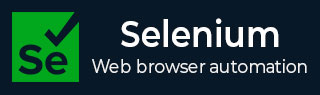
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 定位策略
启动应用程序后,用户会与页面上的元素进行交互,例如单击链接/按钮、在输入框中键入文本、从下拉列表中选择选项等等,以创建自动化测试用例。第一步是使用其属性识别元素。可以使用定位器(例如 id、name、class name、xpath、css、tagname、link text 和 partial link)进行此识别。
Id 定位器
元素的 id 属性可用于识别它。在 Java 中,方法 findElement(By.id("<id 属性的值>")) 用于查找具有 id 属性值的元素。使用此方法,应识别第一个与 id 属性值匹配的元素。如果不存在 id 属性值相同的元素,则会抛出 NoSuchElementException。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.id("id value”));
让我们看一下下面图片中突出显示的姓名旁边的输入框的 html 代码:
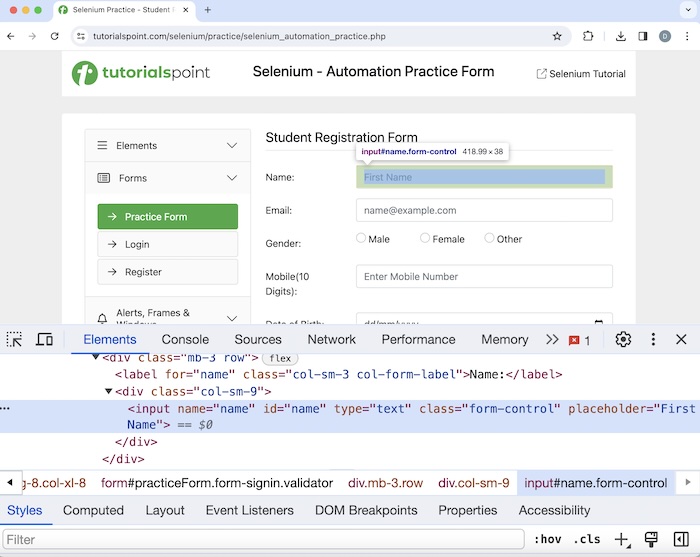
上图中突出显示的编辑框具有一个 id 属性,其值为name。让我们尝试在此编辑框中输入文本Selenium。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorID { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box enter text driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the search box with id locator then enter text Selenium WebElement i = driver.findElement(By.id("name")); i.sendKeys("Selenium"); // Get the value entered String text = i.getAttribute("value"); System.out.println("Entered text is: " + text); // Close browser driver.quit(); } }
它将显示以下输出:
Entered text is: Selenium Process finished with exit code 0
输出显示消息 - 进程退出代码为 0,表示上述代码已成功执行。此外,在控制台中接收到了在编辑框中输入的值(从 getAttribute 方法获得) - Selenium。
Name 定位器
元素的 name 属性可用于识别它。在 Java 中,方法 findElement(By.name("<name 属性的值>")) 用于查找具有 name 属性值的元素。使用此方法,应识别第一个与 name 属性值匹配的元素。如果不存在 name 属性值相同的元素,则会抛出 NoSuchElementException。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.name("name value"));
让我们研究一下下面图片中与之前讨论的相同输入框的 html 代码:
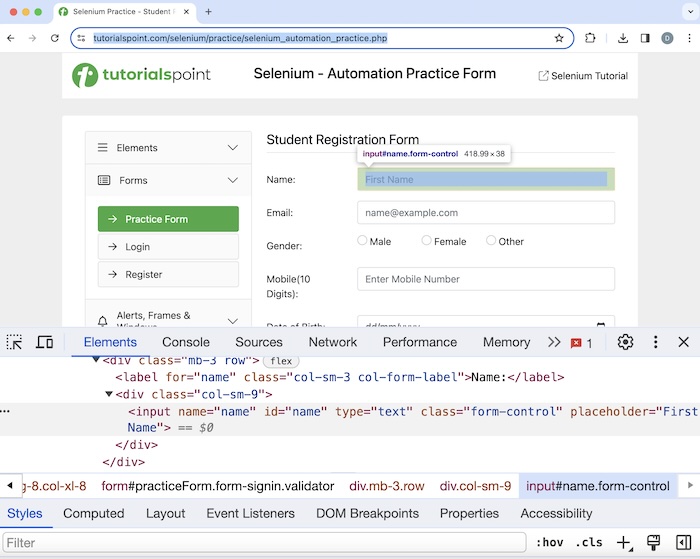
上图中突出显示的编辑框具有一个 name 属性,其值为name。让我们在此编辑框中输入文本Selenium。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorName { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify edit box enter text driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the search box with name locator to enter text WebElement i = driver.findElement(By.name("name")); i.sendKeys("Selenium"); // Get the value entered String text = i.getAttribute("value"); System.out.println("Entered text is: " + text); // Close browser driver.quit(); } }
它将显示以下输出:
Entered text is: Selenium
在控制台中打印了编辑框中输入的值 - Selenium。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
Class Name 定位器
元素的 class name 属性可用于识别它。在 Java 中,方法 findElement(By.className("<class name 属性的值>")) 用于查找具有 class name 属性值的元素。使用此方法,应识别第一个与 class name 属性值匹配的元素。如果不存在 name 属性值相同的元素,则会抛出 NoSuchElementException。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.className("class name value"));
让我们看一下下面图片中突出显示的点击我的 html 代码:
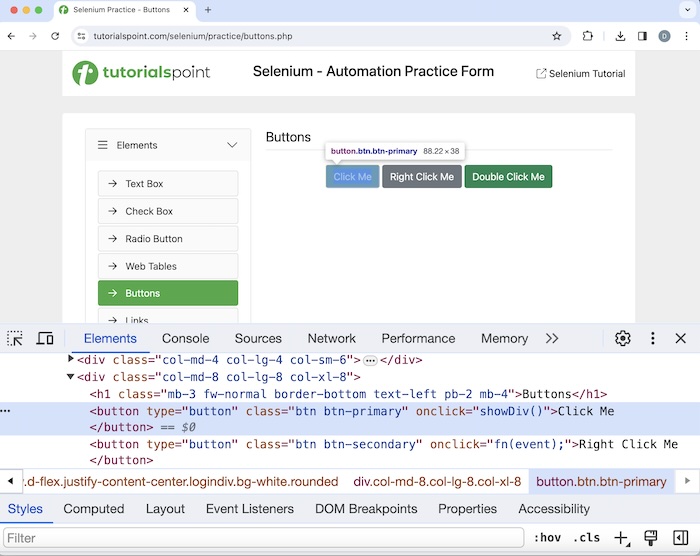
它具有一个 class name 属性,其值为btn-primary。单击该元素后,我们将在页面上获得您已执行动态点击。
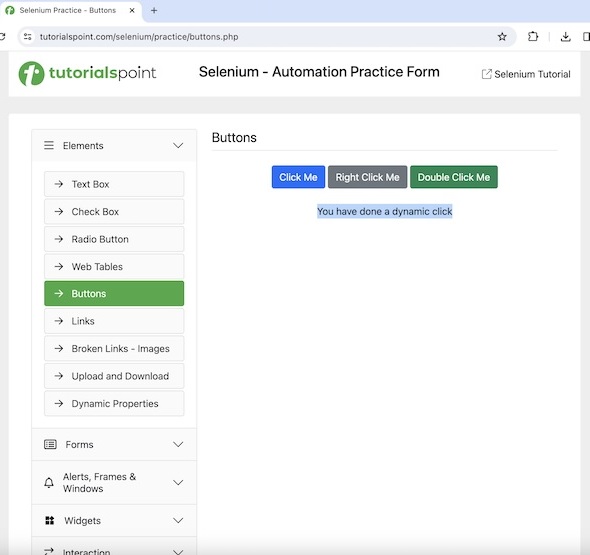
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorClassName { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage where we will identify button to click driver.get("https://tutorialspoint.com/selenium/practice/buttons.php"); // Identify button with class name to click WebElement i = driver.findElement(By.className("btn-primary")); i.click(); // Get text after click WebElement e = driver.findElement(By.xpath("//*[@id='welcomeDiv']")); String text = e.getText(); System.out.println("Text is: " + text); // Closing browser driver.quit(); } }
它将显示以下输出:
Text is: You have done a dynamic click
我们在单击点击我按钮后在控制台中获得了文本,消息为 - 文本为:您已执行动态点击。
TagName 定位器
元素的 tagname 可用于识别它。在 Java 中,方法 findElement(By.tagName("<tagname 的值>")) 用于查找具有 tagname 值的元素。使用此方法,应识别第一个与 tagname 值匹配的元素。如果不存在 tagname 值相同的元素,则会抛出 NoSuchElementException。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.tagName("tag name value"));
让我们获取下面图片中文本姓名旁边的输入框的 html 代码:
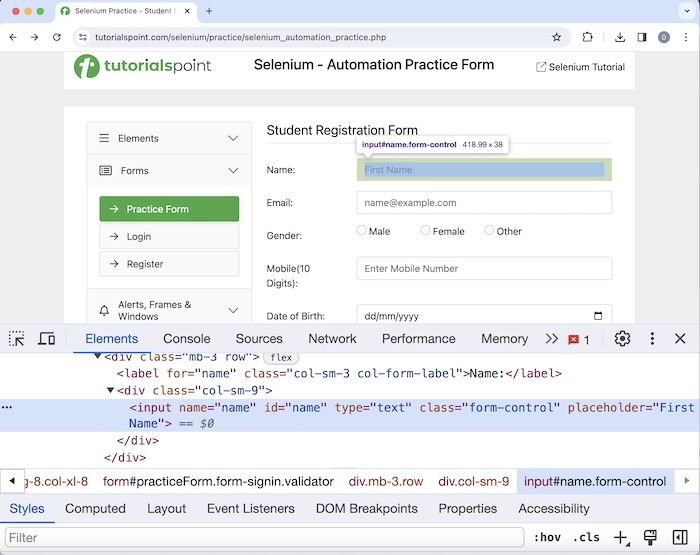
上图中突出显示的输入框的 tagname 为input。让我们尝试在该输入框中输入文本Java。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorTagName { public static void main(String[] args) throws InterruptedException { //Initiate the Webdriver WebDriver driver = new ChromeDriver(); //adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // launch a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify input box with tagname locator WebElement t = driver.findElement(By.tagName("input")); // then enter text t.sendKeys("Java"); // Get the value entered String text = t.getAttribute("value"); System.out.println("Entered text is: " + text); // Closing browser driver.quit(); } }
它将显示以下输出:
Entered text is: Java
在控制台中打印了编辑框中输入的值Java。
Link Text 定位器
链接的 link text 可用于识别它。在 Java 中,方法 findElement(By.linkText("<link text 的值>")) 用于查找具有 link text 值的链接。使用此方法,应识别第一个与 link text 值匹配的元素。如果不存在 link text 值相同的元素,则会抛出 NoSuchElementException。它主要用于在网页上定位链接。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.linkText("value of link text"));
让我们看一下下面图片中突出显示的链接已创建的 html 代码:
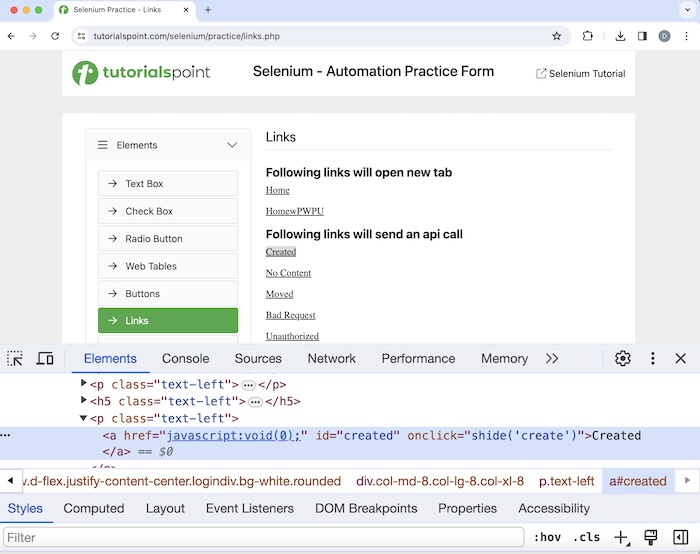
它的 link text 值为已创建。单击它后,我们将在页面上获得链接已响应,状态为 201,状态文本为已创建。
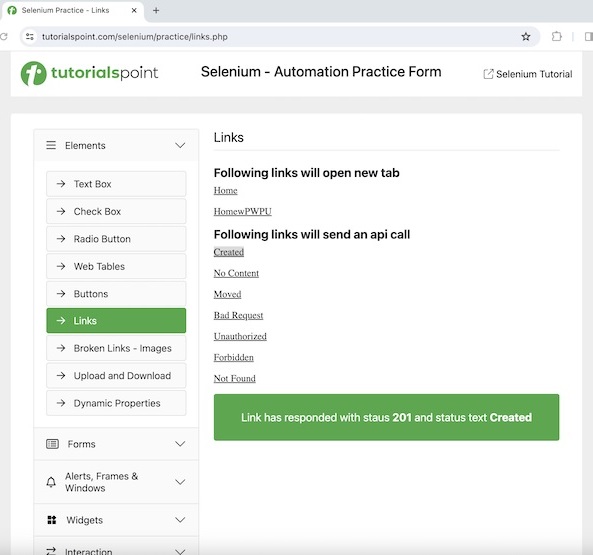
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorLinkText { public static void main(String[] args) throws InterruptedException { //Initiate the Webdriver WebDriver driver = new ChromeDriver(); //adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // launch a URL driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // Identify link with tagname link text WebElement t = driver.findElement(By.linkText("Created")); // then click t.click(); // Get the text WebElement e = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]")); String text = e.getText(); System.out.println("Text is: " + text); // Close browser driver.quit(); } }
它将显示以下输出:
Text is: Link has responded with status 201 and status text Created
我们在单击已创建链接后在控制台中获得了文本,消息为 - 链接已响应,状态为 201,状态文本为已创建。
Partial Link Text 定位器
链接的 partial link text 可用于识别它。在 Java 中,方法 findElement(By.partialLinkText(“<partial link text 的值>”)) 用于查找具有 partial link text 值的链接。使用此方法,应识别第一个与 partial link text 值匹配的元素。如果不存在 partial link text 值相同的元素,则会抛出 NoSuchElementException。它主要用于在网页上定位链接。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.partialLinkText("partial link text value"));
让我们研究一下下面图片中突出显示的链接错误请求的 html 代码:
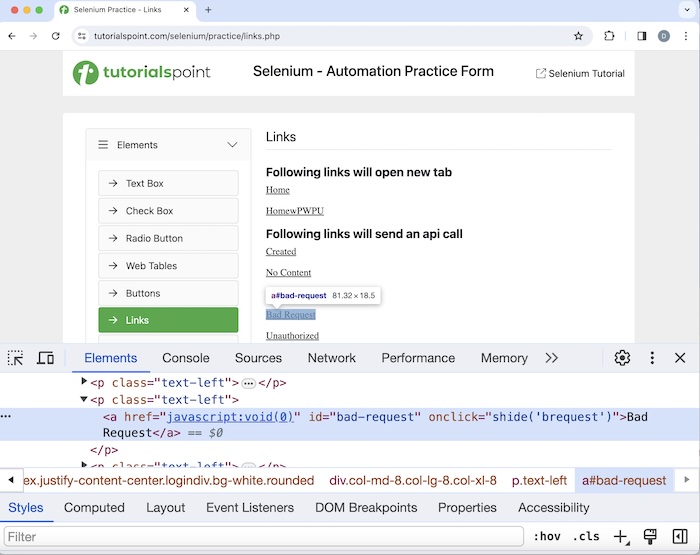
它的 link text 值为错误请求。单击它后,我们将在页面上获得文本链接已响应,状态为 400,状态文本为错误请求。
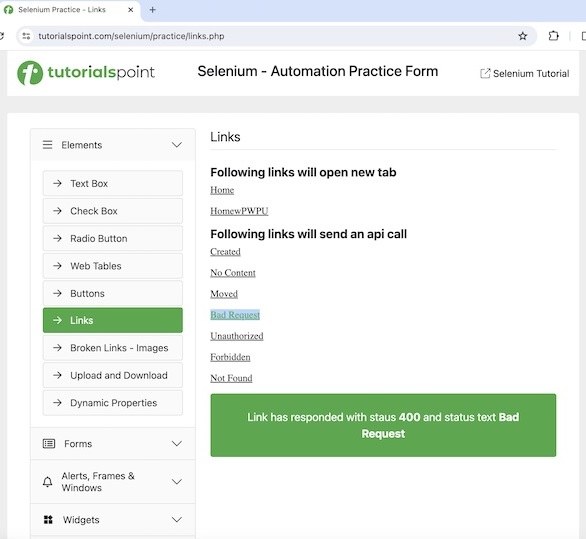
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class LocatorPartialLinkText { public static void main(String[] args) throws InterruptedException { //Initiate the Webdriver WebDriver driver = new ChromeDriver(); //adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // launch a URL driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // Identify link with tagname partial link text WebElement t = driver.findElement(By.partialLinkText("Bad")); // then click t.click(); // Get the text WebElement e = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[4]")); String text = e.getText(); System.out.println("Text is: " + text); // Closing browser driver.quit(); } }
它将显示以下输出:
Text is: Link has responded with status 400 and status text Bad Request
我们在单击错误请求链接后在控制台中获得了文本,消息为 - 链接已响应,状态为 400,状态文本为错误请求。
CSS 定位器
元素的 css 定位器值可用于识别它。在 Java 中,方法 findElement(By.cssSelector("<css 选择器的值>")) 用于查找具有 css 选择器值的元素。使用此方法,应识别第一个与 css 选择器值匹配的元素。如果不存在 css 选择器值相同的元素,则会抛出 NoSuchElementException。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.cssSelector("value of css selector"));
创建 CSS 表达式的规则
下面讨论了创建 css 表达式的规则:
- 要使用 css 识别元素,表达式应为 tagname[attribute='value']。
- 我们还可以专门使用 id 属性来创建 css 表达式。对于 id,css 表达式的格式应为 tagname#id。例如,input#txt [此处 input 是 tagname,txt 是 id 属性的值]。
- 对于 class,css 表达式的格式应为 tagname.class。例如,input.cls-txt [此处 input 是 tagname,cls-txt 是 class 属性的值]。
- 如果一个父元素有 n 个子元素,并且我们想要识别第 n 个子元素,那么 CSS 表达式应该为 nth-of-type(n)。类似地,要识别最后一个子元素,CSS 表达式应为 ul.reading li:last-child。
对于值动态变化的属性,我们可以使用 ^= 来定位属性值以特定文本开头的元素。例如,input[name^='qa'] 这里,input 是标签名,name 属性的值以 qa 开头。
对于值动态变化的属性,我们可以使用 $= 来定位属性值以特定文本结尾的元素。例如,input[class$='txt'] 这里,input 是标签名,class 属性的值以 txt 结尾。
对于值动态变化的属性,我们可以使用 *= 来定位属性值包含特定子文本的元素。例如,input[name*='nam'] 这里,input 是标签名,name 属性的值包含子文本 nam。
让我们获取如下所示 Name 标签旁边的输入框的 HTML 代码:
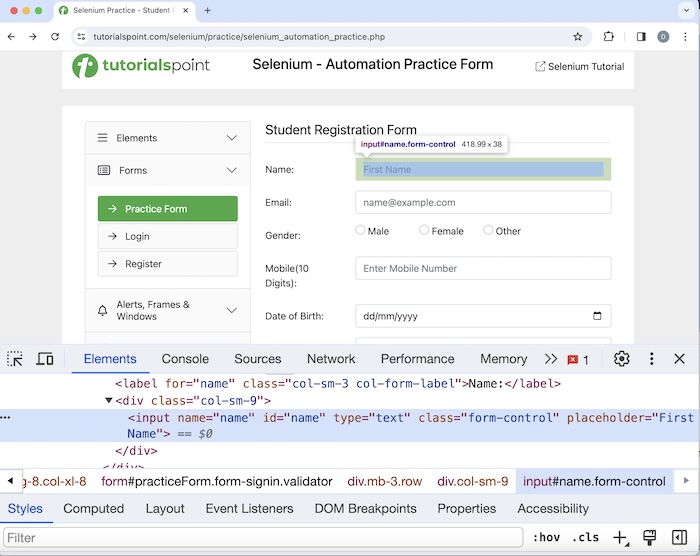
上面图片中高亮的编辑框有一个名为 name 的属性,其值为 name,CSS 表达式应为 input[name='name']。
XPath 定位器
元素的 XPath 定位器值可以用于识别该元素。在 Java 中,方法 findElement(By.xpath("<value of xpath>")) 用于定位具有 XPath 值的元素。使用此方法,可以识别具有匹配 XPath 值的第一个元素。如果不存在具有类似 XPath 值的元素,则会抛出 NoSuchElementException 异常。
语法
Webdriver driver = new ChromeDriver(); driver.findElement(By.xpath("value of xpath”));
创建 XPath 表达式的规则
要使用 XPath 定位元素,表达式应为 //tagname[@attribute='value']。XPath 可以分为两种类型:相对 XPath 和绝对 XPath。绝对 XPath 以 / 符号开头,从根节点开始到要定位的元素。
例如:
/html/body/div[1]/div[2]/div[1]/input
相对 XPath 以 // 符号开头,并且不从根节点开始。
例如:
//img[@alt='TutorialsPoint']
让我们深入了解高亮链接 - Home 的 HTML 代码,从根节点开始。
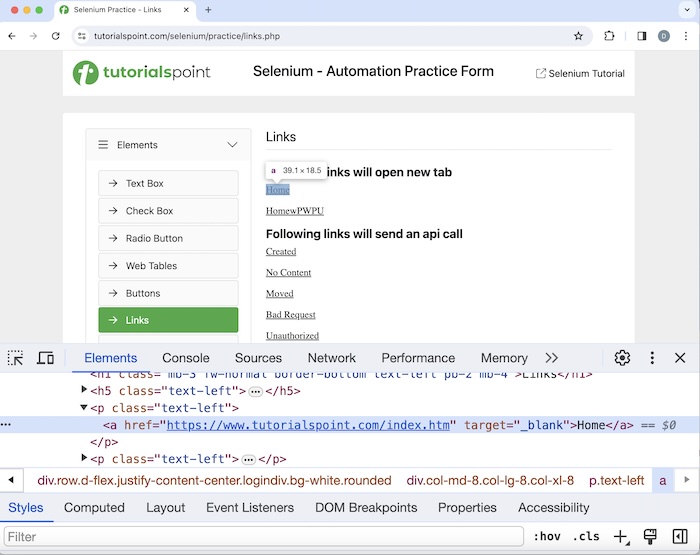
此元素的绝对 XPath 为:
/html/body/main/div/div/div[2]/p[1]/a
元素 Home 的相对 XPath 为:
//a[@href='https://tutorialspoint.com/index.htm']
XPath 函数
还有一些可用的函数可以帮助构建相对 xpath 表达式。
text()
它用于定位网页上具有可见文本的元素。Home 链接的 XPath 表达式为:
//*[text()='Home']
starts-with()
它用于识别属性值以特定文本开头的元素。此函数通常用于属性值在每次页面加载时都会发生变化的情况。
让我们研究链接 Moved 的 HTML 代码:
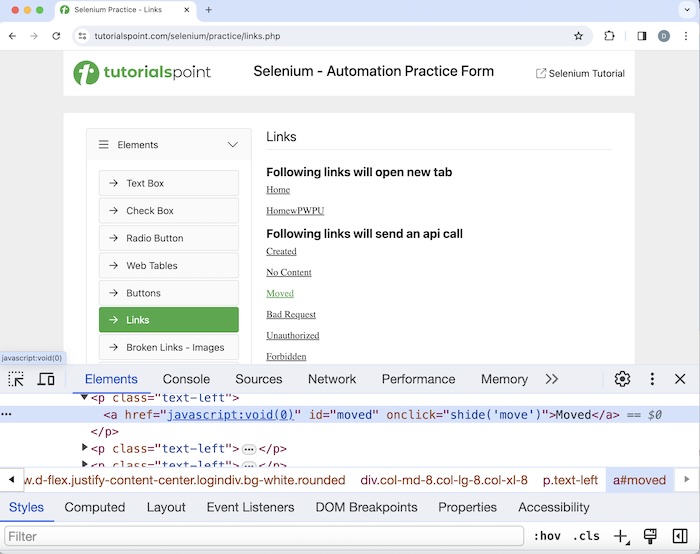
XPath 表达式为:
//a[starts-with(@id, 'mov')].
结论
本教程全面介绍了 Selenium WebDriver 定位策略。我们从描述 Id 定位器、Name 定位器、Class Name 定位器、TagName 定位器、Link Text 定位器、Partial Link Text 定位器、CSS 定位器、XPath 定位器开始,并提供了示例说明如何将它们与 Selenium 一起使用。这使您深入了解 Selenium WebDriver 定位策略。建议您不断练习所学知识,并探索与 Selenium 相关的其他知识,以加深理解并拓宽视野。