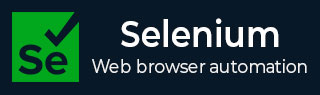
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - 断言/验证方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium - LogExpert 日志记录
Selenium Webdriver 可用于在测试执行期间记录信息。日志记录主要用于提取有关执行过程的信息。
为什么在 Selenium 中使用日志记录?
由于以下原因,日志记录是在 Selenium 中编写测试时的一个重要步骤:
- 日志记录有助于更快地调试故障。此外,如果设置了日志级别,则更容易对故障进行分类。
- 大多数日志记录框架都是免费且开源的,我们可以在各种级别设置和抑制日志,并发挥应用程序的最佳性能。
不同的日志级别
日志记录的第一步是启用日志记录,并针对每个类使用默认设置。让我们首先启用默认日志记录,该日志记录适用于使用根记录器的所有记录器。
Logger logger = Logger.getLogger("");
由于日志记录是针对类设置的,因此我们可以设置适用于类的日志级别。
((RemoteWebDriver) driver).setLogLevel(Level.INFO); Logger.getLogger(SeleniumManager.class.getName()) .setLevel(Level.SEVERE);
总共有七个日志级别 - SEVERE、WARNING、INFO、CONFIG、FINE、FINER 和 FINEST。INFO 日志级别是默认级别,这意味着我们的代码中没有任何操作项需要处理,并且纯粹用于信息目的。
Logger logger = Logger.getLogger(""); logger.setLevel(Level.INFO);
并非始终需要所有日志级别,因此我们可以使用 setLevel() 方法根据级别过滤日志。
Logger logger = Logger.getLogger(""); logger.setLevel(Level.WARNING);
在上面的示例中,已设置 WARNING 日志级别,这意味着需要处理某些操作,特别是对于在我们的代码中使用已弃用版本。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 1 - 警告日志级别
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.remote.RemoteWebDriver; import java.util.concurrent.TimeUnit; import java.util.logging.Level; public class LoggingLvl { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // enabling log levels to Warning ((RemoteWebDriver) driver).setLogLevel(Level.WARNING); //adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting current URL System.out.println("Getting the Current URL: " + driver.getCurrentUrl()); // quitting the browser driver.quit(); } }
输出
Feb 15, 2024 4:41:42 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: setTimeout [510e0fcbc35b47f7637445d1b69bedc2, setTimeout {implicit=12000}] Feb 15, 2024 4:41:42 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: setTimeout (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: null) Feb 15, 2024 4:41:42 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: get [510e0fcbc35b47f7637445d1b69bedc2, get {url=https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php}] Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: get (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: null) Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: getCurrentUrl [510e0fcbc35b47f7637445d1b69bedc2, getCurrentUrl {}] Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: getCurrentUrl (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php) Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executing: quit [510e0fcbc35b47f7637445d1b69bedc2, quit {}] Getting the Current URL: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php Feb 15, 2024 4:41:43 PM org.openqa.selenium.remote.RemoteWebDriver log WARNING: Executed: quit (Response: SessionID: 510e0fcbc35b47f7637445d1b69bedc2, Status: 0, Value: null) Process finished with exit code 0
在上面的示例中,我们获得了 WARNING 日志级别以及浏览器标题,消息为获取当前 URL:Selenium 自动化实践表单。
示例 2 - 精细日志级别
日志信息还包含用于查找特定问题并进行更正的调试信息。这可以通过将日志级别设置为 FINE 来完成。
Logger logger = Logger.getLogger(""); logger.setLevel(Level.FINE);
示例 3 - 严重日志级别
让我们再举一个例子,我们将日志级别设置为 SEVERE。
使用 SEVERE 日志级别的代码实现。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.remote.RemoteWebDriver; import java.util.concurrent.TimeUnit; import java.util.logging.Level; public class LoggingLvls { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // enabling log levels to SEVERE ((RemoteWebDriver) driver).setLogLevel(Level.SEVERE); //adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // getting current URL System.out.println("Getting the Current URL: " + driver.getCurrentUrl()); //quitting the browser driver.quit(); } }
输出
在上面的示例中,我们获得了 SEVERE 日志级别以及浏览器标题,消息为获取当前 URL:Selenium 自动化实践表单。
在单独的文件中获取日志级别
可以通过使用处理程序将控制台中生成的日志写入另一个文件。默认情况下,所有日志都放置在 System.err 中。
示例
package org.example; import org.openqa.selenium.WebDriver; import java.io.IOException; import java.util.logging.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import java.util.logging.Handler; public class LoggingLvelFile { public static void main(String[] args) throws InterruptedException, IOException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // enabling log levels to WARNING Logger logger = Logger.getLogger(""); logger.setLevel(Level.WARNING); // output logging to another file Logs1.xml Handler handler = new FileHandler("Logs1.xml"); logger.addHandler(handler); //adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting current URL System.out.println("Getting the Current URL: " + driver.getCurrentUrl()); //quitting the browser driver.quit(); } }
输出
Getting the Current URL: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php Process finished with exit code 0
在上面的示例中,我们在控制台中检索了带有消息的浏览器标题 - 获取当前 URL:Selenium 自动化实践表单。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
此外,项目目录中会创建一个 Logs1.xml 文件,其中包含日志。
结论
本教程全面介绍了 Selenium WebDriver 日志记录和 LogExpert。我们首先介绍了在 Selenium 中使用日志记录的原因,并介绍了不同的日志级别,并通过一个示例说明了如何将其与 Selenium 一起使用。
这使您对 LogExpert 日志记录有了深入的了解。明智的做法是不断练习您所学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并拓宽您的视野。