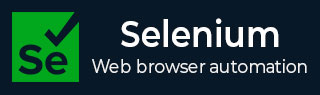
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver 与 RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 Iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 警报和弹出窗口
Selenium Webdriver 可用于处理警报和弹出窗口。网页上的警报旨在显示警告消息、信息或获取用户授权以继续执行进一步的操作。
在 Selenium 中处理警报和弹出窗口的基本方法
Selenium 中有多种方法可用于根据警报和弹出窗口自动化测试。要访问警报,我们必须首先使用 switchTo().alert() 方法将驱动程序上下文切换到警报。让我们详细讨论 Alert 接口的一些方法 -
- driver.switchTo().alert().accept() - 用于通过单击警报上显示的“确定”按钮来接受警报。
- driver.switchTo().alert().dismiss() - 用于通过单击警报上显示的“取消”按钮来取消警报。
- driver.switchTo().alert().getText() - 用于获取警报上显示的文本。
- driver.switchTo().alert().sendKeys("要输入的值") - 用于在警报上显示的输入文本上输入一些文本。
请注意,在使用 Alert 接口时,我们需要在测试中添加导入语句import org.openqa.selenium.Alert。
示例 1 - 警报
让我们讨论一下网页上的普通警报。单击页面上的“警报”按钮后,将生成一个警报,文本为 - Hello world!,如下图所示。
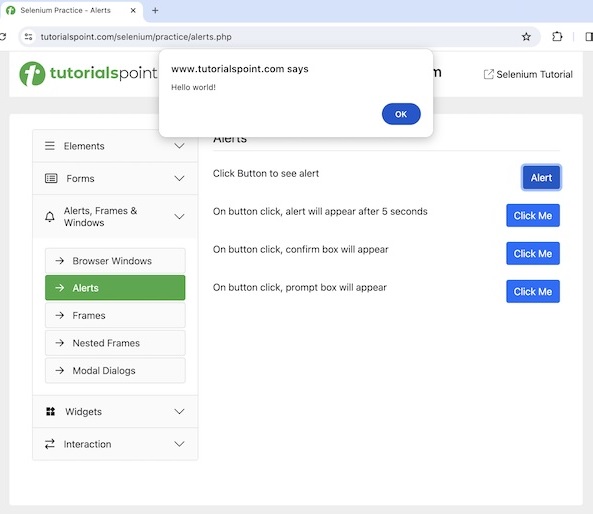
代码实现
package org.example; import org.openqa.selenium.Alert; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class Alrts { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will get alert driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // identify button for clicking to get alert WebElement c = driver.findElement(By.xpath("//button[text()='Alert']")); c.click(); // switch driver context to alert Alert alrt = driver.switchTo().alert(); // dismiss alert alrt.dismiss(); //again get the alert c.click(); // Get alert text String s = alrt.getText(); System.out.println("Alert text is: " + s); // accept alert alrt.accept(); // quitting the browser driver.quit(); } }
输出
Alert text is: Hello world! Process finished with exit code 0
在上面的示例中,我们捕获了警报上的文本,并在控制台中收到了消息 - 警报文本为:Hello world!。
最后,收到了消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 2 - 确认警报
让我们讨论一下网页上的另一个警报,如下图所示。单击第二个单击我按钮后,我们将在网页上获得一个警报,文本为 - 按一个按钮!。
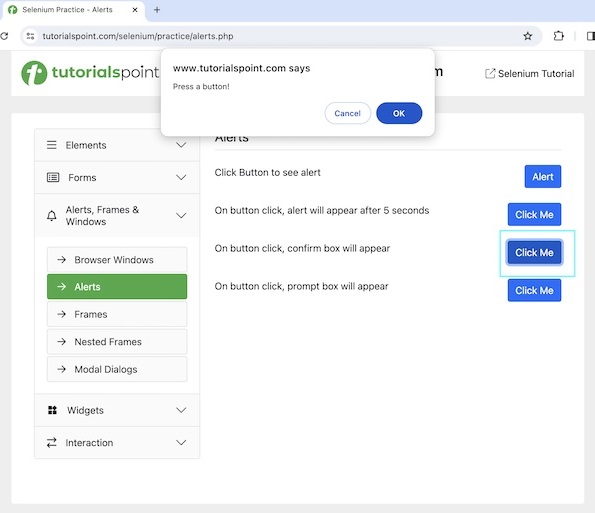
单击警报上的“确定”按钮后,我们将在网页上获得文本您按下了确定!。
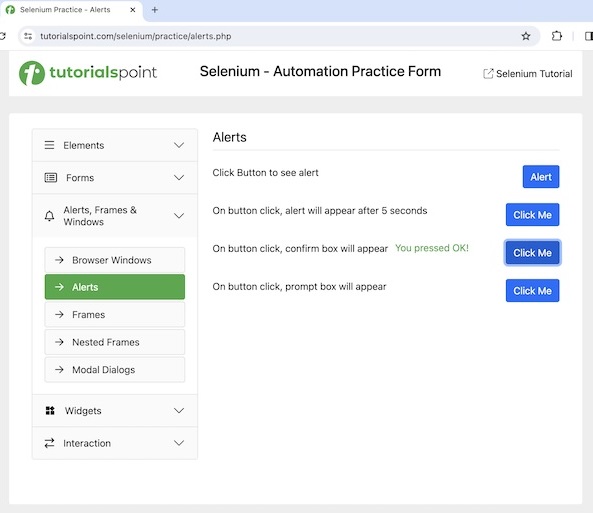
并且在单击警报上的“取消”按钮后,我们将在页面上获得文本您按下了取消!。
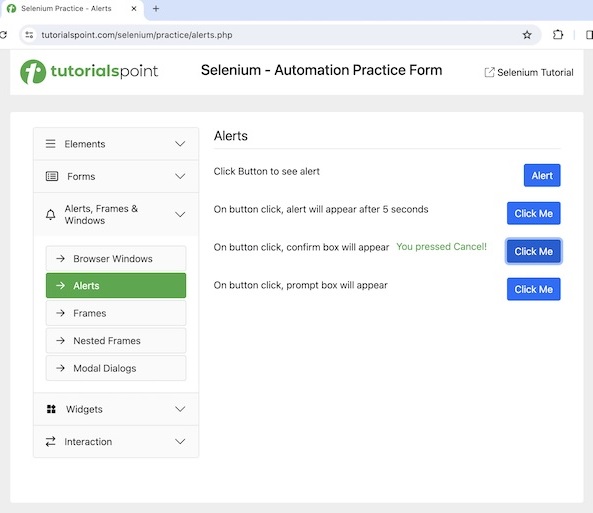
代码实现
package org.example; import org.openqa.selenium.Alert; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class AlertJS { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will get alert driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // identify button for clicking to get alert WebElement c =driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[3]/button")); c.click(); // switch driver context to alert Alert alrt = driver.switchTo().alert(); // Get alert text String s = alrt.getText(); System.out.println("Alert text is: " + s); //accept alert alrt.accept(); // get text after accepting alert WebElement text =driver.findElement(By.xpath("//*[@id='desk']")); System.out.println("Text appearing after alert accept: " + text.getText()); // again get the alert c.click(); // switch driver context to alert Alert alrt1 = driver.switchTo().alert(); // now dismiss alert alrt1.dismiss(); // get text after dismissing alert WebElement text1 =driver.findElement(By.xpath("//*[@id='desk']")); System.out.println("Text appearing after alert dismiss: " + text1.getText()); // quitting the browser driver.quit(); } }
输出
Alert text is: Press a button! Text appearing after alert accept: You pressed OK! Text appearing after alert dismiss: You pressed Cancel!
在上面的示例中,我们捕获了警报上的文本,并在控制台中收到了消息 - 警报文本为:按一个按钮!。然后在接受警报后,我们捕获了页面上显示的文本,并在控制台中显示了消息 - 警报接受后显示的文本:您按下了确定!。此外,在取消警报后,我们捕获了页面上显示的文本,并在控制台中显示了消息 - 警报取消后显示的文本:您按下了取消!。
示例 3 - 提示警报
让我们讨论一下网页上的另一个警报,如下图所示。单击第三个单击我按钮后,我们将在网页上获得一个警报,文本为 - 您的姓名是什么?以及一个输入框。
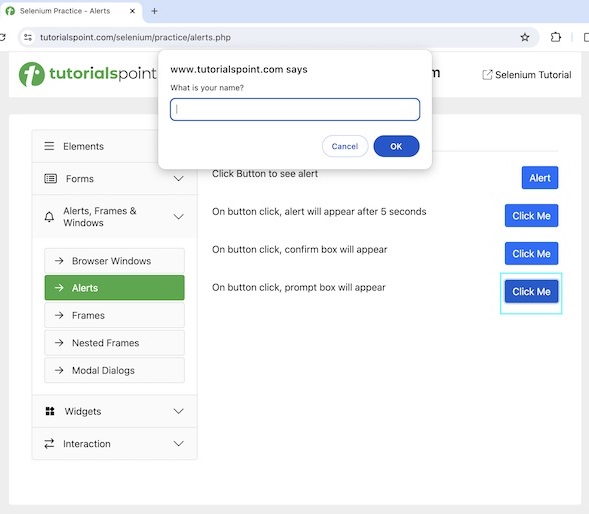
代码实现
package org.example; import org.openqa.selenium.Alert; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class AlertsPromp { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will get alert driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // identify button for clicking to get alert WebElement c =driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[4]/button")); c.click(); // switch driver context to alert Alert alrt = driver.switchTo().alert(); // Get alert text String s = alrt.getText(); System.out.println("Alert text is: " + s); // enter text then accept alert alrt.sendKeys("Selenium"); alrt.accept(); // again get the alert c.click(); // switch driver context to alert Alert alrt1 = driver.switchTo().alert(); // again enter text then dismiss alert alrt1.sendKeys("Selenium"); alrt1.dismiss(); // quitting the browser driver.quit(); } }
输出
Alert text is: What is your name?
在上面的示例中,我们捕获了警报上的文本,并在控制台中收到了消息 - 警报文本为:您的姓名是什么?
示例 4 - 带有等待条件的警报
让我们讨论一下网页上的另一个警报,如下图所示。单击第一个单击我按钮后,我们将获得一个警报,文本为 - Hello just appeared,将在 5 秒后出现。
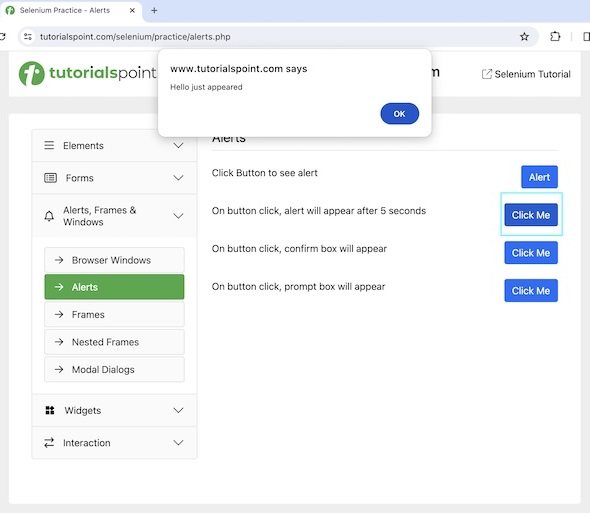
代码实现
package org.example; import org.openqa.selenium.Alert; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import java.time.Duration; public class AlertsPromp { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // Opening the webpage where we will get alert driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // identify button for clicking to get alert WebElement c = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[2]/button")); c.click(); // explicit wait to expected condition for presence alert WebDriverWait wt = new WebDriverWait(driver, Duration.ofSeconds(6)); wt.until(ExpectedConditions.alertIsPresent()); // switch driver context to alert Alert alrt = driver.switchTo().alert(); // Get alert text String s = alrt.getText(); System.out.println("Text is: " + s); // accept alert alrt.accept(); // quitting the browser driver.quit(); } }
输出
Text is: Hello just appeared
结论
这结束了我们关于 Selenium WebDriver 警报和弹出窗口教程的全面介绍。我们从描述在 Selenium 中处理警报和弹出窗口的基本方法开始,并通过示例说明如何在 Selenium Webdriver 中处理不同类型的警报和弹出窗口。这使您深入了解 Selenium WebDriver 警报和弹出窗口。明智的做法是不断练习您学到的知识并探索与 Selenium 相关的其他内容,以加深您的理解并扩展您的视野。