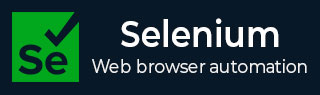
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 杂项概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium Grid - 测试执行
最新版本的 Selenium Grid 与旧版本的 Selenium Grid 有很多区别。旧版本的 Selenium Grid 只有 Hub 和 Node 两种模式,而最新版本的 Selenium Grid 支持三种模式:独立模式、Hub 和 Node 模式以及分布式模式。
以前的 Selenium Grid 版本仅包含 Hub 和 Node 两种模式,而最新版本的 Selenium Grid 有六个组件,如路由器、分发器、节点、会话队列、会话映射和事件总线。
Selenium Grid 是一种工具,可以将测试分布到多个物理或虚拟机上,以便我们可以并行(同时)执行脚本。它极大地加快了测试过程,并在多个浏览器和平台上运行测试,并为我们提供关于产品的快速准确的反馈。
Selenium Grid 允许我们执行多个 WebDriver 或 Selenium Remote Control 实例,允许并行执行使用相同代码库的测试,因此代码不需要存在于它们执行的系统上。selenium-server-standalone 包含 Hub、WebDriver 和 Selenium RC,用于在网格中执行脚本。
Selenium Grid 中测试执行的先决条件
步骤 1 - 在系统中安装 Java(版本高于 8),并使用命令检查它是否存在:java -version。如果安装已成功完成,则将显示已安装的 Java 版本。
步骤 2 - 通过打开浏览器并输入以下内容来检查网格状态
对于 UI 版本,键入https://127.0.0.1:4444。
对于非 UI 版本,键入https://127.0.0.1:4444/status。
在这两种情况下,我们都会收到错误 - 无法访问此网站。因为 Selenium Grid 尚未启动。

Selenium Grid 中的测试执行
在 Hub 和 Node 模式下在 Selenium Grid 中设置测试执行的步骤如下所示:
步骤 1 - 从以下链接下载 Selenium Standalone Jar 并将其保存到文件夹中:https://github.com/SeleniumHQ/selenium/releases。
步骤 2 - 从存储 Selenium Standalone Jar 的文件夹位置,从终端运行以下命令:
java -jar selenium-server-<version>.jar hub.
请注意 - 命令行日志中显示的 Hub IP 地址在注册 Hub 时收到。
在下图中,它显示了 Hub 的成功触发。

步骤 3 - 通过打开浏览器并输入以下内容来检查网格状态:https://127.0.0.1:4444。
错误 - 无法访问此网站将不再存在,并且我们将获得显示消息的网格状态 - 网格尚无注册节点。这是因为节点尚未注册。

在同一台机器上运行节点
按照上述步骤 1、2 和 3 配置 Hub 后,导航到存储 Selenium Standalone Jar 的文件夹位置,并通过打开另一个新终端窗口运行以下命令:
java -jar selenium-server-<version>.jar node.
这将有助于在同一台机器上启动节点。
在不同的机器上运行节点
步骤 1 - 在另一台机器上从以下链接下载 Selenium Standalone Jar 并将其保存到文件夹中:https://github.com/SeleniumHQ/selenium/releases。
步骤 2 - 导航到存储 Selenium Standalone Jar 的文件夹位置,并通过打开终端窗口运行以下命令:
java -jar selenium-server-<version>.jar node --detect-drivers true --publish-events tcp://{hub IP Address}:4442 --subscribe-events tcp://{hub IP Address}:4443.
请注意 - Hub IP 地址将在步骤 3 中获得的注册 Hub 时收到的命令行日志中提供。
这将有助于在不同的机器上启动节点。
在下图中,它显示了从同一台机器成功注册节点。

步骤 3 - 通过打开浏览器并输入以下内容再次检查网格状态:https://127.0.0.1:4444。
显示的消息 - 网格尚无注册节点将不存在,取而代之的是,节点将反映出来。

步骤 4 -
Base.java中的代码实现
package BaseClass; import java.net.MalformedURLException; import java.net.URL; import org.openqa.selenium.WebDriver; import org.openqa.selenium.remote.DesiredCapabilities; import org.openqa.selenium.remote.RemoteWebDriver; public class Base { public WebDriver setBrowser(String browserName) throws MalformedURLException { WebDriver driver = null; DesiredCapabilities dc = new DesiredCapabilities(); if(browserName.equalsIgnoreCase("chrome")) { dc.setBrowserName("chrome"); } else if(browserName.equalsIgnoreCase("edge")) { dc.setBrowserName("MicrosoftEdge"); } // Initiate RemoteWebDriver driver = new RemoteWebDriver(new URL("https://127.0.0.1:4444"),dc); return driver; } }
TestOne.java中的代码实现
package Grid; import BaseClass.Base; import org.openqa.selenium.WebDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import java.net.MalformedURLException; public class TestOne extends Base { public WebDriver driver = null; @Test public void testOne() { // launch application driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // get page title System.out.println("Page title is: " + driver.getTitle() + " obtained from testOne"); } @BeforeMethod public void setup() throws MalformedURLException { driver = setBrowser("chrome"); } @AfterMethod public void tearDown() { // quitting browser driver.quit(); } }
TestTwo.java中的代码实现
package Grid; import BaseClass.Base; import org.openqa.selenium.WebDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import java.net.MalformedURLException; public class TestTwo extends Base { public WebDriver driver = null; @Test public void testTwo() { // launch application driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // get page title System.out.println("Page title is: " + driver.getTitle() + " obtained from testTwo"); } @BeforeMethod public void setup() throws MalformedURLException { driver = setBrowser("edge"); } @AfterMethod public void tearDown() { // quitting browser driver.quit(); } }
testng.xml 文件中的配置。
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd"> <suite name = "Grid Test"> <test thread-count = "5" name="Test"> <classes> <class name="Grid.TestOne" /> <class name="Grid.TestTwo"/> </classes> </test> </suite>
步骤 5 - 从 testng.xml 文件运行测试。
它将显示以下输出:
Page title is: Selenium Practice - Links obtained from testOne Page title is: Selenium Practice - Links obtained from testTwo =============================================== Grid Test Total tests run: 2, Passes: 2, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
在上面的示例中,我们使用 Selenium Grid 的 Hub 和 Node 模式执行了测试执行。
这总结了我们关于 Selenium Grid - 测试执行教程的全面内容。我们从描述在 Selenium Grid 中执行测试执行的先决条件开始,以及如何在 Selenium Grid 中实际执行测试执行。
这使您能够深入了解 Selenium Grid - 测试执行。明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并扩展您的视野。