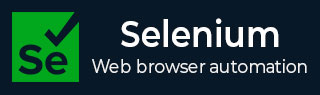
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码生成
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 与其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium - 数据驱动框架
Selenium Webdriver 可用于开发基于数据驱动框架的测试脚本。数据驱动框架主要用于创建依赖于外部数据源数据的测试用例。此外部数据源可以是任何具有 .txt、.properties、.xlsx、.xls、.csv 扩展名的文件,也可以借助数据提供者。
数据驱动框架是一种将测试用例与数据集分离的框架。此外,它还提供了针对多个数据集运行同一测试用例的功能。由于测试代码实现和测试数据彼此独立,因此可以在不影响实现逻辑的情况下修改数据集,反之亦然。
为什么要使用数据驱动测试框架?
在数据驱动测试框架中,可以针对同一测试用例运行多个数据集,因此可以在无需额外代码的情况下针对广泛的数据范围测试同一应用程序。因此,代码只需开发一次即可重复使用多次。
数据驱动测试框架允许多次执行测试用例,而无需增加测试用例的数量。有时,测试数据会自动生成,这允许应用程序针对广泛的随机数据集进行测试。在这种情况下,数据驱动测试框架确保产品更健壮和高质量。
数据驱动测试框架的优势
数据驱动测试框架通过确保更结构化的执行来实现测试用例的最佳利用。这也有助于缩短测试周期,同时确保产品质量。包含各种函数、方法和操作的测试实现代码在数据驱动测试框架中使用广泛的数据集进行测试。
数据驱动测试框架与关键字驱动测试框架的区别
数据驱动框架和关键字驱动框架之间存在一些差异。关键字驱动框架更容易维护,因为在测试数据、关键字和实现逻辑层之间存在更多抽象,而数据驱动框架比关键字驱动框架更难以维护,因为抽象只存在于测试数据和测试脚本之间。
与数据驱动框架相比,关键字驱动框架需要更系统的规划,因为在数据驱动框架中,我们只是将测试数据与测试脚本分离。
示例 1 - 使用 Excel 进行数据驱动
让我们举一个使用 Apache POI 库使用 Excel 执行数据驱动测试的例子。让我们以名为 **DetailsStudent.xlsx** 的 Excel 文件为例,我们将从该 Excel 文件中读取值并将这些数据输入到下面的注册页面,成功后,我们将在单元格(同一行和 E 列)中写入文本 **测试用例:通过**。如果未成功,我们将在同一单元格中写入文本 **测试用例:失败**。
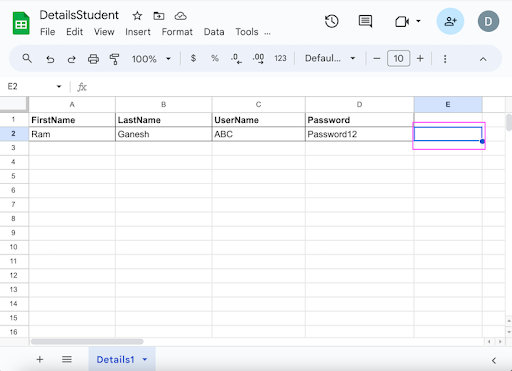
下图显示了注册页面,我们将在此页面中从 **DetailsStudent.xlsx** 文件中输入 **全名:**、**姓氏:**、**用户名:** 和 **密码** 字段中的数据。 成功后,我们将在单元格(同一行和 E 列)中写入文本 **测试用例:通过**。如果未成功,我们将在同一单元格中写入文本 **测试用例:失败**。
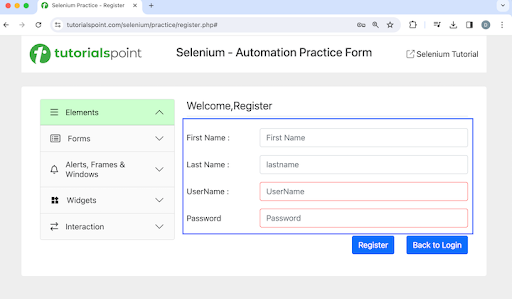
**请注意** - **DetailsStudent.xlsx** Excel 文件放置在项目下的 **Resources** 文件夹中,如下图所示。
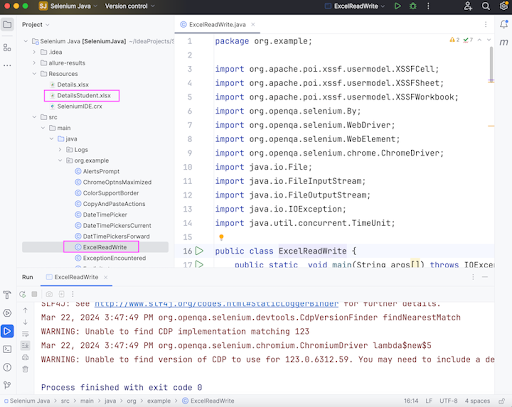
如何安装 Apache POI?
**步骤 1** - 从以下链接添加 Apache POI Common 依赖项 -
https://mvnrepository.com/artifact/org.apache.poi/poi.
**步骤 2** - 从以下链接添加基于 OPC 和 OOXML 架构的 Apache POI API 依赖项 -
https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml.
**步骤 3** - 保存包含所有依赖项的 pom.xml 并更新 Maven 项目。
**ExcelReadWrite.java** 中的代码实现
package org.example; import org.apache.poi.xssf.usermodel.XSSFCell; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.concurrent.TimeUnit; public class ExcelReadWrite { public static void main(String args[]) throws IOException { // identify location of .xlsx file File f = new File("./Resources/DetailsStudent.xlsx"); FileInputStream i = new FileInputStream(f); // instance of XSSFWorkbook XSSFWorkbook w = new XSSFWorkbook(i); // create sheet in XSSFWorkbook with name Details1 XSSFSheet s = w .getSheet("Details1"); // handle total rows in XSSFSheet int r = s.getLastRowNum() - s.getFirstRowNum(); // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); // Opening the webpage where we will identify elements driver.get("https://tutorialspoint.com/selenium/practice/register.php#"); //Identify elements for registration WebElement fname = driver.findElement(By.xpath("//*[@id='firstname']")); WebElement lname = driver.findElement(By.xpath("//*[@id='lastname']")); WebElement uname = driver.findElement(By.xpath("//*[@id='username']")); WebElement pass = driver.findElement(By.xpath("//*[@id='password']")); WebElement btn = driver.findElement(By.xpath("//*[@id='signupForm']/div[5]/input")); // loop through rows, read and enter values in form for(int j = 1; j <= r; j++) { fname.sendKeys(s.getRow(j).getCell(0).getStringCellValue()); lname.sendKeys(s.getRow(j).getCell(1).getStringCellValue()); uname.sendKeys(s.getRow(j).getCell(2).getStringCellValue()); pass.sendKeys(s.getRow(j).getCell(3).getStringCellValue()); // submit registration form btn.click(); // verify form submitted WebElement fname1 = driver.findElement(By.xpath("//*[@id='firstname']")); String value = fname1.getAttribute("value"); // create cell at Column 4 to write values in excel XSSFCell c = s.getRow(j).createCell(4); // write results in excel if (value.isEmpty()) { c.setCellValue("Test Case: PASS"); } else { c.setCellValue("Test Case: FAIL"); } // complete writing value in excel FileOutputStream o = new FileOutputStream("./Resources/DetailsStudent.xlsx"); w.write(o); } // closing workbook object w.close(); // Quitting browser driver.quit(); } }
添加到 pom.xml 的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>5.2.5</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>5.2.5</version> </dependency> </dependencies> </project>
输出
Process finished with exit code 0
在上面的示例中,我们读取了整个 Excel 文件,并在第五列的单元格中写入值 **测试用例:通过**。
最后,收到消息 **Process finished with exit code 0**,表示代码成功执行。
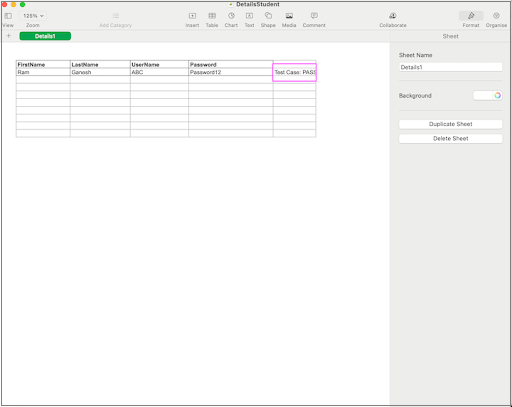
如上图所示,在测试运行后,**测试用例:通过** 被写入 **DetailsStudent.xlsx** Excel 文件的第 5 列中,这与 Excel 中提供的注册数据有关。
示例 2 - 使用 TestNG DataProvider 进行数据驱动
让我们举一个使用 TestNG 的默认功能(称为 DataProvider)进行数据驱动测试的例子。DataProvider 用于向任何测试方法提供数据。
我们将使用 TestNG DataProvider 在与前面示例相同的注册页面中输入值,在该页面中,我们将使用 TestNG DataProvider 输入 **全名:**、**姓氏:**、**用户名:** 和 **密码** 字段中的数据。
如何安装 TestNG?
在系统中安装 Java(版本高于 8),并使用命令:java -version 检查它是否存在。如果安装已成功完成,则将显示已安装的 Java 版本。
在系统中安装 Maven,并使用命令:mvn -version 检查它是否存在。如果安装已成功完成,则将显示已安装的 Maven 版本。
安装任何 IDE,例如 Eclipse、IntelliJ 等。
从以下链接添加 TestNG 依赖项:https://mvnrepository.com/artifact/org.testng/testng。
**DataTest.java** 中的代码实现
package CrossBrw; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.*; import java.util.concurrent.TimeUnit; public class DataTest { WebDriver driver; @BeforeTest public void setup() throws Exception { // Initiate the Webdriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/register.php#"); } @Test(dataProvider = "registration-data") public void userRegistration (String fname1, String lname1, String uname1, String pass1) { //Identify elements for registration WebElement fname = driver.findElement(By.xpath("//*[@id='firstname']")); WebElement lname = driver.findElement(By.xpath("//*[@id='lastname']")); WebElement uname = driver.findElement(By.xpath("//*[@id='username']")); WebElement pass = driver.findElement(By.xpath("//*[@id='password']")); // enter details from data provider fname.sendKeys(fname1); lname.sendKeys(lname1); uname.sendKeys(uname1); pass.sendKeys(pass1); // get values entered String text = fname.getAttribute("value"); String text1 = lname.getAttribute("value"); String text2 = uname.getAttribute("value"); String text3 = pass.getAttribute("value"); System.out.println("Entered first name: " + text); System.out.println("Entered last name: " + text1); System.out.println("Entered username: " + text2); System.out.println("Entered password: " + text3); } @DataProvider (name = "registration-data") public Object[][] registrationData(){ return new Object[][] { {"Ram", "Ganesh", "ABC", "Password12"} }; } @AfterTest public void teardown() { // quitting browser driver.quit(); } }
添加到 pom.xml 的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.testng/testng --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.9.0</version> <scope>test</scope> </dependency> </dependencies> </project>
输出
Entered first name: Ram Entered last name: Ganesh Entered username: ABC Entered password: Password12 =============================================== Default Suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
在上面的示例中,我们从 DataProvider 中提供的数据中在注册页面输入数据,并在控制台中获得输入的值以及消息 - **输入的姓名:Ram,输入的姓氏:Ganesh,输入的用户名:ABC** 和 **输入的密码:Password12**。
控制台中的结果显示 **Total tests run: 1**,因为只有一个方法带有 @Test 注解 - userRegisterration()。
最后,收到消息 **Passes: 1** 和 **Process finished with exit code 0**,表示代码成功执行。
本教程全面介绍了 Selenium Webdriver - 数据驱动框架。我们首先介绍什么是数据驱动框架,为什么要使用数据驱动框架,数据驱动框架的优势是什么,数据驱动框架与关键字驱动框架的区别是什么,并通过示例演示了如何使用 Excel 和 TestNG 中的 DataProvider 以及 Selenium Webdriver 实现数据驱动框架。
这使您掌握了 Selenium Webdriver 中数据驱动框架的深入知识。明智的做法是继续练习您所学到的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并扩展您的视野。