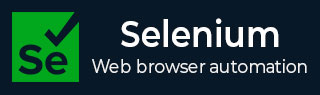
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 基于关键字的框架
- Selenium - 基于数据的框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 文件上传
Selenium WebDriver 可用于自动化需要在网页上上传文件的测试。在 HTML 中,文件上传元素由名为input的标签名称标识。此外,它应该有一个属性type,其值为file。
在 HTML 中识别上传功能
右键单击下面显示的页面,然后单击 Chrome 浏览器中的“检查”按钮。结果,整个页面的完整 HTML 代码将可见。要检查页面上的“选择文件”按钮,请单击左侧向上的箭头,如下所示。
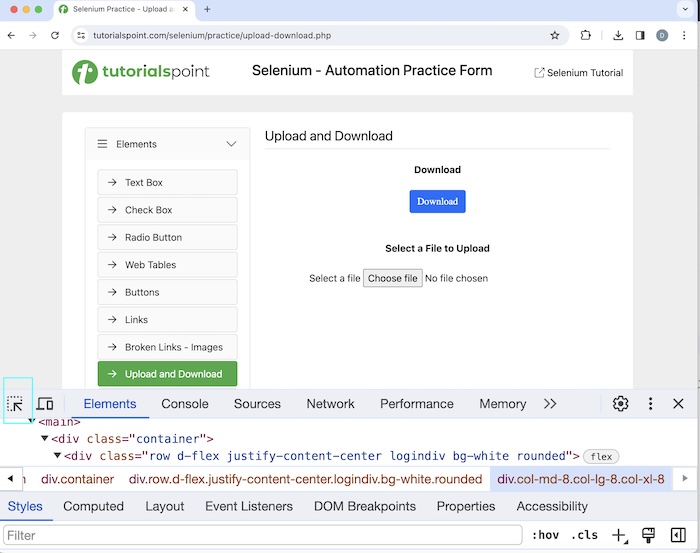
单击并将箭头指向“选择文件”文本旁边的按钮后,其 HTML 代码可见,反映了input标签名称及其值为file的type属性。
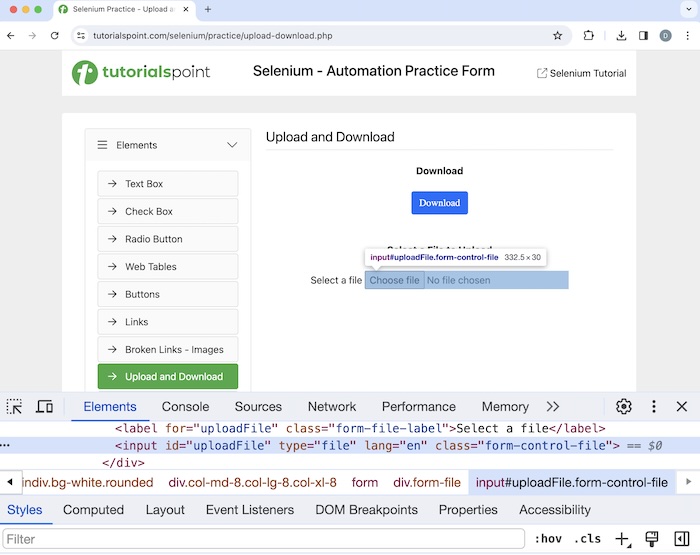
<input id="uploadFile" type="file" lang="en" class="form-control-file">
要上传文件,我们将使用sendKeys()方法。要上传的文件路径作为参数传递给该方法。
示例
让我们以下面的页面为例,我们将通过单击“选择文件”按钮上传文件Picture.png。成功上传后,文件Picture.png应该在网页上可见。
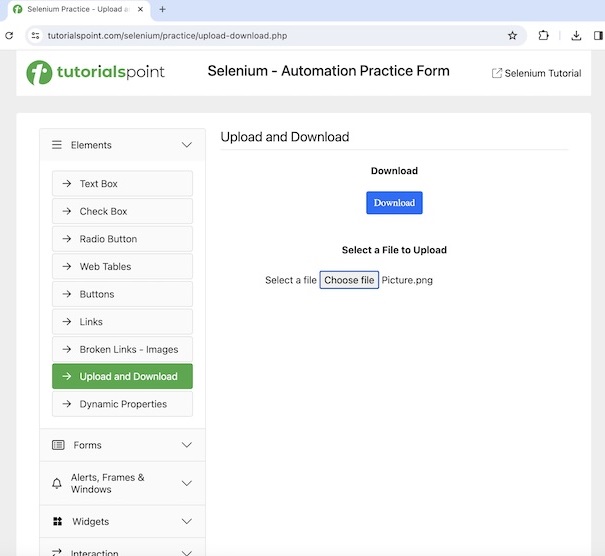
语法
WebDriver driver = new ChromeDriver(); // identify element the element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // getting file path to be uploaded File f = new File("./Picture.png"); System.out.println("Getting the file path to be uploaded: " + f.getAbsolutePath()); // uploading file with path of file uploaded m.sendKeys(f.getAbsolutePath());
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.util.concurrent.TimeUnit; public class FilesUpload { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will upload a file driver.get("https://tutorialspoint.com/selenium/practice/upload-download.php"); // identify element with xpath for file upload WebElement m = driver.findElement(By.xpath("//*[@id='uploadFile']")); // getting file path to be uploaded File f = new File("./Picture.png"); System.out.println("Getting the file path to be uploaded: " + f.getAbsolutePath()); // uploading file with path of file uploaded m.sendKeys(f.getAbsolutePath()); // check if file uploaded successfully if (m.getAttribute("value").equalsIgnoreCase("Picture.png")) { System.out.println("File uploaded successfully "); } else { System.out.println("File uploaded unsuccessfully "); } // Closing browser driver.quit(); } }
在 pom.xml 文件中添加的依赖项:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
输出
Getting the file path to be uploaded: /Users/IdeaProjects/Selenium Java/./Picture.png File uploaded unsuccessfully Process finished with exit code 0
在上面的示例中,我们获得了要上传的文件路径以及控制台中的消息 - 正在获取要上传的文件路径:/Users/IdeaProjects/Selenium Java/./Picture.png。然后我们成功上传了文件,控制台中的消息为 - 文件上传失败。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
文件路径错误的异常
让我们再举一个例子,其中将错误的文件路径作为参数传递给 sendKeys() 方法(即要上传的文件名为Picture1.png而不是 Picture.png)。在这种情况下,文件将不会上传,并且会抛出异常。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.util.concurrent.TimeUnit; public class FilesExcUpload { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will upload a file driver.get("https://tutorialspoint.com/selenium/practice/upload-download.php"); // identify element with xpath for file upload WebElement m = driver.findElement(By.xpath("//*[@id='uploadFile']")); // getting file path to be uploaded File f = new File("./Picture1.png"); System.out.println("Getting the file path to be uploaded: " + f.getAbsolutePath()); // uploading file with path of file uploaded m.sendKeys(f.getAbsolutePath()); // check if file uploaded successfully if (m.getAttribute("value").equalsIgnoreCase("Picture1.png")) { System.out.println("File uploaded successfully "); } else { System.out.println("File uploaded unsuccessfully "); } // Closing browser driver.quit(); } }
输出
Getting the file path to be uploaded: /Users/IdeaProjects/Selenium Java/./Picture1.png Exception in thread "main" org.openqa.selenium.InvalidArgumentException: invalid argument: File not found : /Users/IdeaProjects/Selenium Java/./Picture1.png Process finished with exit code 1
在上面的示例中,我们获得了要上传的文件路径以及控制台中的消息 - 正在获取要上传的文件路径:/Users/IdeaProjects/Selenium Java/./Picture1.png。然后由于发送了错误的文件路径进行上传,因此收到异常。
最后,收到消息进程已完成,退出代码为 1,表示代码执行失败。
结论
本教程全面介绍了 Selenium WebDriver 文件上传。我们从描述如何在 HTML 中识别上传功能开始,并通过示例来说明如何在 Selenium WebDriver 中上传文件。这使您深入了解 Selenium WebDriver 文件上传。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。