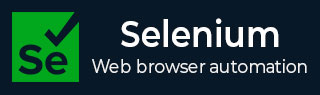
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium - 异常处理
可以使用 Selenium Webdriver 执行异常处理。在开发测试时,我们应该确保脚本即使出现错误也能继续执行。异常类似于在触发测试执行时遇到的错误。
如果由于元素未找到或预期结果与实际结果不匹配或任何其他原因而发生异常,我们的代码应生成异常并以逻辑方式结束测试,而不是突然终止脚本。异常分为两种类型:
已检查异常 - 这些异常可以在代码编译之前处理。
未检查异常 - 这些异常只能在运行时检测到,并且比已检查异常更难以处理。
识别和解决未检查异常的方法称为异常处理。Selenium 中的一些异常包括:
ElementNotVisibleException - 当元素存在于 DOM 中但不可见时,会引发此异常。因此,无法对其执行任何操作。
ElementNotInteractableException - 当元素存在于 DOM 中时,会引发此异常。但是,当对其执行操作时,另一个元素会受到影响。
ElementClickInterceptedException - 当无法完成元素单击命令时,会引发此异常。这是因为接收事件的元素隐藏了请求单击操作的元素。
ElementNotSelectableException - 当尝试选择不可选择的元素时,会引发此异常。
InsecureCertificateException - 当导航导致出现证书警告时,会引发此异常。这导致创建了已过期和不正确的 TLS 证书。
ErrorInResponseException - 此异常是由于服务器端错误而引发的。
ImeActivationFailedException - 此异常是由于 IME 引擎激活失败而引发的。
ImeNotAvailableException - 如果 IME 支持不可用,则会引发此异常。
InvalidElementStateException - 如果命令由于元素状态无效而未完成,则会引发此异常。
InvalidArgumentException - 如果命令参数无效,则会引发此异常。
InvalidCoordinatesException - 如果操作的坐标无效,则会引发此异常。
InvalidCookieDomainException - 为在不同域而不是当前 URL 下添加 Cookie 时引发此异常。
InvalidSwitchToTargetException - 当要切换到的目标窗口或框架不存在时,会引发此异常。
InvalidSelectorException - 如果用于标识元素的选择器无法获取 WebElement,则会引发此异常。
MoveTargetOutOfBoundsException - 当 ActionsChains move() 方法的目标无效时,会引发此异常。
InvalidSessionIdException - 如果提供的会话 ID 无效或不存在,并且不是活动会话的一部分,则会引发此异常。
NoSuchFrameException - 当要切换到的目标框架不存在时,会引发此异常。
NoAlertPresentException - 当要切换到的目标警报不存在时,会引发此异常。
NoSuchCookieException - 当当前活动浏览内容的 Cookie 中没有匹配的 Cookie 时,会引发此异常。
NoSuchAttributeException - 当元素属性丢失时,会引发此异常。
UnableToSetCookieException - 当驱动程序无法设置 Cookie 时,会引发此异常。
NoSuchWindowException - 当要切换到的目标窗口不存在时,会引发此异常。
TimeoutException - 当命令执行未在时间范围内完成时,会引发此异常。
StaleElementReferenceException - 当元素引用当前已过期时,会引发此异常。
UnexpectedTagNameException - 当辅助类找不到正确的 Web 元素时,会引发此异常。
UnexpectedAlertPresentException - 当出现意外警报时,会引发此异常。
NoSuchElementException - 此异常是 NotFoundException 类的子类。当无法在 DOM 中识别元素时,通常由 findElement() 方法引发。
SessionNotFoundException - 当驱动程序尝试在浏览器会话终止或关闭时对网页执行某些操作时,会引发此异常。
WebDriverException - 当驱动程序在驱动程序会话关闭后立即尝试工作时,会引发此异常。
让我们讨论一些处理异常的方法:
使用 try-catch 块
try 指示块的开始,catch 紧跟在 try 块之后以解决异常。
语法
try { //Perform Action } catch(Exception e1) { //Catch block 1 }
使用 try 和多个 catch 块
某些代码片段可能产生多个异常。每个catch块用于处理一种类型的异常。
语法
try { //Perform Action } catch(Exception e1) { //Catch block 1 } catch(Exception e2) { //Catch block 2 }
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
使用throw和throws
throw关键字用于生成自定义异常。如果抛出异常而没有处理它,则应该在方法签名中提到throws关键字。
语法
public static void errorHandling() throws Exception try { //Perform Action } catch(Exception e) { throw(e); }
使用try-catch-finally块
try指示块的开始,catch紧跟在try块之后用于解决异常。finally代码块无论脚本是否抛出异常都会执行。
语法
try { //Perform Action } catch(Exception e1) { //Catch block 1 } finally { //The finally block always executes. }
printStackTrace() − 此方法提供有关异常、堆栈跟踪以及执行的其他关键信息。
toString() − 此方法描述异常名称及其用途。
getMessage() − 此方法描述异常。
让我们来看一个例子,程序中遇到错误,由于代码中没有异常处理,执行在中途停止。
示例
ExceptionsEncountered.java类文件的代码实现。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ExceptionsEncountered { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // launch an application and open a URL driver.get("https://tutorialspoint.com/selenium/practice/auto-complete.php"); // identify element with incorrect xpath value WebElement l = driver.findElement(By.xpath("//*[@id='tag']")); // enter text l.sendKeys("Selenium"); // Quitting browser driver.quit(); } }
输出
Exception in thread "main" org.openqa.selenium.NoSuchElementException: no such element: Unable to locate element: {"method":"xpath","selector":"//*[@id='tag']"} (Session info: chrome=121.0.6167.160) For documentation on this error, please visit: https://selenium.net.cn/documentation/webdriver/troubleshooting/errors#no-such-element-exception Process finished with exit code 1
在上面的例子中,我们收到了NoSuchElementException异常。由于在上面的实现中没有应用异常处理,因此在遇到错误后执行立即停止。
最后,收到消息Process finished with exit code 1,表示代码执行不成功。
让我们看看如何使用try-catch和finally块在上述测试中添加异常处理。
示例
ExceptionHandlingEncountered.java类文件的代码实现。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.NoSuchElementException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ExceptionHandlingEncountered { public static void main(String[] args) { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // launch an application and open a URL driver.get("https://tutorialspoint.com/selenium/practice/auto-complete.php"); //try-catch finally block try { // identify element with incorrect xpath value WebElement l = driver.findElement(By.xpath("//*[@id='tag']")); // enter text l.sendKeys("Selenium"); } catch (NoSuchElementException e){ System.out.println("Catch block executed after try block"); } // finally block finally { System.out.println("finally block executed after try - catch block"); } // Quitting browser driver.quit(); } }
输出
Catch block executed after try block finally block executed after try - catch block Process finished with exit code 0
在本教程中,我们讨论了如何使用Selenium Webdriver执行异常处理。