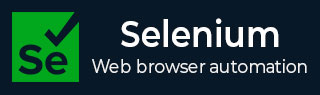
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码生成
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 截取屏幕截图
- Selenium - 录制视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 单选按钮
Selenium WebDriver 可用于处理网页上的单选按钮。在 HTML 术语中,每个单选按钮都由名为 input 的标签名标识。此外,网页上的每个单选按钮都将始终具有名为 type 的属性,其值为 radio。
HTML 中单选按钮的标识
启动浏览器(例如 Chrome),右键单击网页,然后单击“检查”按钮。要识别页面上的单选按钮,请单击如下所示突出显示的左上方箭头。
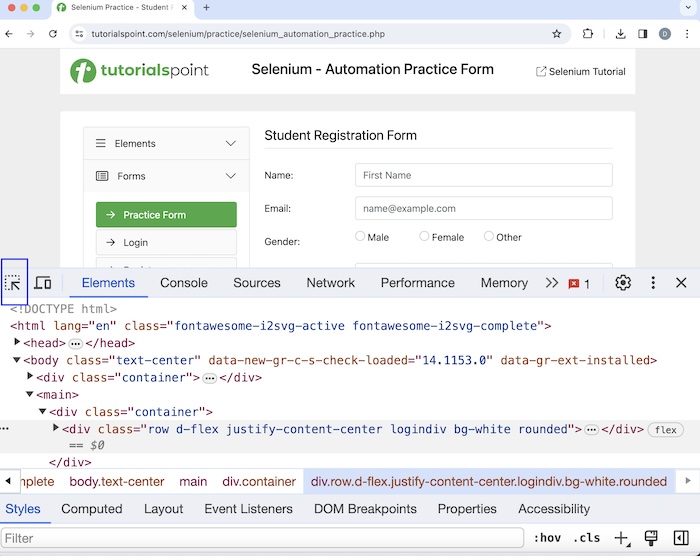
单击箭头指向单选按钮(如下图所示突出显示)后,其 HTML 代码将可见,反映了 input 标签名和 type 属性的值为 radio。
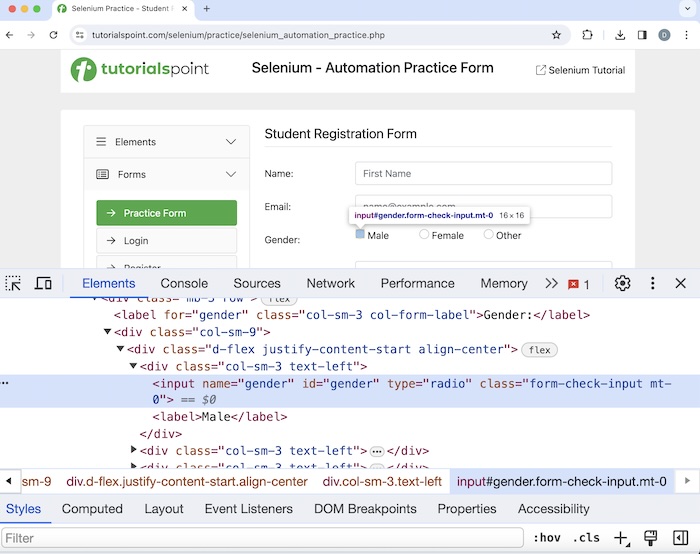
单击并选中单选按钮
让我们以以上页面为例,我们将使用 click() 方法单击其中一个单选按钮。然后,我们将使用 isSelected() 方法验证单选按钮是否被选中。此方法返回布尔值(true 或 false)。如果单选按钮被选中,isSelected() 将返回 true,否则返回 false。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingRadioButton { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify radio button driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify radio button then click WebElement radiobtn = driver.findElement(By.xpath("//*[@id='gender']")); radiobtn.click(); // verify if radio button is selected boolean result = radiobtn.isSelected(); System.out.println("Checking if a radio button is selected: " + result); // Closing browser driver.quit(); } }
输出
Checking if a radio button is selected: true Process finished with exit code 0
在上面的示例中,我们首先单击了一个单选按钮,然后在控制台中验证了该单选按钮是否被选中,消息为 - 正在检查单选按钮是否被选中:true。
最后,收到消息 进程已完成,退出代码为 0,表示代码已成功执行。
统计单选按钮数量
让我们以以下页面为例,我们将计算单选按钮的总数。在这个例子中,单选按钮总数应为 3。
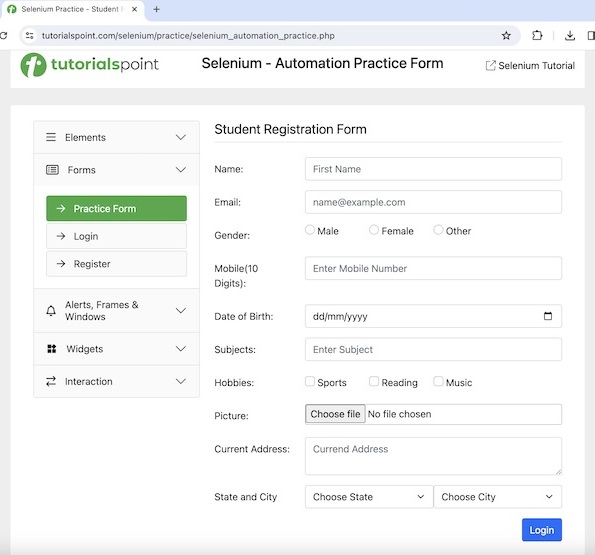
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; public class HandlingRadioButton { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify radio button driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Retrieve all radio buttons using locator and storing in List List<WebElement> totalRadioBtns = driver.findElements(By.xpath("//input[@type='radio']")); // count number of radio buttons int count = totalRadioBtns.size(); System.out.println("Count the radio buttons: " + count); // Closing browser driver.quit(); } }
输出
Count the radio buttons: 3
在上面的示例中,我们计算了网页上单选按钮的总数,并在控制台中收到消息 - 单选按钮计数:3。
验证单选按钮
让我们以上述网页为例,我们将对单选按钮执行一些验证。首先,我们将使用 isEnabled() 方法检查单选按钮是否启用/禁用。此外,我们将分别使用 isDisplayed() 和 isSelected() 方法验证它是否显示和选中/未选中。
这些方法返回布尔值(true 或 false)。如果单选按钮被选中、启用和显示,则返回 true,否则返回 false。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ValidateRadioButton { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will click radio button driver.get("https://tutorialspoint.com/selenium/selenium_automation_practice.htm"); // identify radio button then click WebElement radiobtn = driver.findElement (By.xpath("//*[@id='practiceForm']/div[3]/div/div/div[2]/input")); radiobtn.click(); // verify if radio button is selected boolean result = radiobtn.isSelected(); System.out.println("Checking if a radio button is selected: " + result); // verify if radio button is displayed boolean result1 = radiobtn.isDisplayed(); System.out.println("Checking if a radio button is displayed: " + result1); // verify if radio button is enabled boolean result2 = radiobtn.isEnabled(); System.out.println("Checking if a radio button is enabled: " + result2); // identify another radio button WebElement radiobtn1 = driver.findElement(By.xpath("//*[@id='gender']")); // verify if radio button is not selected boolean result3 = radiobtn1.isSelected(); System.out.println("Checking if the other radio button is unselected: " + result3); // Closing browser driver.quit(); } }
输出
Checking if a radio button is selected: true Checking if a radio button is displayed: true Checking if a radio button is enabled: true Checking if the other radio button is unselected: false
在上面的示例中,我们验证了一个单选按钮是否显示、启用和选中,并在控制台中收到以下消息:正在检查单选按钮是否被选中:true,正在检查单选按钮是否显示:true,正在检查单选按钮是否启用:true 和 正在检查另一个单选按钮是否未选中:false。
结论
本教程全面介绍了 Selenium WebDriver 单选按钮。我们从描述 HTML 中单选按钮的标识开始,并通过示例来说明如何在 Selenium WebDriver 中处理单选按钮。这将为您提供 Selenium WebDriver 单选按钮的深入知识。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。