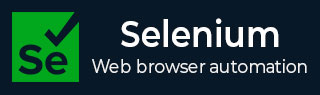
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - 断言/验证方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Python Selenium 教程
Selenium 用于为大多数基于 Web 的应用程序开发测试用例。它可以与多种编程语言一起使用,例如 Java、Python、Kotlin 等。
使用 Python 设置 Selenium 并启动浏览器
步骤 1 - 从链接 Windows 最新版本 下载并安装 Python。
要更详细地了解如何设置 Python,请参阅链接 Python 环境设置。
成功安装 Python 后,通过运行命令:python –version(在命令提示符下)确认其安装。执行的命令输出将指向机器中安装的 Python 版本。
步骤 2 - 从链接 PyCharm 安装名为 PyCharm 的 Python 代码编辑器。
使用此编辑器,我们可以开始处理 Python 项目以启动我们的测试自动化。要更详细地了解如何设置 PyCharm,请参阅以下链接 Pycharm 教程。
步骤 3 - 从命令提示符运行命令:pip install selenium。这是为了安装 Selenium。要确认机器中安装的 Selenium 版本,请运行命令 -
pip show selenium
此命令的输出给出了以下结果 -
Name: selenium Version: 4.19.0 Summary: None Home-page: https://selenium.net.cn Author: None Author-email: None License: Apache 2.0.
步骤 4 - 完成步骤 3 后,重新启动 PyCharm。
步骤 5 - 打开 PyCharm 并通过导航到“文件”菜单创建一个新项目。
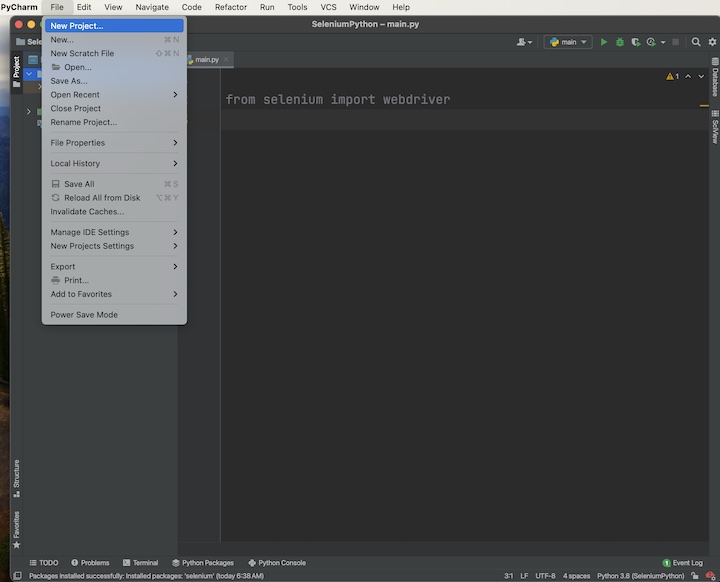
在“位置”字段中输入项目名称和位置,然后单击“创建”按钮。
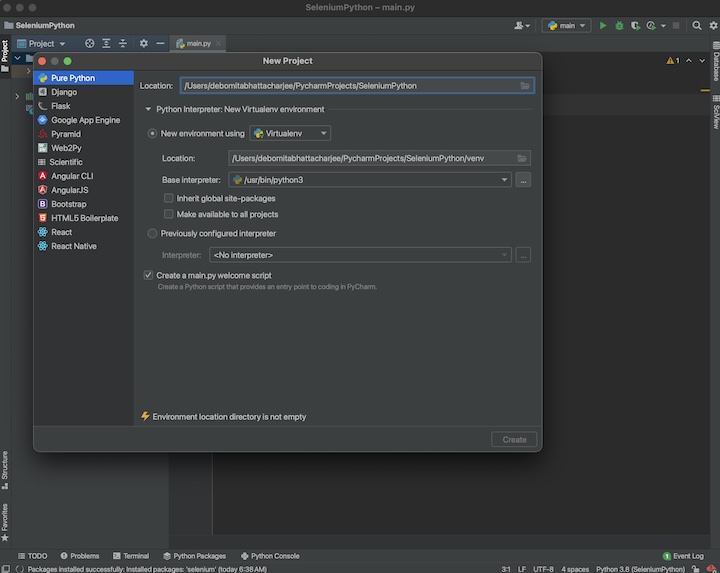
步骤 6 - 从 PyCharm 编辑器的右下角,选择“解释器设置”选项。
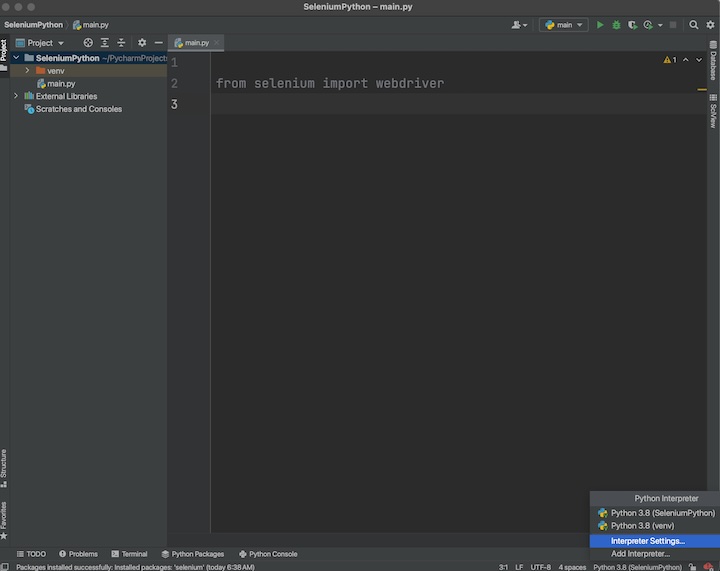
从左侧选择“Python 解释器”选项,然后单击“+”。
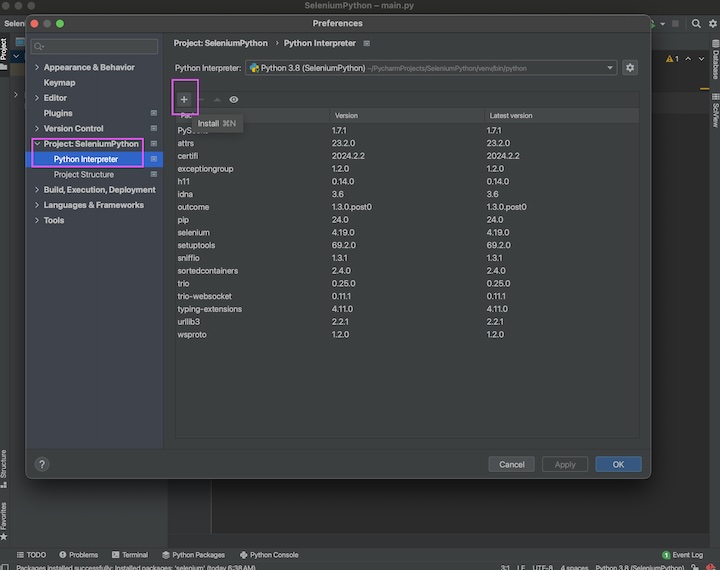
步骤 7 - 在“可用包”弹出窗口内的包搜索框中输入 selenium,然后搜索结果将与右侧的“描述”一起显示。描述包含有关将要安装的 Selenium 包版本的信息。
在“指定版本”字段旁边还有一个安装特定版本 Selenium 包的选项。然后单击“安装包”按钮。安装成功后,应显示消息“包'selenium'安装成功”。
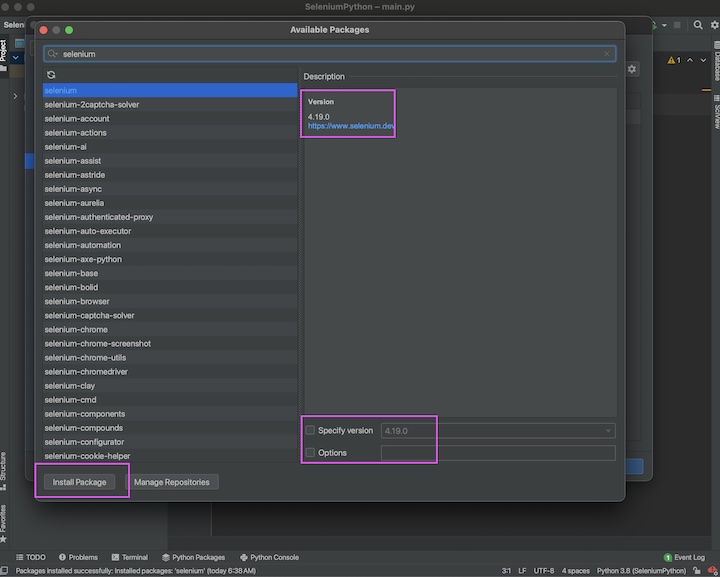
步骤 8 - 在“可用包”弹出窗口内的包搜索框中输入 webdriver-manager,并以相同的方式安装它。
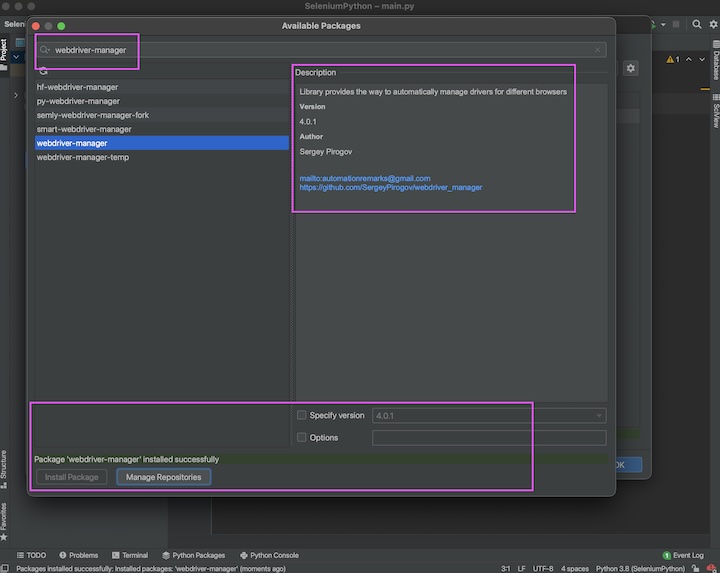
退出“可用包”弹出窗口。
步骤 9 - selenium 和 webdriver-manager 这两个包都应该反映在“包”下。单击“确定”按钮。重新启动 PyCharm。
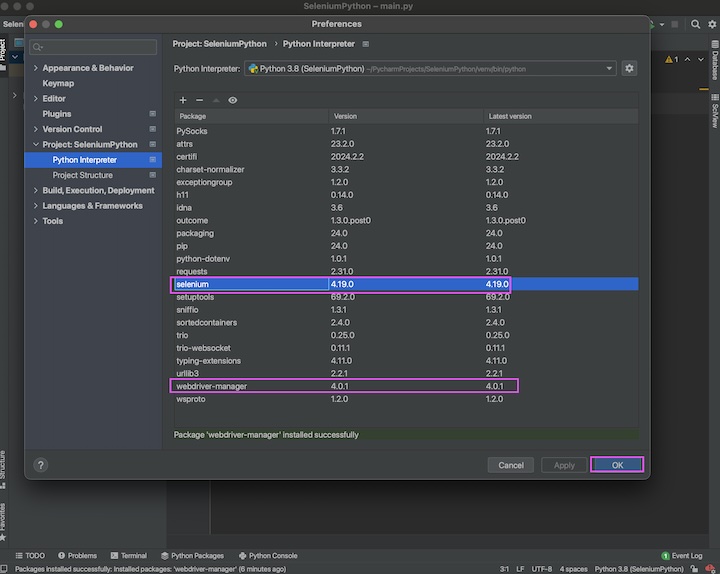
步骤 10 - 通过右键单击项目文件夹创建第一个测试用例。这里,我们将项目名称命名为 SeleniumPython。然后单击“新建”,最后单击“Python 文件”选项。
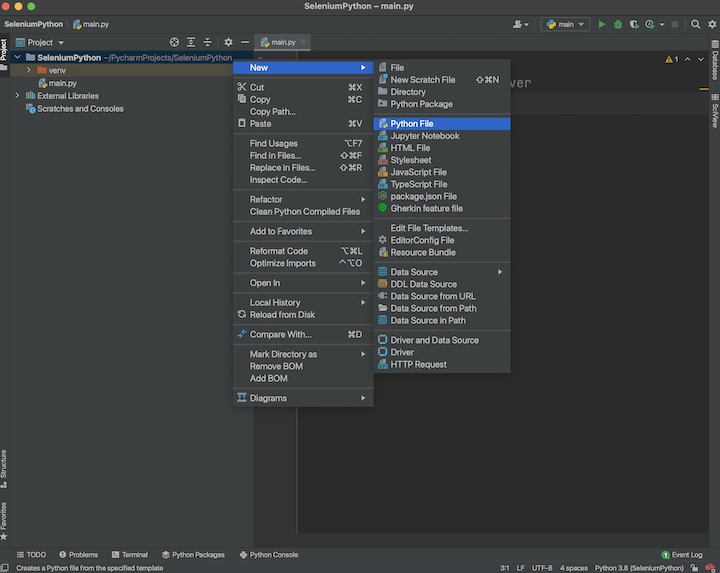
步骤 11 - 输入文件名,例如 Test1.py,并选择“Python 文件”选项,最后单击 Enter。
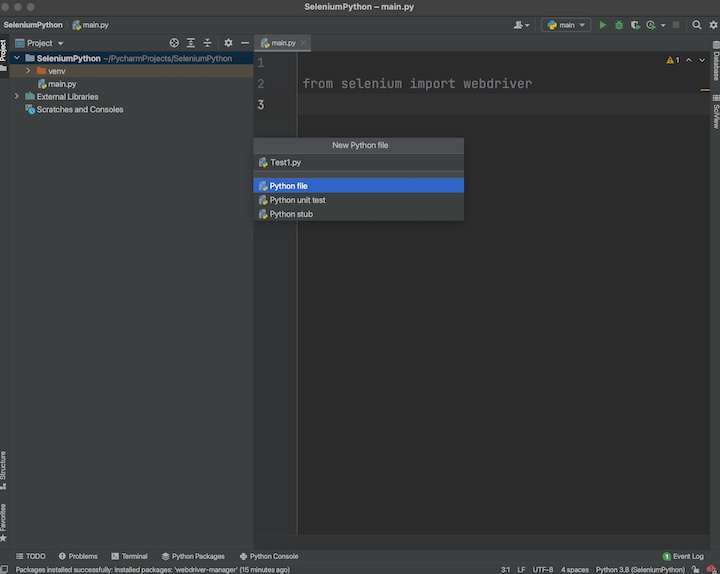
Test1.py 应该显示在左侧的 SeleniumPython 项目文件夹下。
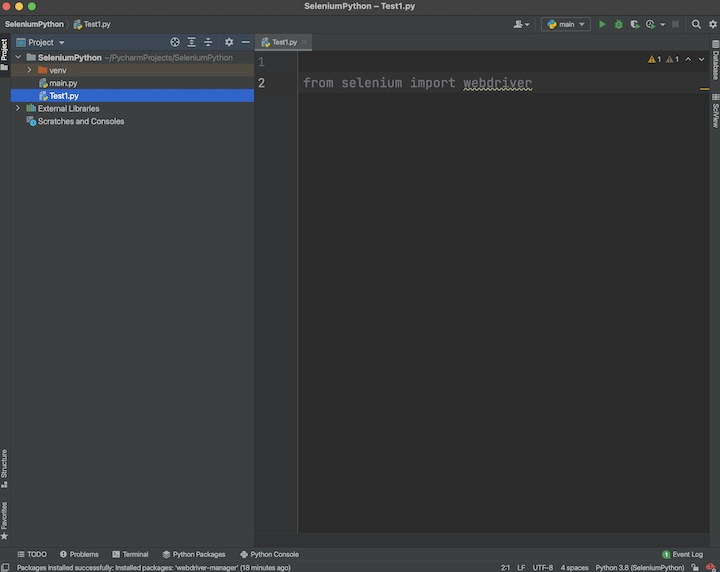
步骤 12 - 打开新创建的名称为 Test1.py 的 Python 文件,并获取以下页面的页面标题 - Selenium Practice - Alerts。
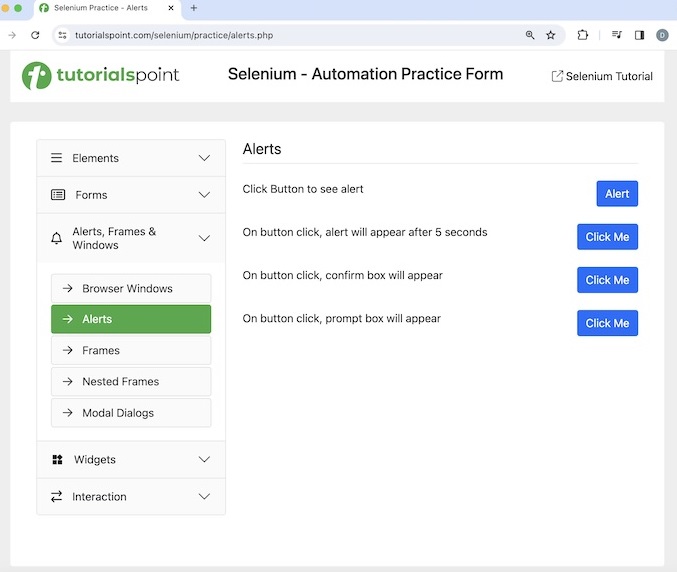
示例
from selenium import webdriver # create instance of webdriver driver = webdriver.Chrome() # launch application driver.get("https://tutorialspoint.com/selenium/practice/alerts.php") # get page title print("Page title is: " + driver.title) # quitting browser driver.quit
输出
Page title is: Selenium Practice - Alerts Process finished with exit code 0
在上面的示例中,我们启动了一个应用程序并在控制台中获取了其页面标题,消息为 - 页面标题为:Selenium Practice - Alerts。
输出显示消息 - 进程退出代码为 0,这意味着上述代码已成功执行。
使用 Selenium Python 识别元素并检查其功能
当应用程序启动后,用户会在网页上的 Web 元素上执行操作,例如单击链接或按钮、在输入框中输入文本、提交表单等,以自动化测试用例。
第一步是找到元素。Selenium 中有多种定位器可用,例如 id、类名、类、名称、标签名、部分链接文本、链接文本、标签名、xpath 和 css。这些定位器与 Python 中的 find_element() 方法一起使用。
例如,driver.find_element("classname", 'btn-primary') 定位第一个类名属性值为 btn-primary 的元素。如果不存在具有该类名属性匹配值的元素,则会抛出 NoSuchElementException。
让我们看看下面图像中突出显示的单击我按钮的 html 代码 -
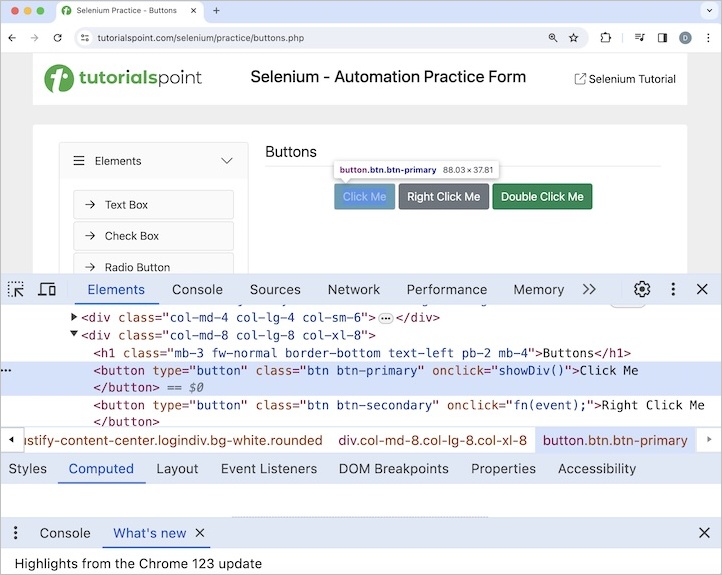
它的类名属性值为btn-primary。单击“单击我”按钮后,文本您已完成动态点击将出现在页面上。
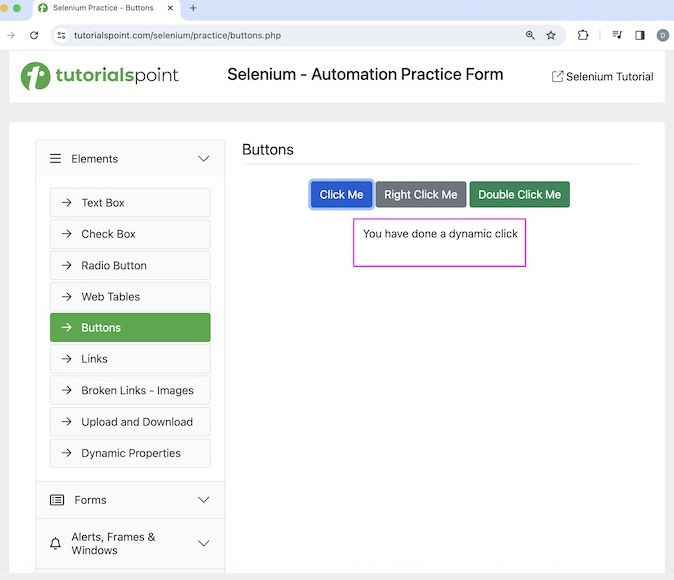
示例
from selenium import webdriver from selenium.webdriver.common.by import By # create instance of webdriver driver = webdriver.Chrome() # implicit wait of 15 seconds driver.implicitly_wait(15) # launch application driver.get("https://tutorialspoint.com/selenium/practice/buttons.php") # identify element with class name button = driver.find_element(By.CLASS_NAME, 'btn-primary') # click on button button.click() # identify element with id txt = driver.find_element(By.ID, 'welcomeDiv') # get text print("Text obtained is: " + txt.text) # quitting browser driver.quit
输出
Text obtained is: You have done a dynamic click
点击Click Me按钮后,我们在控制台获取了文本 - 获取到的文本为:You have done a dynamic click。
结论
本教程全面介绍了 Selenium Python 教程。我们从介绍如何使用 Python 设置 Selenium 并启动浏览器开始,然后讲解了如何识别元素并使用 Selenium Python 检查其功能。这使您能够深入了解 Selenium Python 教程。建议您不断练习所学内容,并探索其他与 Selenium 相关的知识,以加深理解并拓宽视野。