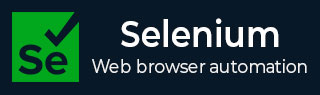
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态网页表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 杂项概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 用户交互
Selenium WebDriver 可用于各种用户交互,例如点击链接或按钮,在输入框中输入或清除文本,以及在下拉列表中选择或取消选择选项。
用户交互的基本 Selenium Webdriver 命令
Selenium Webdriver 中有多个命令可以帮助对元素执行操作。它们列在下面 -
click()
此方法用于在元素的中间执行点击事件。如果元素的中间隐藏,则会抛出异常。
右键点击网页,然后在 Chrome 浏览器中点击“检查”按钮。然后,将打开该页面的相关 HTML 代码。要检查下面页面中的某个链接,例如无内容,请点击 HTML 代码顶部突出显示的向上箭头。
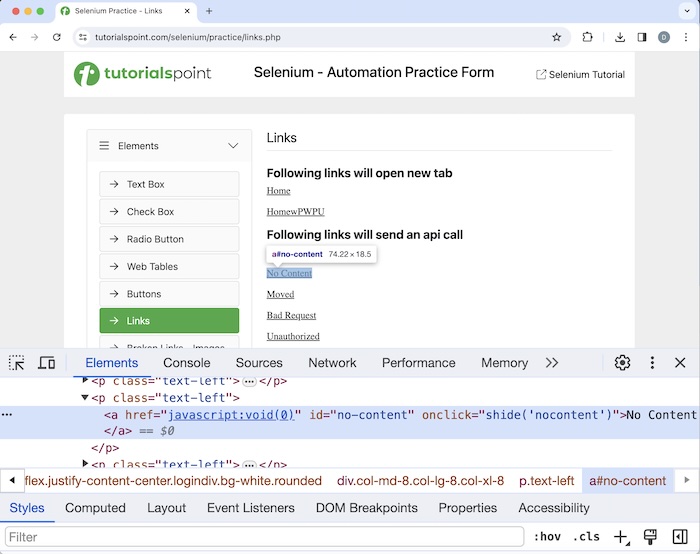
一旦我们点击并指向了“无内容”超链接的箭头,它的 HTML 代码就可见了。可以使用链接文本定位器检测链接。使用此方法,将定位具有链接文本匹配值的第一个链接。
示例
让我们举个例子,我们首先点击无内容链接,然后我们将获得文本链接已使用状态 204 和状态文本进行响应。
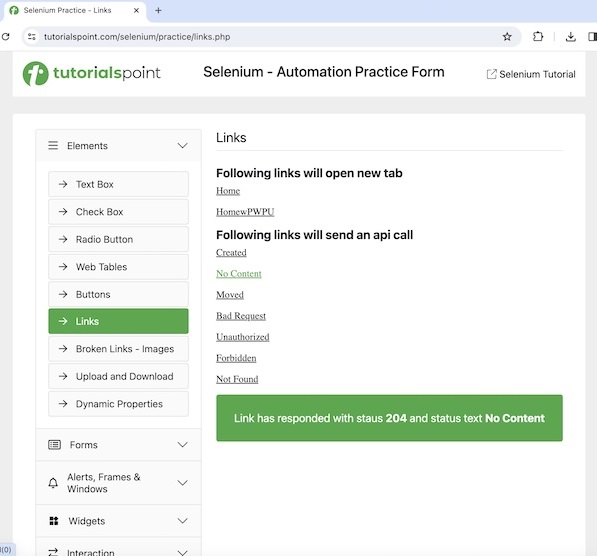
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.edge.EdgeDriver; import java.util.concurrent.TimeUnit; public class Lnks { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new EdgeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify link driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // Identify link with link text driver.findElement(By.linkText("No Content")).click(); // identify text WebElement l = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[2]")); // Get text after clicking the link System.out.println("Text is: " + l.getText()); // Closing browser driver.quit(); } }
输出
Text is: Link has responded with status 204 and status text No Content Process finished with exit code 0
在上面的示例中,点击无内容链接后获得的文本为文本为:链接已使用状态 204 和状态文本无内容进行响应。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
sendKeys()
此方法用于在编辑框或任何可编辑字段中输入一些文本。每个输入框都具有名为 input 的标签名。此外,输入框应具有 type 属性,其值为 text。如果元素不可编辑,并且在该元素上应用了 sendKeys() 方法,则应抛出异常。
clear()
此方法用于清除编辑框或任何可编辑字段中的文本。如果元素不可编辑,并且在该元素上应用了 clear() 方法,则应抛出异常。
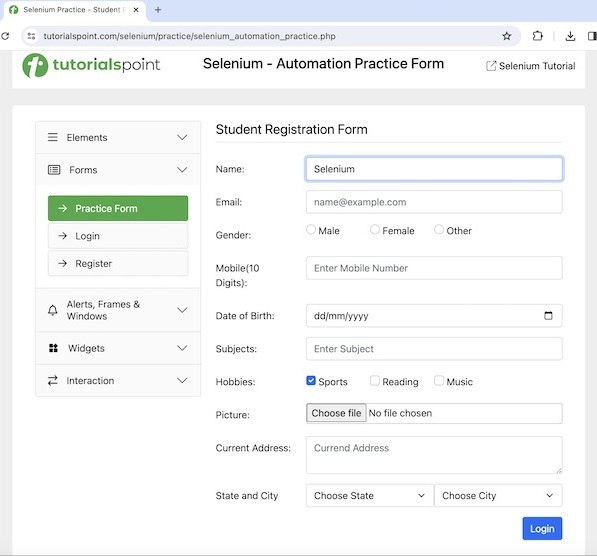
示例
让我们以上面页面的一个示例为例,我们首先使用sendKeys()方法在输入框中输入一些文本。要输入的值作为参数传递给该方法。然后,我们将使用clear()方法清除输入的文本。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class InptBox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box and enter driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // Get the value entered String text = e.getAttribute("value"); System.out.println("Entered text is: " + text); // clear the text entered e.clear(); // Get no text after clearing text String text1 = e.getAttribute("value"); System.out.println("Get text after clearing: " + text1); // Closing browser driver.quit(); } }
输出
Entered text is: Selenium Get text after clearing: Process finished with exit code 0
在上面的示例中,我们首先在输入框中输入了文本Selenium,并在控制台中检索了输入的值,消息为 - 输入的文本为:Selenium。然后清除输入的值,并且输入框中没有值。因此,我们在控制台中收到以下消息:清除后获取文本。
Select 类
Select 类中有多个方法可以帮助我们与下拉列表进行交互。每个下拉列表都由 select 标签名标识,其选项由 option 标签名标识。网页上可能有两种类型下拉列表 - 单选(允许选择一个选项)和多选(允许选择多个选项)。多选下拉列表还应具有一个属性 multiple。
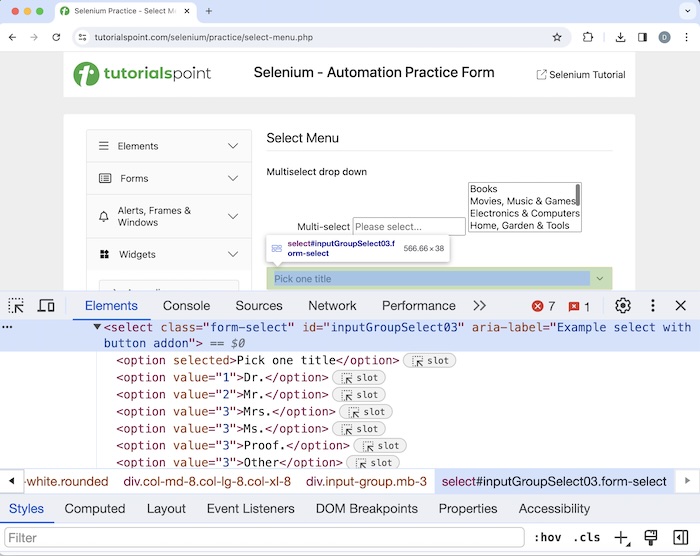
在上图中,选项选择一个标题具有属性 selected,这意味着默认情况下已选中此选项。Select 类中有多个方法允许处理Selenium Webdriver 中的下拉列表。
在下面的页面中,我们将访问“多选下拉列表”旁边的多选下拉列表,选择值电子产品和电脑和运动和户外,并执行一些验证。
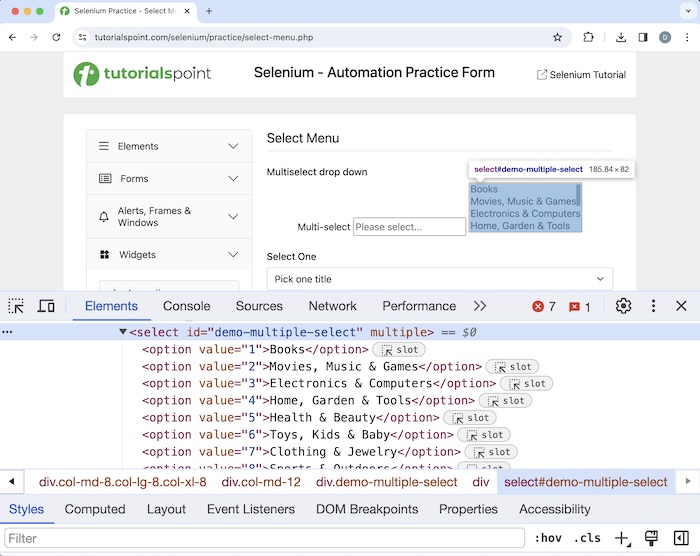
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class SelectMultipleDropdowns { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://tutorialspoint.com/selenium/practice/select-menu.php"); // identify multiple dropdown WebElement dropdown = driver.findElement (By.xpath("//*[@id='demo-multiple-select']")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by value select.selectByValue("3"); // select item by index select.selectByIndex(7); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(7); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> delectedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected: " + delectedOptions.size()); // Closing browser driver.quit(); } }
输出
Options are: Books Options are: Movies, Music & Games Options are: Electronics & Computers Options are: Home, Garden & Tools Options are: Health & Beauty Options are: Toys, Kids & Baby Options are: Clothing & Jewelry Options are: Sports & Outdoors Boolean value for multiple dropdown: true Selected Options are: Electronics & Computers Selected Options are: Sports & Outdoors First selected option is: Electronics & Computers No. options selected: 0 Process finished with exit code 0
在上面的示例中,我们使用控制台中的消息获取了下拉列表的所有选项 - 选项为:书籍,选项为:电影,音乐和游戏,选项为:家居,花园和工具,选项为:健康和美容,选项为:玩具,儿童和婴儿,选项为:服装和珠宝,选项为:运动和户外。我们还使用控制台中的消息验证了下拉列表是否具有多个选择选项 - 用于检查的布尔值为:true。
我们使用控制台中的消息检索了下拉列表中选定的选项 - 选定的选项为:电子产品和电脑,选定的选项为:运动和户外。我们还使用控制台中的消息获取了第一个选定的选项 - 第一个选定的选项为:电子产品和电脑。最后,我们取消选择了下拉列表中所有选定的选项,因此在控制台中获得了消息 - 选定的选项数:0。
滚动操作
Selenium webdriver 可以使用 JavaScriptExecutor(一个接口)在网页上执行滚动操作。Selenium Webdriver 可以使用 JavaScriptExecutor 执行 JavaScript 命令。
阅读更多:Selenium Webdriver 滚动操作.
结论
本教程全面介绍了 Selenium WebDriver 用户交互。我们从描述用户交互的基本 Selenium Webdriver 命令开始,并提供示例来说明如何在 Selenium Webdriver 中使用这些命令。这将使您深入了解 Selenium WebDriver 用户交互。建议您不断练习所学内容,并探索与 Selenium 相关的其他内容,以加深理解并拓宽视野。