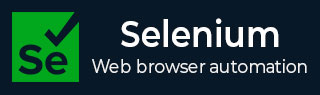
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 Iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 输入框
Selenium Webdriver 可以用来处理输入框(也称为文本框)。在 HTML 术语中,每个输入框都由名为 input 的标签名标识。
HTML 中输入框的识别
打开任何浏览器,例如 Chrome,右键单击网页,然后单击“检查”按钮。然后,将显示该页面的完整 HTML 代码。要检查页面上的输入框,请单击位于 HTML 代码顶部的左侧向上箭头,如下面的图像中突出显示的那样。
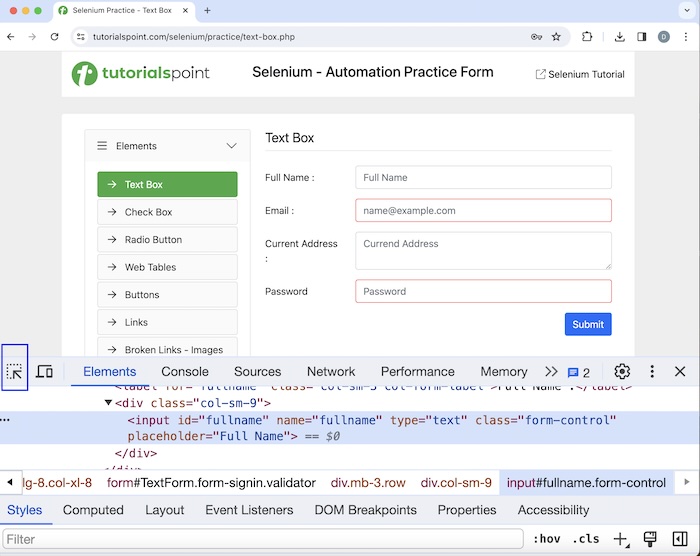
一旦我们单击并将箭头指向输入框(在下面的图像中突出显示),其 HTML 代码就会可见,反映输入标签名(括在 <> 中)。
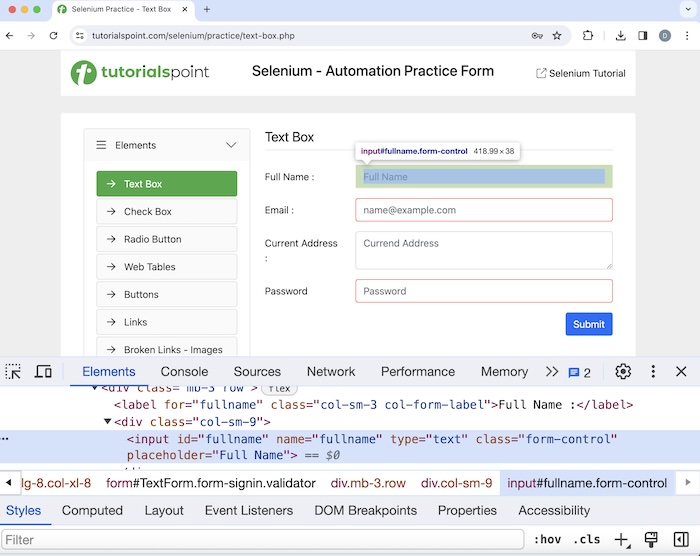
示例 1- 使用 sendKeys 输入文本
让我们以上面页面的一个示例为例,我们首先使用 sendKeys() 方法在 输入框 中输入一些文本。然后,我们使用 clear() 方法擦除输入的文本。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.edge.EdgeDriver; import java.util.concurrent.TimeUnit; public class EnterText{ public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new EdgeDriver(); // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the input box WebElement elm = driver.findElement(By.xpath("//*[@id='fullname']")); // enter text elm.sendKeys("Java"); // Get the value String txt = driver.findElement(By.xpath("//*[@id='fullname']")).getAttribute("value"); System.out.println("Entered text: " + text); // clear the text entered elm.clear(); // Get no text String txt1 = driver. findElement(By.xpath("//*[@id='fullname']")).getAttribute("value"); System.out.println("Get text after clearing: " + txt1); // Closing browser driver.quit(); } }
输出
Entered text: Java Get text after clearing: Process finished with exit code 0
在上面的示例中,我们首先在编辑框中输入了文本 Java,并在控制台中获取了输入的值,消息为 - 输入的文本:Java。然后清除输入的值,并在删除文本后在编辑框中没有获取任何值,并在控制台中收到消息:清除后获取文本:。
最后,收到消息 进程已完成,退出代码为 0,表示代码成功执行。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 2 - 使用 Actions 类输入文本
我们还可以将 sendKeys() 方法与 Selenium 中的 Actions 类 一起使用。我们将采用上面讨论的相同示例并查看实现。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class HandlingInputBoxWithActions { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box to enter driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // enter text in input box using Actions class Actions action = new Actions(driver); action.sendKeys(e, "SeleniumS").perform(); // Get the value entered String text = driver. findElement(By.xpath("//*[@id='fullname']")).getAttribute("value"); System.out.println("Entered text with Actions class is: " + text); // clear the text entered e.clear(); // Get no text after clearing text String text1 = driver. findElement(By.xpath("//*[@id='fullname']")).getAttribute("value"); System.out.println("Get text after clearing: " + text1); // Closing browser driver.quit(); } }
输出
Entered text with Actions class is: SeleniumS Get text after clearing: Process finished with exit code 0
在上面的示例中,我们首先在输入框中输入了文本 SeleniumS,并在控制台中获取了输入的值,消息为 - 使用 Actions 类输入的文本为:SeleniumS。然后清除输入的值,并在清除文本后在输入框中没有获取任何值。因此,我们还在控制台中收到了消息:清除后获取文本:。
示例 3 - 使用 JavaScriptExecutor 输入文本
我们还可以使用 JavaScriptExecutor 在 Selenium 中的输入框中输入文本。我们将采用上面讨论的相同示例。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingInputBoxWithJS { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box to enter driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // enter text in input box using JavascriptExecutor JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver; javascriptExecutor.executeScript("arguments[0].setAttribute('value', 'Selenium Java')", e); // Get the value entered String text = driver.findElement(By.xpath("//*[@id='fullname']")).getAttribute("value"); System.out.println("Entered text with JavaScript Executor is: " + text); // clear the text entered e.clear(); // Get no text after clearing text String text1 = driver. findElement(By.xpath("//*[@id='fullname']")).getAttribute("value"); System.out.println("Get text after clearing: " + text1); // Closing browser driver.quit(); } }
输出
Entered text with JavaScript Executor is: Selenium Java Get text after clearing: Process finished with exit code 0
在上面的示例中,我们首先在输入框中输入了文本 Selenium Java,并在控制台中获取了输入的值,消息为 - 使用 JavaScript Executor 输入的文本为:Selenium Java。然后清除输入的值,并在输入框中没有获取任何值,然后在控制台中收到消息:清除后获取文本:。
结论
这总结了我们对 Selenium Webdriver 输入框教程的全面介绍。我们从描述 HTML 中输入框的识别开始,并通过示例说明如何使用 sendKeys 方法、Actions 类和 Selenium Webdriver 中的 JavaScript 在输入框中输入文本。这使您能够深入了解 Selenium Webdriver 输入框。明智的做法是不断练习您所学到的知识,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。