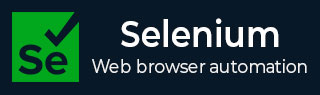
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - Chrome 选项
ChromeOptions 是 Selenium WebDriver 中的一个特定类,用于处理仅适用于 Chrome 驱动的选项。它有助于在 Chrome 上运行自动化测试时修改浏览器的设置和功能。ChromeOptions 类扩展了另一个称为 MutableCapabilities 的类。
ChromeOptions 类从 Selenium 3.6 版本开始添加。Selenium WebDriver 默认情况下以全新的浏览器配置文件开始,没有任何预定义的 Cookie、历史记录等设置。
使用 ChromeOptions 添加 Chrome 扩展程序
让我们来看一个例子,我们将使用 Selenium IDE 扩展程序打开 Chrome 浏览器。Chrome 扩展程序应该具有 .crx 文件。在本例中,我们将获取 Selenium IDE Chrome 扩展程序的 .crx 文件,并将其放置在测试项目中的 Resources 文件夹下。
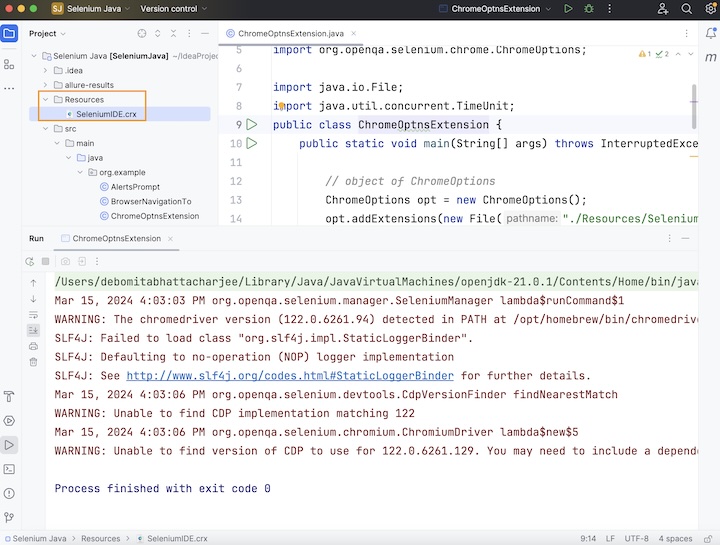
示例
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.concurrent.TimeUnit; public class ChromeOptnsExtension { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // adding .crx extension opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://tutorialspoint.com/selenium/practice/register.php"); } }
输出
Process finished with exit code 0
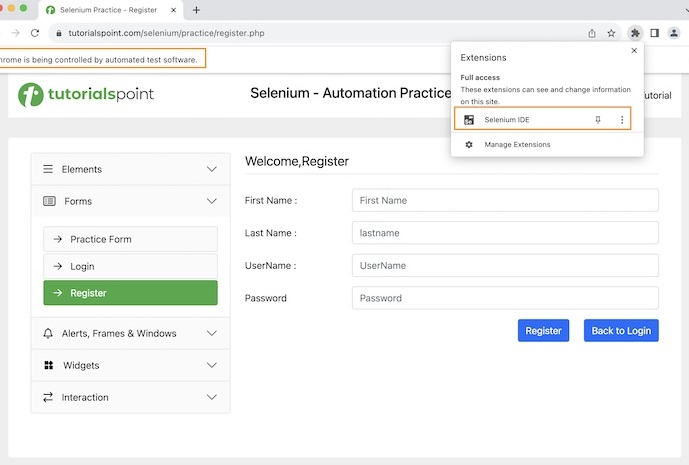
Chrome 浏览器已打开 Selenium IDE 扩展程序,并显示信息栏 **Chrome is being controlled by automated test software**。
使用 ChromeOptions 禁用信息栏
在前面的示例中,我们获得了一个包含文本 **Chrome is being controlled by automated test software** 的信息栏,但是我们可以使用 ChromeOptions 类禁用此信息栏。
示例
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.Collections; import java.util.concurrent.TimeUnit; public class ChromeOptns { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // adding .crx extensions opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable information bar opt.setExperimentalOption("excludeSwitches", Collections.singletonList("enable-automation")); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with disabling information bar driver.get("https://tutorialspoint.com/selenium/practice/register.php"); } }
输出
Process finished with exit code 0
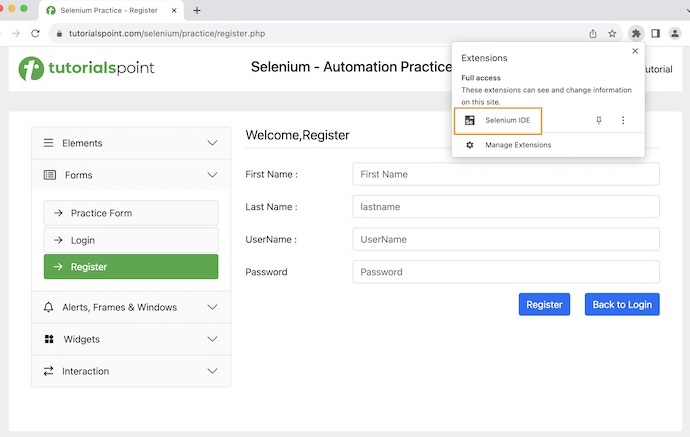
Chrome 浏览器已启动 Selenium IDE 扩展程序,没有信息栏。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
使用 ChromeOptions 打开最大化浏览器
在本例中,我们将以最大化模式在 Chrome 浏览器中打开并启动应用程序。
示例
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.Collections; import java.util.concurrent.TimeUnit; public class ChromeOptnsMaximized { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // adding .crx extensions opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // disable information bar opt.setExperimentalOption("excludeSwitches", Collections.singletonList("enable-automation")); // open browser in maximized opt.addArguments("--start-maximized"); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://tutorialspoint.com/selenium/practice/register.php"); // quitting browser driver.quit(); } }
输出
Process finished with exit code 0
在上面的示例中,我们观察到 Chrome 浏览器已启动 Selenium IDE 扩展程序,在最大化浏览器中没有信息栏 **Chrome is being controlled by automated test software**。
使用 ChromeOptions 处理 SSL 证书
要在 Chrome 中处理 SSL 证书,可以使用 ChromeOptions 类和 DesiredCapabilities 类。要使 DesiredCapabilities 的功能可用于 ChromeOptions,可以使用 merge 方法。
示例
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.remote.CapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; import java.util.concurrent.TimeUnit; public class SSLErrorInChrome { public static void main(String[] args) throws InterruptedException { // Desired Capabilities class to profile Chrome DesiredCapabilities dc = new DesiredCapabilities(); dc.setCapability(CapabilityType.ACCEPT_INSECURE_CERTS, true); // Chrome Options class ChromeOptions opt = new ChromeOptions(); // merging browser capabilities with options opt.merge(dc); // Initiate the Webdriver with options WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // launch application driver.get("https://expired.badssl.com"); // obtain browser title System.out.println("Browser title in Chrome: " + driver.getTitle()); // quit the browser driver.quit(); } }
输出
Browser title in Chrome: Privacy error
结论
本教程全面介绍了 Selenium WebDriver Chrome 选项。我们首先描述了 ChromeOptions 类,然后逐步讲解了如何向 Chrome 浏览器添加扩展程序、如何禁用信息栏、如何最大化浏览器以及如何在 Selenium WebDriver 和 ChromeOptions 的帮助下处理 SSL 证书错误。这使您能够深入了解 Selenium WebDriver 中的 ChromeOptions 类。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。