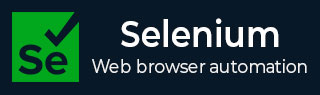
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 定位器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 基于关键字的框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium - 查找所有链接
Selenium Webdriver 可用于计算 Web 应用程序上的链接总数。通常,在执行网页抓取时,通常需要计算链接。所有链接(称为超链接)都具有名为anchor 的标签名,并且它具有一个称为 href 的属性。
HTML 中链接的识别
现在让我们讨论一下下图所示网页上“首页”链接的识别。打开 Chrome 浏览器,右键单击网页,然后单击“检查”按钮。然后,该页面的完整 HTML 代码将可用。要检查页面上的“首页”链接,请单击位于可见 HTML 代码顶部的左上方箭头,如下所示。
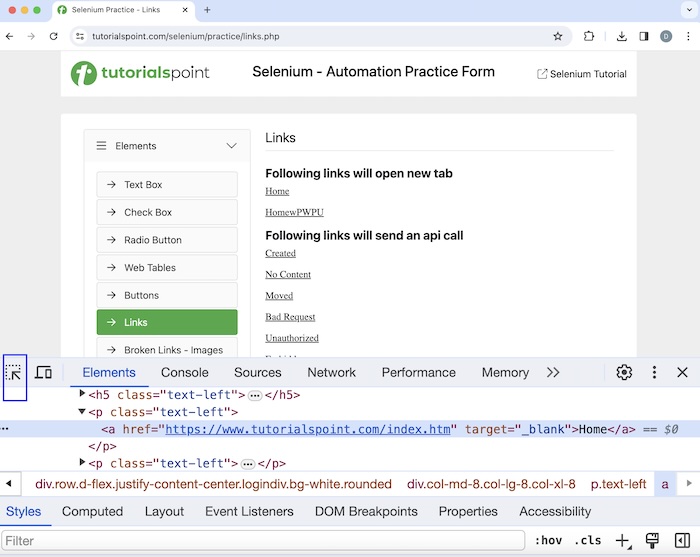
单击并将箭头指向首页超链接后,其 HTML 代码将可见,同时反映锚标签名(称为“a”)和包含页面链接的 href 属性。
<a href="https://tutorialspoint.com/index.htm" target="_blank">Home</a>
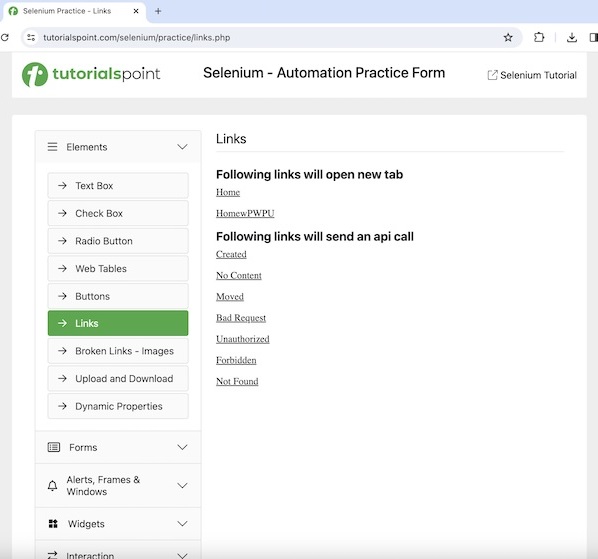
计算总链接数
让我们以以上页面为例,我们将在其中计算总链接数。在此页面上,总链接数应为 42。
示例
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.edge.EgeDriver; import java.util.List; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebElement; public class CountLinks { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new EdgeDriver() ; // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage where we will count the links driver.get( "https://tutorialspoint.com/selenium/practice/links.php" ) ; // Retrieve all links using locator By.tagName a and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a")); System.out.println( "Total number of links:" + totalLnks.size() ) ; // Closing browser driver.quit(); } }
添加到 pom.xml 的 Maven 依赖项
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
输出
Total number of links on a page: 42 Process finished with exit code 0
在上面的示例中,我们计算了网页上的总链接数,并在控制台中收到消息 - 页面上的总链接数:42。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
获取所有链接名称
现在,让我们来看一个获取上面讨论的网页上所有超链接名称的示例。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; public class TotalLinksWithNm { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver() ; // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage where we will count the links driver.get( "https://tutorialspoint.com/selenium/practice/links.php") ; // Retrieve all links using locator By.tagName and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a")); System.out.println( "Total number of links: " + totalLnks.size() ); // Running loop through list of web elements for ( int j = 0; j < totalLnks.size() ; j++) { // get the all hyperlink texts System.out.println( "Link text is: " + totalLnks.get(j).getText() ) ; } // Closing browser driver.quit(); } }
输出
Total number of links: 42 Link text is: Link text is: Selenium Tutorial Link text is: Text Box Link text is: Check Box Link text is: Radio Button Link text is: Web Tables Link text is: Buttons Link text is: Links Link text is: Broken Links - Images Link text is: Upload and Download Link text is: Dynamic Properties Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Link text is: Home Link text is: HomewPWPU Link text is: Created Link text is: No Content Link text is: Moved Link text is: Bad Request Link text is: Unauthorized Link text is: Forbidden Link text is: Not Found Process finished with exit code 0
在上面的示例中,我们计算了网页上的总链接数,并在控制台中收到消息 - 总链接数:42 和所有链接文本。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
结论
本教程全面介绍了 Selenium Webdriver 查找所有链接。我们从描述 HTML 中链接的识别开始,并通过示例说明如何在 Selenium Webdriver 中计算总链接数和链接名称。这使您能够深入了解 Selenium Webdriver - 查找所有链接。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。
广告