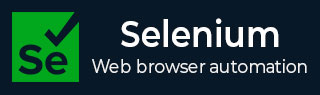
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - JSON 数据文件
Selenium WebDriver 可用于与 JSON 数据文件交互。在自动化测试中,经常需要通过 JSON 文件为测试用例提供大量数据,以验证特定场景或创建数据驱动框架。JSON 文件以键值对形式包含数据,其扩展名为 .json。
Java 提供了一些类和方法,可以使用 JSON Simple 库对 JSON 文件执行读写数据操作。JSON Simple 是一个轻量级的库,用于操作 JSON 对象。
如何安装 JSON Simple?
步骤 1 - 从以下链接添加 JSON Simple 依赖项:
https://mvnrepository.com/artifact/.
步骤 2 - 保存包含所有依赖项的 pom.xml 文件并更新 Maven 项目。
示例 1 - 从 JSON 文件读取所有值
让我们以名为 Detail.json 的 JSON 文件为例,我们将读取整个 JSON 文件并使用 JSONParser 类及其 parse() 方法检索其所有值。
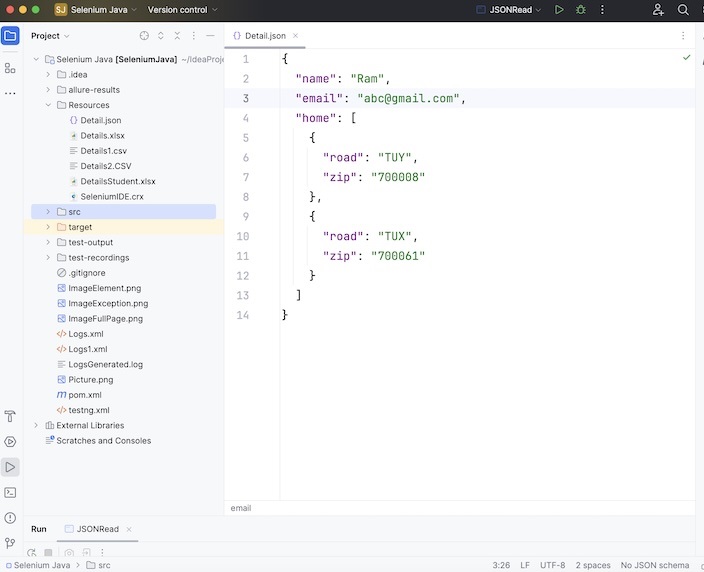
Detail.json 文件的内容如下:
{ "name": "Ram", "email": "[email protected]", "home": [ { "road": "TUY", "zip": "700008" }, { "road": "TUX", "zip": "700061" } ] }
从 Detail.json 文件检索值后,我们将把 name 和 email 键的值输入到以下页面中的 全名 和 电子邮件 字段中。
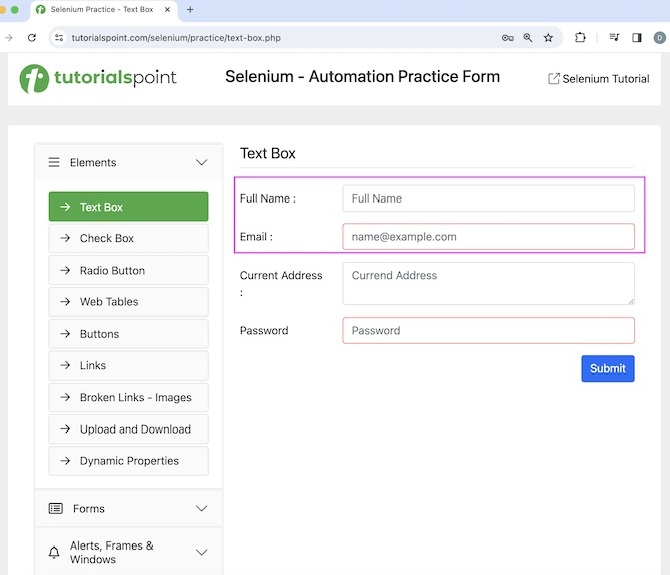
请注意:Details.json 文件放置在项目下的 Resources 文件夹中,如下图所示。
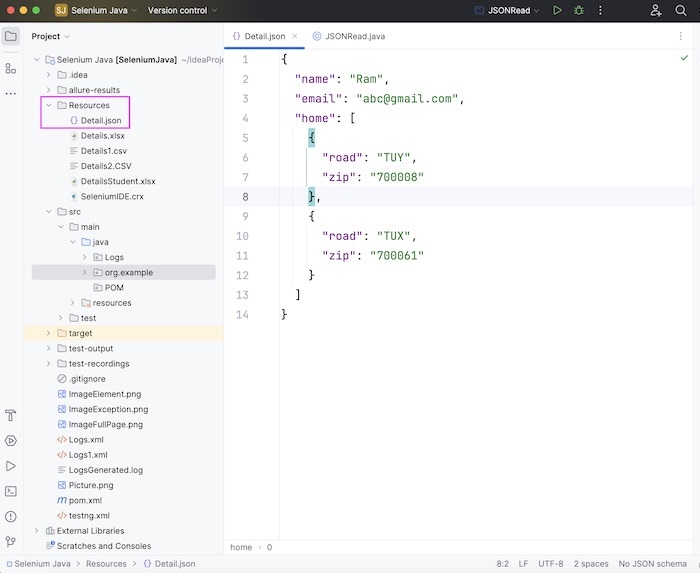
JSONRead.java 类文件的代码实现。
package org.example; import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.io.FileReader; import java.io.IOException; import java.util.concurrent.TimeUnit; public class JSONRead { public static void main(String[] args) throws IOException, ParseException { //object of JSONParser JSONParser j = new JSONParser(); // load json file to be read FileReader f = new FileReader("./Resources/Detail.json"); // parse json content Object o = j.parse(f); // convert parsing object to JSON object JSONObject detail = (JSONObject)o; // get values from JSON file String name = (String)detail.get("name"); String email = (String)detail.get("email"); System.out.println("First Name: " + name); System.out.println("Email: " + email); // get values from JSON array JSONArray h = (JSONArray)detail.get("home"); // iterate through the JSONArray for(int i = 0; i < h.size(); i ++){ JSONObject home = (JSONObject) h.get(i); String road = (String)home.get("road"); String zip = (String)home.get("zip"); System.out.println("Road: " + road); System.out.println("Zip: " + zip); } // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); //Identify elements WebElement n = driver.findElement(By.xpath("//*[@id='fullname']")); WebElement m = driver.findElement(By.xpath("//*[@id='email']")); // enter name and email after reading from JSON file n.sendKeys(name); m.sendKeys(email); // get values entered String enteredName = n.getAttribute("value"); String enteredEmail = m.getAttribute("value"); System.out.println("Entered Name: " + enteredName); System.out.println("Entered Email: " + enteredEmail); // quitting browser driver.quit(); } }
添加到 pom.xml 的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple --> <dependency> <groupId>com.googlecode.json-simple</groupId> <artifactId>json-simple</artifactId> <version>1.1.1</version> </dependency> </dependencies> </project>
输出
First Name: Ram Email: [email protected] Road: TUY Zip: 700008 Road: TUX Zip: 700061 Entered Name: Ram Entered Email: [email protected] Process finished with exit code 0
在上面的示例中,我们读取了整个 JSON 文件,并在控制台中获得了其所有值,消息为 - 姓名:Ram,电子邮件:[email protected],道路:TUY,邮编:700008,道路:TUX 和 邮编:700061。然后,将通过读取 JSON 文件获得的姓名和电子邮件的值输入到网页上的输入框中,并在控制台中也检索到输入的值,消息为 - 输入的姓名:Ram 和 输入的电子邮件:[email protected]。
最后,收到消息 Process finished with exit code 0,表示代码已成功执行。
示例 2 - 在 JSON 文件中写入和读取值
让我们再举一个例子,我们将创建一个名为 Detail1.json 的 JSON 文件,该文件位于项目中的 Resources 文件夹下,并在其中写入一些值。
package org.example; import org.json.simple.JSONObject; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; public class JSONReadWrite { public static void main(String[] args) throws IOException, ParseException { // object of JSONObject JSONObject j = new JSONObject(); // generate key-value pairs in JSON file j.put("Name", "Selenium"); j.put("Language", "Java"); // create JSON file with no duplicate values FileWriter f = new FileWriter("./Resources/Detail1.json", false); // write on json file f.write(j.toJSONString()); // close file after write f.close(); //object of JSONParser JSONParser j1 = new JSONParser(); // load json file to be read FileReader f1 = new FileReader("./Resources/Detail1.json"); // parse json content Object o = j1.parse(f1); // convert parsing object to JSON object JSONObject detail = (JSONObject)o; // get values from JSON file String name = (String)detail.get("Name"); String language = (String)detail.get("Language"); System.out.println("Name: " + name); System.out.println("Language: " + language); } }
添加到 pom.xml 的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple --> <dependency> <groupId>com.googlecode.json-simple</groupId> <artifactId>json-simple</artifactId> <version>1.1.1</version> </dependency> </dependencies> </project>
输出
Name: Selenium Language: Java Process finished with exit code 0
在上面的示例中,我们在项目中的 Resources 文件夹下创建了一个名为 Detail1.json 的 JSON 文件,并在其中写入了一些值。然后我们读取所有这些值,最后在控制台中以消息的形式获得它们 - 名称:Selenium 和 语言:Java。
最后,收到消息 Process finished with exit code 0,表示代码已成功执行。
此外,名为 Detail1.csv 的 JSON 文件已在项目目录中创建。单击它,我们将获得通过上述代码写入的值。
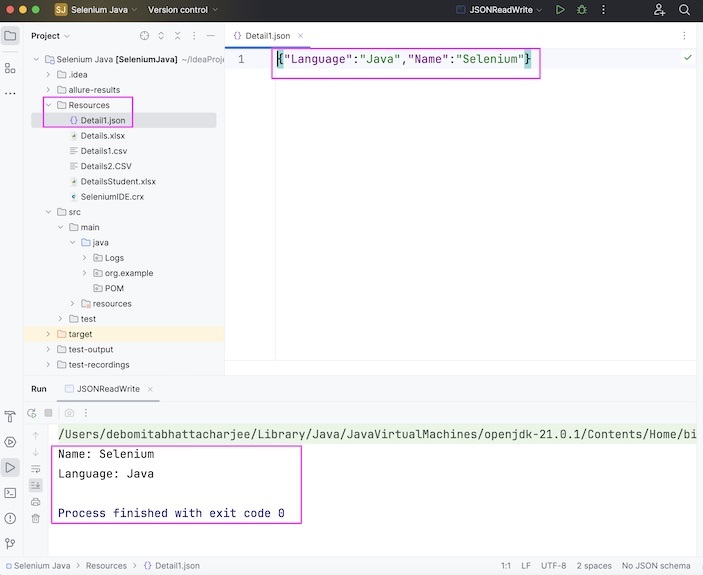
这总结了我们关于 Selenium WebDriver - JSON 数据文件的教程的全面内容。我们首先描述了什么是 JSON Simple 库,如何安装 JSON Simple,并逐步讲解了如何在使用 JSON Simple 和 Selenium WebDriver 的情况下读取和写入 JSON 文件中的值的示例。这使您能够深入了解 Selenium WebDriver 中的 JSON 数据文件。最好不断练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。