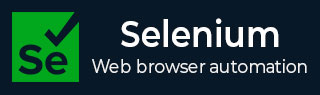
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键盘向上/向下键
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 键盘向上/向下键
Selenium WebDriver 可以借助 **Actions 类** 执行键盘向上和键盘向下操作。 使用 keyUp() 和 keyDown() 方法执行键盘向上和键盘向下操作。
复制和粘贴操作可以通过在 Selenium 中使用 Keys 类来执行。 用于复制和粘贴的键可以使用 Ctrl + C 和 Ctrl + V 分别完成。 要按下的这些键作为参数发送到 sendKeys() 方法。
网页上元素的识别
右键单击网页,然后在 Chrome 浏览器中单击“检查”按钮。 要调查该网页上的元素,请单击可见 HTML 代码顶部的左上箭头,如下所示。
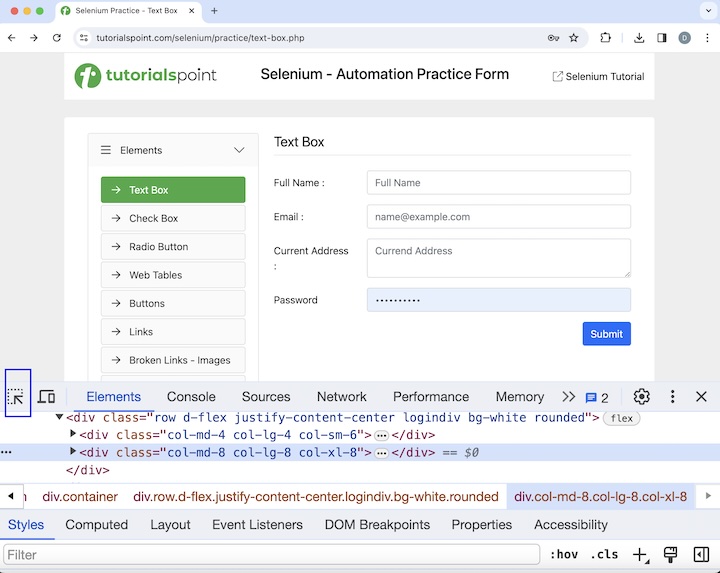
复制和粘贴文本
在下面的页面中,在第一个编辑框中的 Full Name 旁边输入文本 - Copy,然后将相同的文本复制并粘贴到 Email 旁边的另一个输入框中。
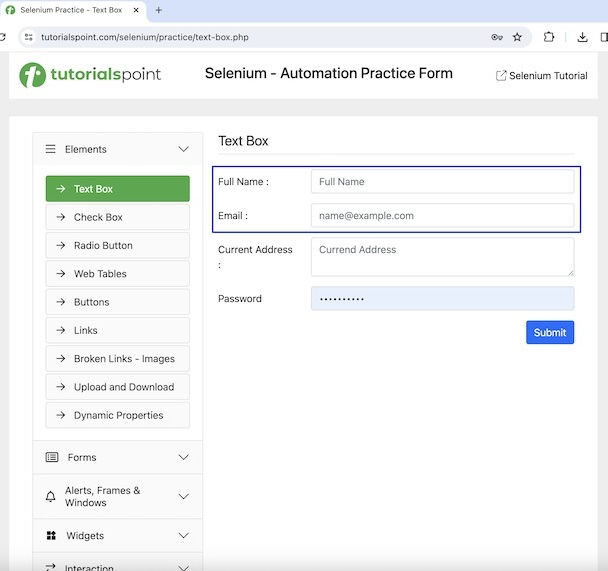
语法
在 MAC 机器上的语法 -
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Copy"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // Actions class methods to select text Actions a = new Actions(driver); a.keyDown(Keys.COMMAND); a.sendKeys("a"); a.keyUp(Keys.COMMAND); a.build().perform(); // Actions class methods to copy text a.keyDown(Keys.COMMAND); a.sendKeys("c"); a.keyUp(Keys.COMMAND); a.build().perform(); // Action class methods to tab and reach to the next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(Keys.COMMAND); a.sendKeys("v"); a.keyUp(Keys.COMMAND); a.build().perform();
在 Windows 机器上的语法 -
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Copy"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // Actions class methods to select text Actions a = new Actions(driver); a.keyDown(Keys.CONTROL); a.sendKeys("a"); a.keyUp(Keys.CONTROL); a.build().perform(); // Actions class methods to copy text a.keyDown(Keys.CONTROL); a.sendKeys("c"); a.keyUp(Keys.CONTROL); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(Keys.CONTROL); a.sendKeys("v"); a.keyUp(Keys.CONTROL); a.build().perform();
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class CopyAndPasteAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the first input box WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // enter text e.sendKeys("Copy"); // select text Actions act = new Actions(driver); act.keyDown(Keys.COMMAND); act.sendKeys("a"); act.keyUp(Keys.COMMAND); act.build().perform(); // copy text act.keyDown(Keys.COMMAND); act.sendKeys("c"); act.keyUp(Keys.COMMAND); act.build().perform(); // tab to reach to next input box act.sendKeys(Keys.TAB); act.build().perform(); // paste text act.keyDown(Keys.COMMAND); act.sendKeys("v"); act.keyUp(Keys.COMMAND); act.build().perform(); // Identify the second input box WebElement s = driver.findElement(By.xpath("//*[@id='email']")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Quit browser driver.quit(); } }
输出
Value copied and pasted: Copy Process finished with exit code 0
在上面的示例中,我们首先在第一个编辑框中输入了文本 Copy,然后将相同的文本复制并粘贴到第二个编辑框中。 最后,我们在控制台中检索了第二个输入框中输入的文本作为消息 - Value copied and pasted: Copy。
最后,收到消息 Process finished with exit code 0,表示代码成功执行。
文本大写
让我们再举一个例子,我们将使用 Actions 类的键盘向上、键盘向下功能在输入框中输入文本 TUTORIALSPOINT(大写字母)。 将值发送到 sendKeys() 方法时,我们将传递 tutorialspoint 以及按住 SHIFT 键,以使输入框中的输出为 TUTORIALSPOINT。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class KeyAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 17 secs driver.manage().timeouts().implicitlyWait(17, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the first input box WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // Actions class Actions act = new Actions(driver); // moving to element and clicking on it act.moveToElement(e).click(); // key UP and DOWN action for SHIFT act.keyDown(Keys.SHIFT); act.sendKeys("Selenium").keyUp(Keys.SHIFT).build().perform(); // get value entered System.out.println("Text entered: " + e.getAttribute("value")); // Close browser driver.quit(); } }
输出
Text entered: TUTORIALSPOINT
我们在控制台中使用消息获得了大写输入的文本 - Text entered: TUTORIALSPOINT。
结论
这总结了我们关于 Selenium WebDriver 键盘向上/向下键教程的全面概述。 我们从描述网页上元素的识别开始,并逐步介绍了如何使用 Selenium WebDriver 处理键盘向上和键盘向下方法的示例。 这使您深入了解了 Selenium WebDriver 键盘向上/向下键。 明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并扩展您的视野。