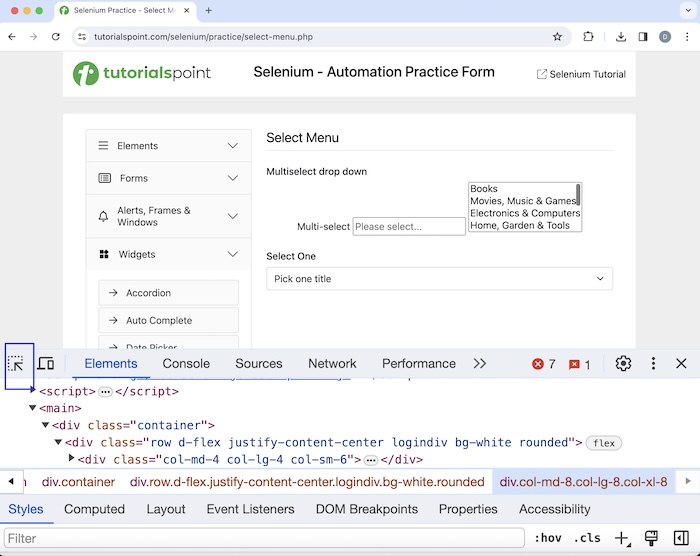
一旦我们单击并将箭头指向文本多选下拉菜单旁边的下拉菜单,其 HTML 代码就会可见,反映了 select 标签名(括在<>中),以及 option 标签名内的选项及其属性 multiple。
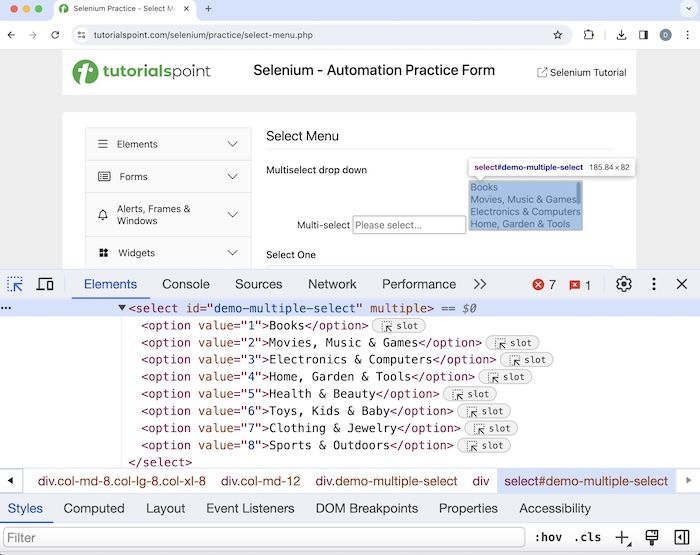
Select 中的基本多选方法
Selenium webdriver 的 Select 类中提供了多种方法,可以帮助我们处理这两种多选下拉菜单。
示例
让我们再举一个以下页面的例子,在这里我们将访问文本多选下拉菜单旁边的多选下拉菜单,选择值电影、音乐和游戏、电子产品和电脑以及书籍,并使用上面讨论的方法执行一些验证。
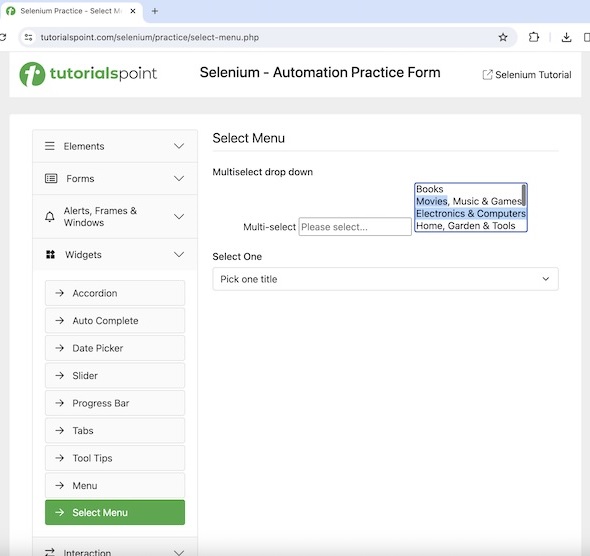
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class HandlingMultDropdown { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://tutorialspoint.com/selenium/practice/select-menu.php"); // identify multiple dropdown WebElement dropdown = driver.findElement(By.xpath("//*[@id='demo-multiple-select']")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by visible text select.selectByVisibleText("Books"); // select item by value select.selectByValue("2"); // select item by index select.selectByIndex(2); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(2); // get all selected options of dropdown after deselecting 1 List<WebElement> deletedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected after deselecting one option: " + deletedOptions.size()); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> deletedAllOptions = select.getAllSelectedOptions(); System.out.println("No. options selected after deselecting all: " + deletedAllOptions.size()); // Closing browser driver.quit(); } }
输出
Options are: Books Options are: Movies, Music & Games Options are: Electronics & Computers Options are: Home, Garden & Tools Options are: Health & Beauty Options are: Toys, Kids & Baby Options are: Clothing & Jewelry Options are: Sports & Outdoors Boolean value for multiple dropdown: true Selected Options are: Books Selected Options are: Movies, Music & Games Selected Options are: Electronics & Computers First selected option is: Books No. options selected after deselecting one option: 2 No. options selected after deselecting all: 0 Process finished with exit code 0
在上面的示例中,我们使用控制台中的消息获取了下拉菜单的所有选项 - 选项为:书籍,选项为:电影、音乐和游戏,选项为:家居、园艺和工具,选项为:健康和美容,选项为:玩具、儿童和婴儿,选项为:服装和珠宝,选项为:运动和户外。
我们还使用控制台中的消息验证了下拉菜单具有多个选择选项 - 用于检查的布尔值为:true。我们使用控制台中的消息检索了下拉菜单中的选定选项 - 选定的选项为:书籍,选定的选项为:电影、音乐和游戏,以及选定的选项为:电子产品和电脑。
我们还使用控制台中的消息获取了第一个选定的选项 - 第一个选定的选项为:书籍。取消选择一个选定的选项后,我们使用控制台中的消息收到了选定选项的数量 - 取消选择一个选项后选定的选项数:2。
最后,我们取消选择了下拉菜单中所有选定的选项,因此在控制台中获得了消息 - 取消选择所有选项后选定的选项数:0。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
这总结了我们对 Selenium Webdriver - 多选教程的全面介绍。我们从描述 HTML 中多选下拉菜单的识别、Select 中的基本多选方法以及一个示例来说明如何在 Selenium Webdriver 中处理多选下拉菜单开始。
这为您提供了 Selenium Webdriver - 多选的深入知识。明智的做法是不断练习您所学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并拓宽您的视野。