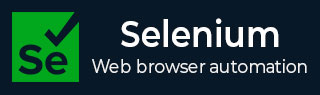
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 发出代码
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 浏览器命令
Selenium Webdriver 提供了多个命令,这些命令有助于打开浏览器,在打开的浏览器上执行某些操作,以及退出浏览器。
Selenium Webdriver 中的基本浏览器命令
下面讨论了一些浏览器命令:
get(String URL) 命令
此命令打开浏览器,然后启动作为参数传递的 URL。
语法
driver.get("https://tutorialspoint.com/selenium/practice/nestedframes.php");
getTitle() 命令
此命令获取作为字符串的焦点浏览器页面标题。它不接受任何参数。
语法
String str = driver.getTitle();
getCurrentUrl() 命令
此命令获取作为字符串的焦点浏览器 URL。它不接受任何参数。
语法
String str = driver.getCurrentUrl();
getPageSource() 命令
此命令获取作为字符串的焦点浏览器页面源代码。它不接受任何参数。
语法
String str = driver.getPageSource();
close() 命令
此命令仅关闭焦点浏览器窗口。它不接受任何参数,也不返回任何内容。
语法
driver.close();
quit() 命令
此命令关闭与驱动程序会话相关的所有内容(浏览器和所有后台驱动程序进程)。它不接受任何参数,也不返回任何内容。
语法
driver.quit();
如何在网页上检查元素?
首先右键单击网页,然后在 Chrome 浏览器中单击“检查”按钮。接下来将打开完整页面的 HTML 代码。要调查页面上的特定元素,请单击可见 HTML 代码顶部左侧的向上箭头,如下所示。
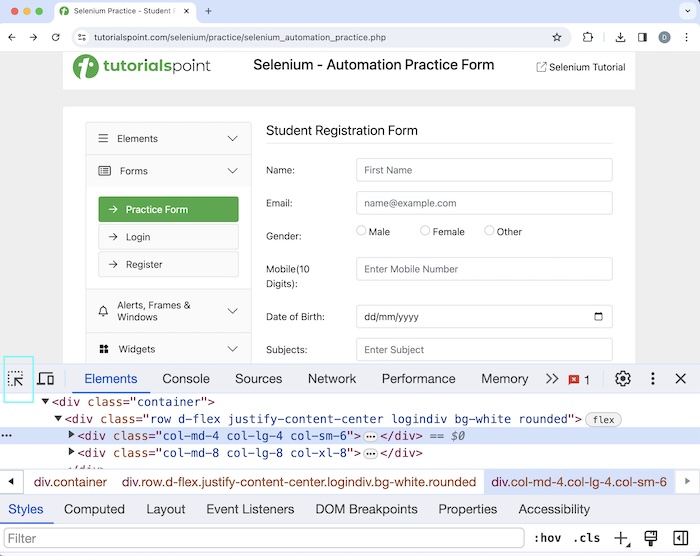
示例 1
让我们以以下页面为例,我们首先启动一个具有 URL 的应用程序:Selenium 自动化练习表单,然后获取浏览器标题Selenium Practice - 学生注册表单。
请注意,我们可以在 HTML 代码中的title标签内获取网页的浏览器标题,该标签位于head标签内。在下图中,我们看到页面的标题是Selenium Practice - 学生注册表单。
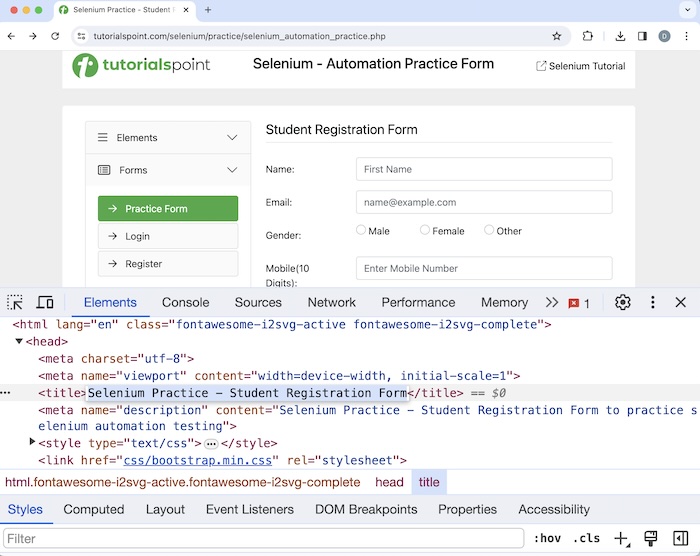
然后,我们将单击登录按钮,之后我们将导航到另一个页面,该页面的浏览器标题为Selenium Practice - 登录,URL 为:Selenium 自动化练习表单。最后,我们将退出 Web 驱动程序会话。
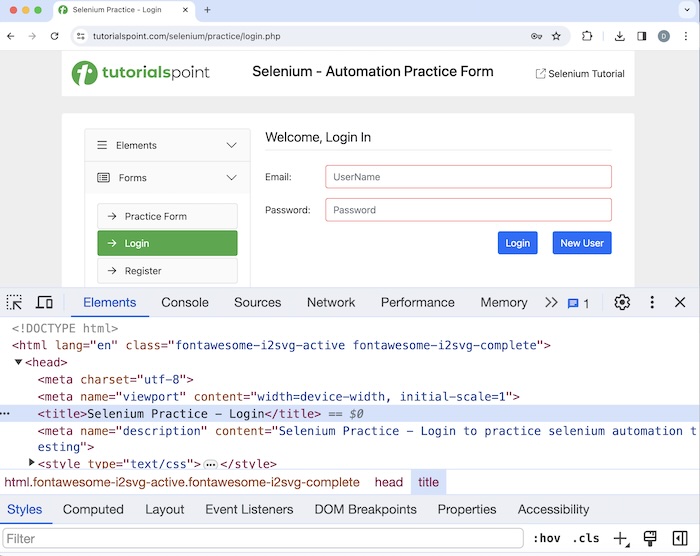
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class BrowserCommand { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Getting browser title after launch System.out.println("Getting browser title after launch: " + driver.getTitle()); // Getting browser URL after launch System.out.println("Getting URL after launch: " + driver.getCurrentUrl()); // identify link then click WebElement l = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a")); l.click(); // Getting browser title after clicking link System.out.println("Getting browser title after clicking link: " + driver.getTitle()); // Getting browser URL after launch System.out.println("Getting URL after clicking link: " + driver.getCurrentUrl()); // Quitting browser driver.quit(); } }
输出
Getting browser title after launch: Selenium Practice - Student Registration Form Getting URL after launch: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php Getting browser title after clicking link: Selenium Practice - Login Getting URL after clicking link: https://tutorialspoint.com/selenium/practice/login.php Process finished with exit code 0
在上面的示例中,我们在打开的浏览器中启动了一个 URL,并分别获取了浏览器标题和当前 URL,并在控制台中显示消息 - 启动后获取浏览器标题:Selenium Practice - 学生注册表单和启动后获取 URL:Selenium 自动化练习表单。
然后我们单击“登录”,并在导航后接收浏览器标题和当前 URL,并在控制台中显示消息 - 单击链接后获取浏览器标题:Selenium Practice - 登录和单击链接后获取 URL:Selenium 自动化练习表单。接下来,我们必须退出驱动程序会话。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 2
让我们再举一个例子,如下图所示,我们将单击新标签页。
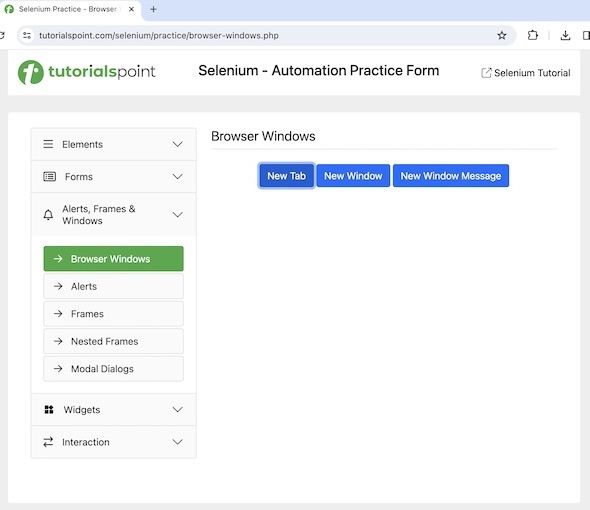
之后,我们将获得另一个包含文本新标签页的窗口。
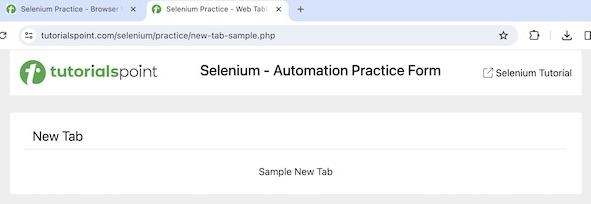
然后我们将关闭包含文本新标签页的新窗口,并返回到原始窗口并在那里访问文本 - 浏览器窗口。最后,我们将退出会话。
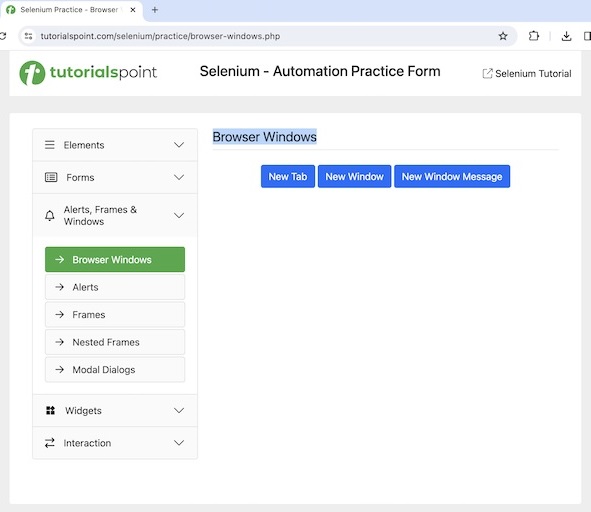
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.Set; import java.util.concurrent.TimeUnit; public class WindowClose { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will open a new window driver.get("https://tutorialspoint.com/selenium/practice/browser-windows.php"); // click button and navigate to next window WebElement b = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/button[1]")); b.click(); // Get the window handle of the original window String oW = driver.getWindowHandle(); // get all opened windows handle ids Set<String> windows = driver.getWindowHandles(); // Iterating through all window handles for (String w : windows) { if(!oW.equalsIgnoreCase(w)) { // switching to child window driver.switchTo().window(w); // accessing element in new window WebElement e = driver.findElement (By.xpath("/html/body/main/div/div/h1")); System.out.println("Text in new window is: " + e.getText()); // closing new window driver.close(); break; } } // switching to parent window driver.switchTo().window(oW); // accessing element in parent window WebElement e1 = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/h1")); System.out.println("Text in parent window is: " + e1.getText()); // quitting the browser session driver.quit(); } }
输出
Text in new window is: New Tab Text in parent window is: Browser Windows
在上面的示例中,我们捕获了新窗口上的文本,并在控制台中收到消息 - 新窗口中的文本为:新标签页。然后我们关闭了子窗口并切换回父窗口。最后,在控制台中获取父窗口上的文本 - 父窗口中的文本为:浏览器窗口。
示例 3
让我们再举一个以上页面的例子,我们将使用getPageSource()方法获取页面源代码。
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class WindowPagSource { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); // Opening the webpage where we will open a new window driver.get("https://tutorialspoint.com/selenium/practice/browser-windows.php"); // Getting browser page source System.out.println("Getting page source: " + driver.getPageSource()); // quitting the browser session driver.quit(); } }
在上面的示例中,我们捕获了在网页上启动的网页的页面源代码,并在控制台中显示消息。
结论
这结束了我们对 Selenium WebDriver 浏览器命令教程的全面介绍。我们首先描述了 Selenium Webdriver 中的基本浏览器命令,如何在网页上检查元素,并逐步介绍了如何使用 Selenium Webdriver 使用浏览器命令的示例。这使您对 Selenium WebDriver 浏览器命令有了深入的了解。明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并拓宽您的视野。