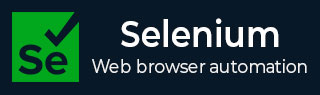
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 及其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - Edge 选项
EdgeOptions 是 Selenium Webdriver 中的一个特定类,它有助于处理仅适用于 Edge 驱动程序的选项。它有助于在 Edge 上运行自动化测试时修改浏览器的设置和功能。EdgeOptions 类扩展了另一个称为 MutableCapabilities 类的类。
EdgeOptions 类在最新版本的 Selenium 中可用。默认情况下,Selenium Webdriver 从一个新的浏览器配置文件开始,该配置文件没有任何关于 Cookie、历史记录等的预定义设置。
使用 EdgeOptions 添加 Edge 扩展
使用 EdgeOptions 类启动带有 Selenium IDE 扩展的 Edge 浏览器。Edge 扩展应具有 .crx 扩展名。我们将保留扩展的 .crx 文件,并将其放置在项目中的 Resources 文件夹下。
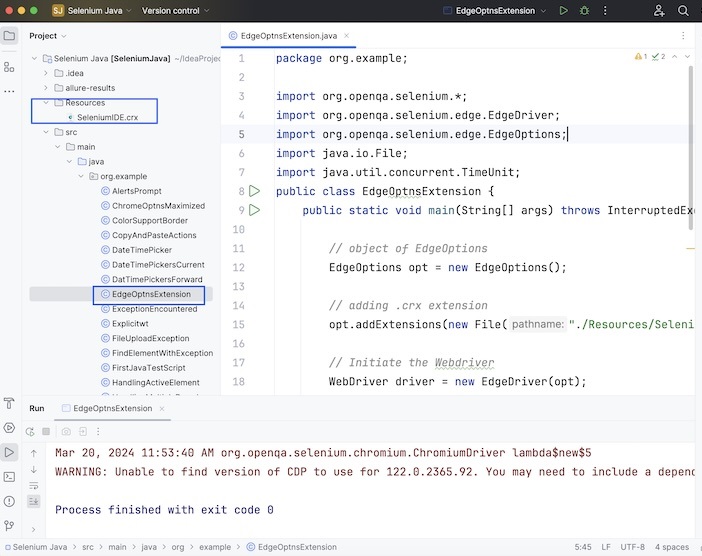
示例
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.edge.EdgeDriver; import org.openqa.selenium.edge.EdgeOptions; import java.io.File; import java.util.concurrent.TimeUnit; public class EdgeOptnsExtension { public static void main(String[] args) throws InterruptedException { // object of EdgeOptions EdgeOptions opt = new EdgeOptions(); // adding .crx extension to project structure opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // Initiate the Webdriver WebDriver driver = new EdgeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://tutorialspoint.com/selenium/practice/register.php"); } }
它将显示以下输出 -
Process finished with exit code 0
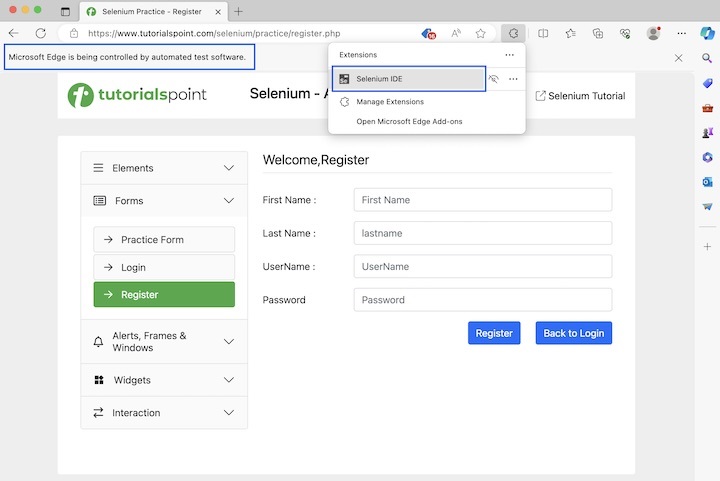
Edge 浏览器已启动 Selenium IDE 扩展,以及信息栏“**Microsoft Edge 正在由自动化测试软件控制**”。
使用 EdgeOptions 禁用弹出窗口阻止程序
让我们举一个例子,我们将打开禁用弹出窗口阻止程序的 Edge 浏览器。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.edge.EdgeDriver; import org.openqa.selenium.edge.EdgeOptions; import java.io.File; import java.util.List; import java.util.concurrent.TimeUnit; public class EdgeOptnsBlockPopUp { public static void main(String[] args) throws InterruptedException { // object of EdgeOptions EdgeOptions opt = new EdgeOptions(); // adding .crx extension to project structure opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable pop-up blocker opt.setExperimentalOption("excludeSwitches", List.of("disable-popup-blocking")); // Initiate the Webdriver WebDriver driver = new EdgeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://tutorialspoint.com/selenium/practice/register.php"); } }
它将显示以下输出 -
Process finished with exit code 0
在上面的示例中,Edge 浏览器已启动 Selenium IDE 扩展,并且阻止了弹出窗口。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
使用 EdgeOptions 打开最大化浏览器
在此示例中,我们将以最大化大小打开并启动 Edge 浏览器。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.edge.EdgeDriver; import org.openqa.selenium.edge.EdgeOptions; import java.io.File; import java.util.List; import java.util.concurrent.TimeUnit; public class EdgeOptnsMaximized { public static void main(String[] args) throws InterruptedException { // object of EdgeOptions EdgeOptions opt = new EdgeOptions(); // adding .crx extension opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable pop-up blocker opt.setExperimentalOption("excludeSwitches", List.of("disable-popup-blocking")); // open browser in maximized mode opt.addArguments("--start-maximized"); // Initiate the Webdriver WebDriver driver = new EdgeDriver(opt); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/slider.php"); } }
它将显示以下输出 -
Process finished with exit code 0
在上面的示例中,我们观察到 Edge 浏览器已启动 Selenium IDE 扩展,并带有弹出窗口阻止程序,并且处于最大化浏览器状态。
使用 EdgeOptions 在无头浏览器中打开
在此示例中,我们将以 Edge 无头模式打开并启动应用程序。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.edge.EdgeDriver; import org.openqa.selenium.edge.EdgeOptions; import java.io.File; import java.util.List; import java.util.concurrent.TimeUnit; public class EdgeOptnsHeadless { public static void main(String[] args) throws InterruptedException { // object of EdgeOptions EdgeOptions opt = new EdgeOptions(); // adding .crx extension to project structure opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable pop-up blocker opt.setExperimentalOption("excludeSwitches", List.of("disable-popup-blocking")); // open in headless mode opt.addArguments("--headless=new"); // Initiate the Webdriver WebDriver driver = new EdgeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/progress-bar.php"); // getting page title System.out.println("Getting the page title in headless mode: " + driver.getTitle()); // Quitting browser driver.quit(); } }
它将显示以下输出 -
Getting the page title: Selenium Practice - Student Registration Form
在上面的示例中,我们观察到 Edge 浏览器已启动 Selenium IDE 扩展,并带有弹出窗口阻止程序,并且处于无头模式。我们还在控制台中获得了带有消息的浏览器标题 - **在无头模式下获取页面标题:Selenium 实践 - 进度条**。
结论
这总结了我们关于 Selenium Webdriver Edge 选项教程的全面内容。我们从描述 EdgeOptions 类开始,并逐步介绍了如何向 Edge 浏览器添加扩展、如何阻止弹出窗口、如何最大化浏览器以及如何使用 EdgeOptions 和 Selenium Webdriver 处理无头 Edge 浏览器执行的示例。这使您深入了解 Selenium Webdriver 中的 EdgeOptions 类。明智的做法是不断练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并扩展您的视野。