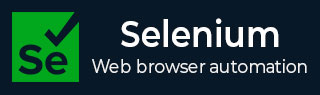
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试用例
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键点击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态网页表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放操作
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 拖放操作
当我们尝试将网页的一个部分的元素移动到另一个部分时,就会在应用程序上执行拖放操作。Selenium web driver 可用于自动化处理页面上拖动元素的测试。
Selenium webdriver 可以借助 Actions 类中的 dragAndDrop() 和 dragAndDropBy() 方法来执行拖放操作。dragAndDrop 方法接受两个参数 - 源和目标定位器值。dragAndDropBy 方法接受三个参数 - 源定位器、水平方向上的 x 偏移值(以像素为单位)和垂直方向上的 y 偏移值(以像素为单位)。
元素识别
打开 Chrome 浏览器并启动应用程序。右键单击网页,然后单击“检查”按钮。然后,整个页面的实际 HTML 代码将可见。要检查该网页上的源元素和目标元素,请单击位于可见 HTML 代码顶部的左上箭头,如下所示。
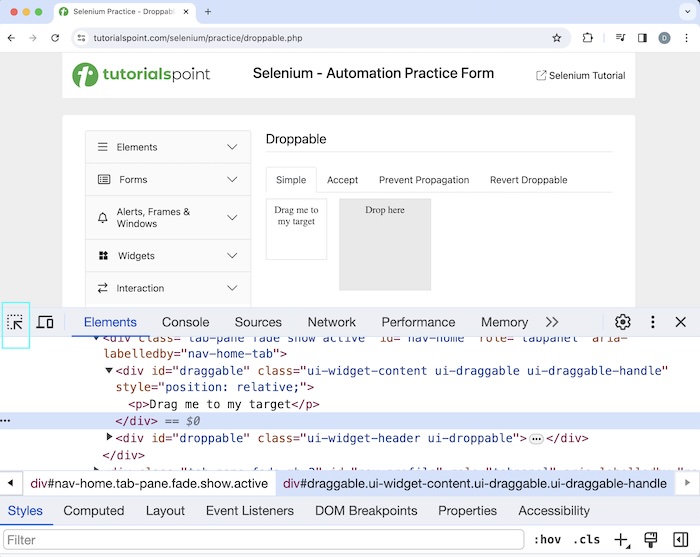
示例 1
让我们以以上页面为例,将文本为 Drag me to my target 的源元素拖动到文本为 Drop here 的目标元素。完成后,我们将在网页上获得文本 Dropped!。
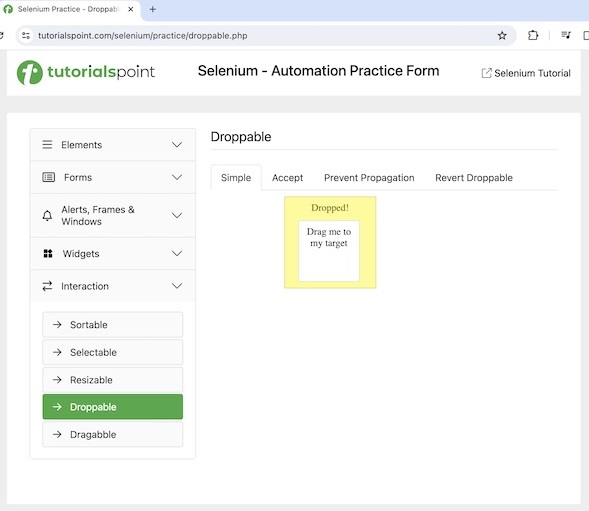
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class DragAndDrp { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch driver.get("https://tutorialspoint.com/selenium/practice/droppable.php"); // identify source and target elements WebElement sourceElement= driver.findElement(By.id("draggable")); WebElement targetElement= driver.findElement(By.id("droppable")); // drag and drop operations without build and perform methods Actions a = new Actions(driver); a.dragAndDrop(sourceElement, targetElement).build().perform(); // identify text after element is dropped WebElement text = driver.findElement(By.xpath("//*[@id='droppable']/p")); System.out.println("Text is : " + text.getText()); // quitting browser after drag and drop operations completed driver.quit(); } }
输出
Text is : Dropped! Process finished with exit code 0
在以上示例中,我们从源到目标定位器执行了拖放操作,并在控制台中获得了消息 Text is : Dropped!。
最后,收到了消息 Process finished with exit code 0,表示代码已成功执行。
示例 2
我们还可以借助 Actions 类 中的 clickAndHold()、moveToElement() 和 release() 方法来实现以上示例。最后,我们将使用 perform() 和 build() 方法分别执行和完成所有操作。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class DragAndDrpOption2 { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // URL launch for accessing drag and drop elements driver.get("https://tutorialspoint.com/selenium/practice/droppable.php"); // identify source and target elements for drag and drop WebElement sourceElement= driver.findElement(By.id("draggable")); WebElement targetElement= driver.findElement(By.id("droppable")); // performing drag and drop operations Actions a = new Actions(driver); a.clickAndHold(sourceElement) .moveToElement(targetElement) .release(targetElement) .build().perform(); // identify text after element is dropped WebElement text = driver.findElement(By.xpath("//*[@id='droppable']/p")); System.out.println("Text is : " + text.getText()); // quitting browser after drag and drop driver.quit(); } }
输出
Text is after dragging: Dropped!
这里,我们从源到目标定位器执行了拖放操作,并在控制台中收到了消息 Text is : Dropped!。
示例 3
我们还可以借助 Actions 类的 dragAndDropBy() 方法来实现以上示例。
WebDriver driver = new ChromeDriver(); WebElement sourceElement= driver.findElement(By.id("<value of id>")); WebElement targetElement= driver.findElement(By.id("<value of id>")); // Drag and drop by method of Actions class Actions a = new Actions(driver); a.dragAndDropBy(sourceElement, x offset value, y offset value).build().perform();
x 偏移值可以通过目标元素和源元素的 x 坐标之差获得。类似地,y 偏移值可以通过目标元素和源元素的 y 坐标之差获得。
使用 dragAndDropBy 方法执行拖放操作的语法:
WebDriver driver = new ChromeDriver(); WebElement sourceElement= driver.findElement(By.id("<value of id>")); WebElement targetElement= driver.findElement(By.id("<value of id>")); // get x coordinates of source element int x = sourceElement.getLocation().getX(); // get y coordinates of target element int y = targetElement.getLocation().getY(); // get x coordinates of target element int x1 = targetElement.getLocation().getX(); // get y coordinates of source element int y1 = sourceElement.getLocation().getY(); // off set difference between target and source int locX = x1 - x; int locY = y1 - y; // drag and drop operations with offset Actions a = new Actions(driver); a.dragAndDropBy(sourceElement, locX, locY).build().perform();
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class DragAndDrpBy { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // URL launch for accessing drag and drop elements driver.get("https://tutorialspoint.com/selenium/practice/droppable.php"); // identify source and target elements for drag and drop WebElement sourceElement= driver.findElement(By.id("draggable")); WebElement targetElement= driver.findElement(By.id("droppable")); // get x coordinates of source element int x = sourceElement.getLocation().getX(); // get y coordinates of target element int y = targetElement.getLocation().getY(); // get x coordinates of target element int x1 = targetElement.getLocation().getX(); // get y coordinates of source element int y1 = sourceElement.getLocation().getY(); // off set difference between target and source int locX = x1 - x; int locY = y1 - y; // drag and drop with offset Actions act = new Actions(driver); act.dragAndDropBy(sourceElement, locX, locY).build().perform(); // identify text after element is dropped WebElement text = driver.findElement(By.xpath("//*[@id='droppable']/p")); System.out.println("Text is : " + text.getText()); // quitting browser after drag and drop operations completed driver.quit(); } }
输出
Text is : Dropped!
在以上示例中,我们首先从源到目标定位器以及 x、y 偏移值执行了拖放操作,并在控制台中收到了消息 Text is : Dropped!。
结论
这结束了我们关于 Selenium Webdriver 拖放操作教程的全面介绍。我们从描述网页上拖放操作的源元素和目标元素的识别开始,并逐步讲解了如何使用 Selenium Webdriver 处理拖放操作的示例。这使您深入了解 Selenium Webdriver 拖放操作。明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并扩展您的视野。