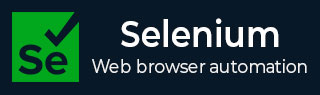
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 定位器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 定位器
Selenium Webdriver 可以用来定位具有相同匹配定位器值的元素。在网页上,如果多个元素具有相同的定位器值,则仅获取第一个匹配元素(从页面右上角开始)的引用。
findElement 方法
findElement() 方法返回 DOM 中第一个具有相同定位器值的元素。如果有多个元素具有相同的定位器值,我们可以使用 id 定位器识别单个元素,因为它始终唯一地标识网页上的一个元素。
使用嵌套 findElement 识别元素
让我们以下面图片中突出显示的 HTML 代码片段为例,其中包含与 li 标记名称相同的元素。让我们识别第一个 li 元素(其父元素的标记名称为 ul)具有子元素链接(标记名称为“a”)为 文本框 -
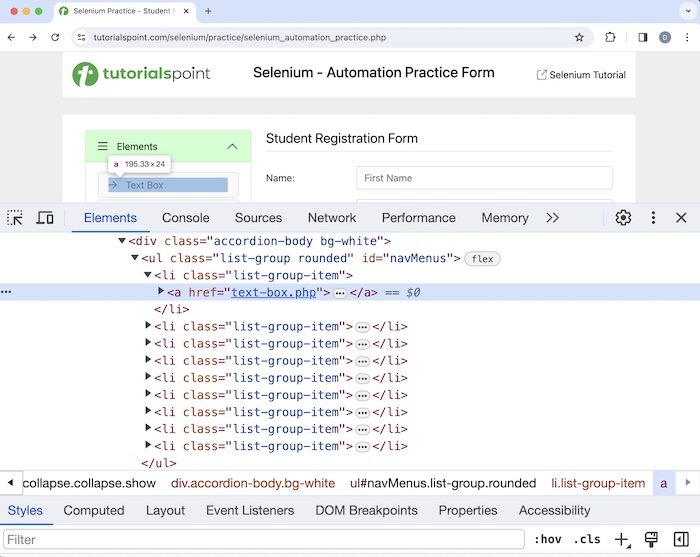
为了获取该元素,我们首先唯一地识别了作为搜索元素的祖先而不是不需要的元素的祖先的元素,然后在该对象上应用了 findElement() 方法。
我们可以使用 findElements() 方法获取所有具有相同定位器值的匹配元素。这将返回一个匹配元素列表。如果不存在匹配元素,我们将获得一个大小为 0 的列表。
带嵌套命令的语法 -
WebDriver driver = new ChromeDriver(); // identify all elements under tagname ul WebElement elements = driver.findElement(By.id("navMenus")); // identify first li element under ul then click WebElement element = elements.findElement(By.xpath("//li[1]/a"));
示例 1
让我们单击 文本框 链接,并在网页上获取文本 文本框。
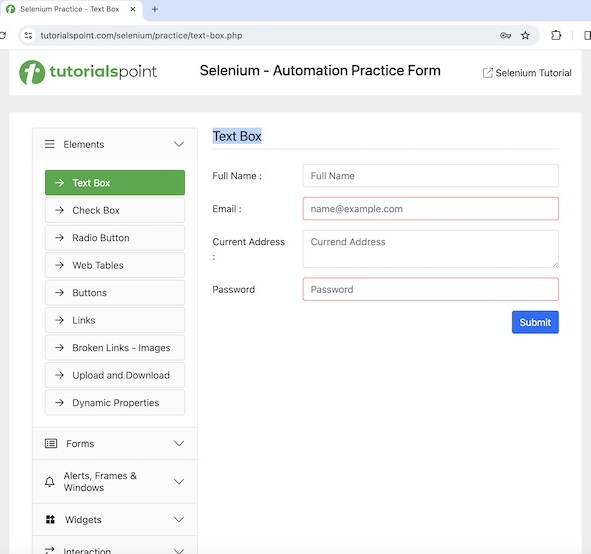
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class Finders { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify element then click WebElement l = driver.findElement(By.xpath("//*[@id='headingOne']/button")); l.click(); // identify all elements under tagname ul WebElement elements = driver.findElement(By.id("navMenus")); // identify first li element under ul then click WebElement element = elements.findElement(By.xpath("//li[1]/a")); element.click(); WebElement e = driver.findElement(By.xpath("//*[@id='TextForm']/h1")); System.out.println("Text is: " + e.getText()); // Closing browser driver.quit(); } }
输出
Text is: Text Box
在上面的示例中,我们首先使用嵌套定位器识别了元素,然后在控制台中获取带有消息的文本:文本是:文本框。
最后,收到消息 进程已完成,退出代码为 0,表示代码已成功执行。
但是,我们可以使用优化的技术来使用 xpath 或 css 定位器识别该元素,因为从性能方面考虑,嵌套方法并不好。
语法
WebDriver driver = new ChromeDriver(); // identify element with xpath WebElement element = driver.findElement(By.xpath("//*[@id='navMenus']/li[1]/a"));
示例 2
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class Finder { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify element then click WebElement l = driver.findElement(By.xpath("//*[@id='headingOne']/button")); l.click(); // identify element with xpath WebElement element = driver.findElement(By.xpath("//*[@id='navMenus']/li[1]/a")); element.click(); WebElement e = driver.findElement(By.xpath("//*[@id='TextForm']/h1")); System.out.println("Text is: " + e.getText()); // Closing browser driver.quit(); } }
输出
Text is: Text Box
在上面的示例中,我们首先使用 xpath 定位器识别了元素,然后在控制台中获取带有消息的文本:文本是:文本框。
使用 findElements 方法识别链接
链接由锚标记名称与称为 href 的属性识别。
现在让我们讨论一下下面图片中显示的网页上链接 错误请求 的识别。首先,右键单击页面,然后在 Chrome 浏览器中单击“检查”按钮。然后,将显示整个页面的相应 HTML 代码。要检查页面上的链接,请单击位于 HTML 代码顶部的左侧向上箭头,如下所示。
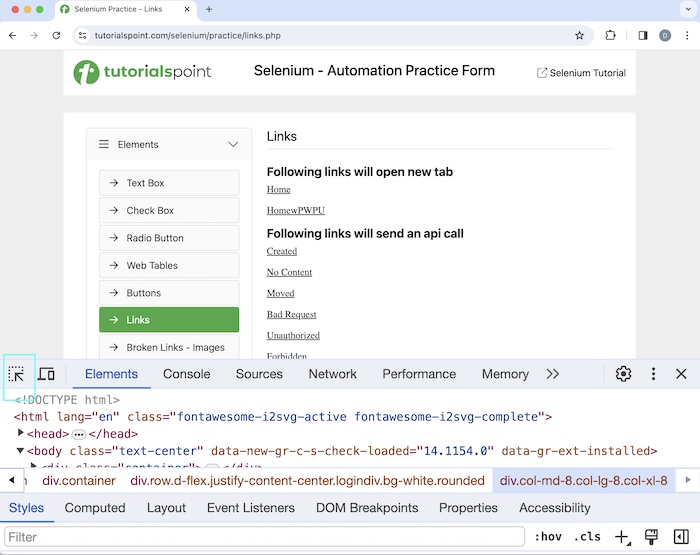
一旦我们单击并指向箭头指向“错误请求”超链接,其 HTML 代码就会可见,反映了锚标记名称(称为“a”并用 <> 括起来)和 href 属性。
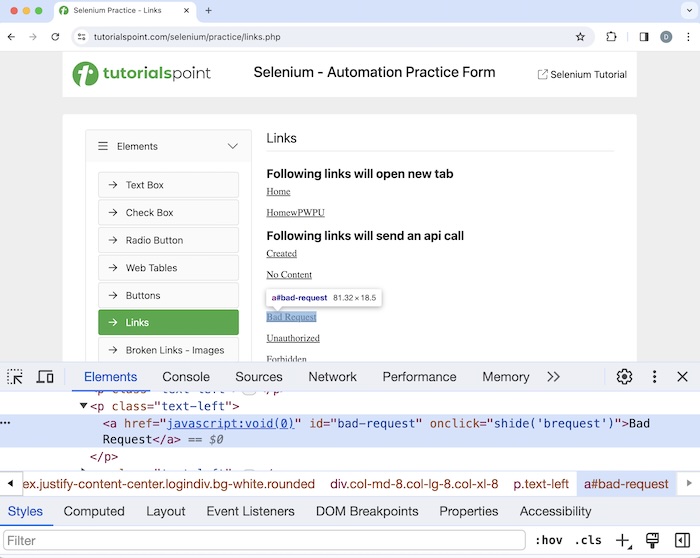
让我们以上面页面的示例为例,我们首先使用 findElements() 方法计算链接的总数,然后单击特定的链接,例如 错误请求。单击该链接后,我们将收到消息 链接已使用状态 400 和状态文本错误请求进行响应。
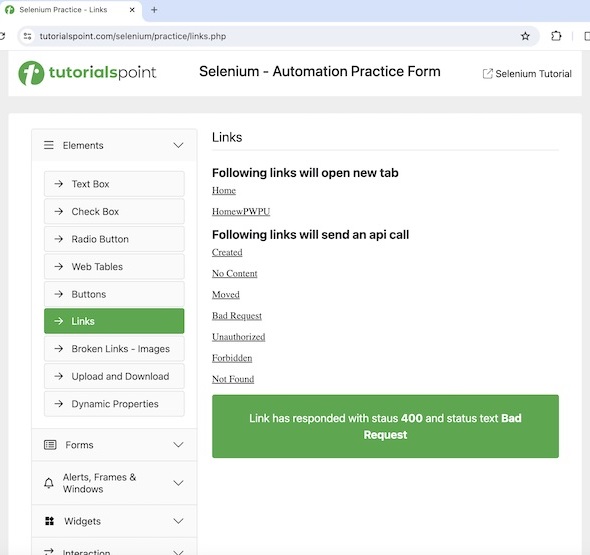
语法
WebDriver driver = new ChromeDriver(); List<WebElement> totalLnks = driver.findElements(By.tagName("a") );
示例
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebElement; public class TotalLnks { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver() ; // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage where we will count the links driver.get("https://tutorialspoint.com/selenium/practice/links.php" ) ; // Retrieve all links using locator By.tagName and storing in List List<WebElement> totalLnks = driver.findElements(By.tagName("a") ); System.out.println( "Total number of links: " + totalLnks.size() ) ; // Running loop through list of web elements for( int j = 0; j < totalLnks.size(); j ++){ if( totalLnks.get(j).getText().equalsIgnoreCase("Bad Request") ) { totalLnks.get(j).click() ; break ; } } // get text WebElement t = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/div[4]")); System.out.println("Text is: " + t.getText()); // Closing browser driver.quit(); } }
输出
Total number of links: 42 Text is: Link has responded with status 400 and status text Bad Request
在上面的示例中,我们计算了网页上的链接总数,并在控制台中收到了消息 - 链接总数:42,然后在执行单击后获取生成的文本,并显示消息 - 文本是:链接已使用状态 400 和状态文本错误请求进行响应。
findElements 方法无匹配元素
让我们举一个例子并实现一个代码,在该代码中我们将看到 findElements() 方法返回的列表可能包含 0 个元素,如果使用给定定位器值没有 0 个元素。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; public class FindElement { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify element driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // Retrieve all elements using By.class name ul and storing in List List<WebElement> total = driver.findElements(By.tagName("input")); System.out.println( "Total number of elements with tagname input: " + total.size() ) ; //Closing browser driver.quit(); } }
输出
Total number of elements with tagname input: 0
在上面的示例中,我们计算了所有具有标记名称为 input 的元素,并在控制台中收到了消息 - 具有标记名称 input 的元素总数:0(因为没有匹配的元素)。
findElement 方法无匹配元素
让我们举一个例子并实现一个代码,在该代码中我们将收到 findElement() 方法返回的 NoSuchElement 异常,如果使用给定定位器值没有 0 个元素。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class FindElementWithException { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify element driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // Retrieve element using By.class name ul WebElement e = driver.findElement(By.className("ul")); System.out.println( "Element is : " + e); // Closing browser driver.quit(); } }
输出
Exception in thread "main" org.openqa.selenium.NoSuchElementException: no such element: Unable to locate element: {"method":"css selector","selector":".ul"} (Session info: chrome=121.0.6167.160) For documentation on this error, please visit: https://selenium.net.cn/documentation/webdriver/troubleshooting/errors#no-such-element-exception Process finished with exit code 1
在上面的示例中,我们收到了 进程已完成,退出代码为 1,表示代码执行失败。此外,我们还获得了 NoSuchElementException(因为网页上没有元素具有匹配的定位器值)。
findElements 和 findElement 方法的区别
因此,findElement() 和 findElements() 之间的基本区别如下所示 -
findElement() 方法返回一个 Web 元素,而 findElements() 方法返回 Web 元素列表。
如果不存在匹配元素,则 findElement() 方法将抛出 NoSuchElementException,而 findElements() 方法将返回一个大小为 0 的列表。
activeElement 方法
在网页上的所有元素中,我们可以使用 activeElement() 方法获取获得焦点的元素。
让我们以上面页面的示例为例,我们首先使用 sendKeys() 方法在输入框中输入文本 Selenium。然后使用 activeElement() 方法获取焦点元素的属性(在将驱动程序的上下文切换到焦点元素后完成)。
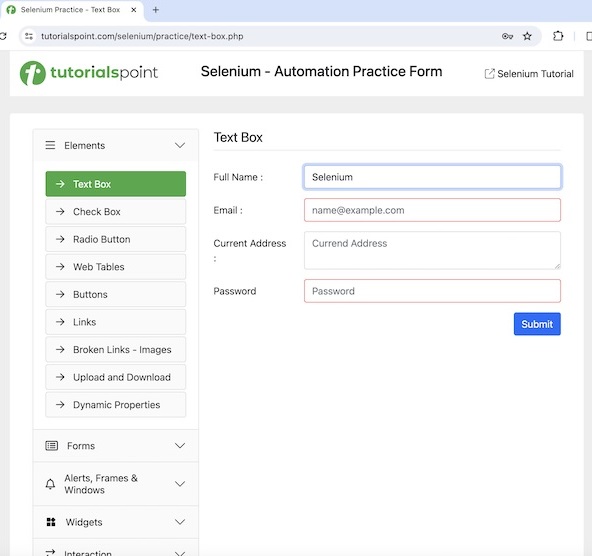
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingActivesElement { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching URL driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // enter text in input box e.sendKeys("Selenium"); // Get the value of element in focus after switching driver context String a = driver.switchTo().activeElement().getAttribute("value"); System.out.println("Value entered: " + a); // Closing browser driver.quit(); } }
输出
Value entered: Selenium
在上面的示例中,我们首先在输入框中输入了文本 Selenium,并在控制台中检索了当前焦点元素的值,并显示消息 - 输入的值:Selenium(即输入的值)。
结论
这总结了我们对 Selenium Webdriver 定位器教程的全面介绍。我们首先介绍了 findElement 方法、使用嵌套 findElement 识别元素、使用 findElements 方法识别链接、findElements 方法无匹配元素、findElement 方法无匹配元素、findElements 和 findElement 方法的区别、activeElement 方法以及说明如何在 Selenium Webdriver 中使用定位器的示例。这使您对 Selenium Webdriver 定位器有了深入的了解。明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并扩展您的视野。