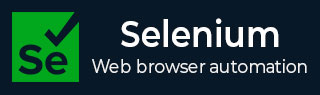
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试用例
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码生成
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 定位器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键按下/抬起
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 截图
- Selenium - 录制视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 与其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志
- Selenium - Log4j 日志
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 基于关键字的框架
- Selenium - 数据驱动的框架
- Selenium - 混合驱动的框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium Java 教程
Selenium 用于自动化测试 web 应用的测试用例。它支持多种编程语言,例如 Java、Python、C# 等。
如何使用 Java 设置 Selenium?
步骤 1 - 从以下链接下载并安装 Java:Java 下载。
有关如何设置 Java 的更详细视图,请参阅以下链接:Java 环境设置。
成功安装 Java 后,我们可以通过在命令提示符中运行命令:java 来确认其安装。
C:\java
步骤 2 - 接下来,我们将通过运行命令:java –version 来确认已安装的 Java 版本。
java –version
它将显示以下输出:
openjdk version "17.0.9" 2023-10-17 OpenJDK Runtime Environment Homebrew (build 17.0.9+0) OpenJDK 64-Bit Server VM Homebrew (build 17.0.9+0, mixed mode, sharing)
步骤 3 - 使用以下链接在我们的系统中安装 Maven:下载 Apache Maven。
接下来,我们将通过运行以下命令来确认已安装的Maven版本:
通过运行命令:mvn –version 来确认已安装的 Maven 版本。
mvn –version.
它将显示以下输出:
Apache Maven 3.9.6 (bc0240f3c744dd6b6ec2920b3cd08dcc295161ae) Maven home: /opt/homebrew/Cellar/maven/3.9.6/libexec Java version: 21.0.1, vendor: Homebrew, runtime: /opt/homebrew/Cellar/openjdk/21.0.1/libexec/openjdk.jdk/Contents/Home Default locale: en_IN, platform encoding: UTF-8 OS name: "mac os x", version: "14.0", arch: "aarch64", family: "mac"
执行的命令输出表明系统中安装的 Maven 版本为 Apache Maven 3.9.6。
步骤 4 - 安装名为IntelliJ的代码编辑器来编写和运行 Selenium 测试。
步骤 5 - 在 Main.java 文件中添加以下代码。
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class Main { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // URL launch driver.get("https://tutorialspoint.com/selenium/practice/resizable.php"); // get browser title after browser launch System.out.println("Browser title: " + driver.getTitle()); } }
在 pom.xml 文件中添加的总体依赖项:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.19.0</version> </dependency> </dependencies> </project>
步骤 6 - 右键单击并选择“运行‘Main.main()’”选项。等待运行完成。
步骤 7 - 应该启动 Chrome 浏览器,控制台中显示的消息应为 - 浏览器标题:Selenium 实践 - 可调整大小。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
此外,Chrome 浏览器启动时,顶部会显示消息Chrome 受自动化测试软件控制。
使用 Selenium Java 启动浏览器并退出驱动程序
我们可以使用 driver.get() 方法启动浏览器并打开应用程序,最后使用 close() 方法关闭浏览器。
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.edge.EdgeDriver; import java.util.concurrent.TimeUnit; public class CloseBrow{ public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // URL launch and get the browser title driver.get("https://tutorialspoint.com/selenium/practice/droppable.php"); System.out.println( "Browser title obtained : " + driver.getTitle()); // close browser driver.close(); } }
它将显示以下输出:
Browser title obtained: Browser Title: Selenium Practice - Droppable Process finished with exit code 0
在上面的示例中,我们首先启动了 Chrome 浏览器,然后获取了浏览器标题,然后关闭了浏览器,在控制台中收到了消息 - 获取的浏览器标题:Selenium 实践 - 学生注册表单。
Learn Java in-depth with real-world projects through our Java certification course. Enroll and become a certified expert to boost your career.
如何使用 Selenium Java 识别元素并检查其功能?
启动应用程序后,用户会与页面上的 web 元素交互,例如单击链接或按钮、在输入框中输入文本等,以创建自动化测试用例。
第一个任务是找到元素。Selenium 中有多个定位器,即 id、类、类名、名称、链接文本、部分链接文本、标签名、css 和 xpath。它们与 Java 中的 findElement() 方法一起使用。
例如,findElement(By.name(“name”)) 将识别具有名称属性值的第一个 web 元素。如果名称属性值相同的元素为零,则应抛出 NoSuchElementException
让我们看看下图中突出显示的同一输入框的 html 代码:
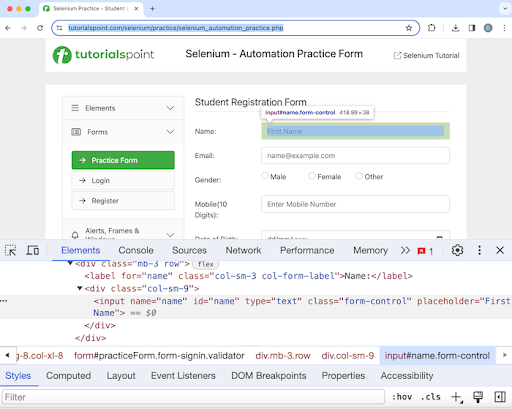
<input name="name" id="name" type="text" class="form-control" placeholder="First Name">
上图中突出显示的编辑框具有名称属性,其值为name。让我们在识别它之后将文本Selenium输入到此编辑框中。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorsName { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box enter text driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the search box with name locator to enter text WebElement i = driver.findElement(By.name("name")); i.sendKeys("Selenium"); // Get the value entered String text = i.getAttribute("value"); System.out.println("Entered text is: " + text); // Closing browser driver.quit(); } }
它将显示以下输出:
Entered text is: Selenium Process finished with exit code 0
输出显示消息 - 退出代码为 0 的进程,这意味着上述代码已成功执行。此外,在控制台中打印了从 getAttribute 方法获得的编辑框中输入的值 - Selenium。
结论
本教程总结了 Selenium Java 教程。我们首先介绍了如何使用 Java 设置 Selenium,如何使用 Selenium Java 启动浏览器并退出会话,以及如何使用 Selenium Java 识别元素并检查其功能。这使您掌握了 Selenium Java 教程的深入知识。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。