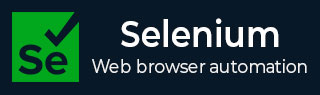
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码生成
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理Cookie
- Selenium - 日期时间选择器
- Selenium - 动态网页表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理Ajax调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 下拉框
Selenium WebDriver 可以借助 Select 类来处理网页上的下拉框。网页上可能有两种下拉框 - 单选(选择一个选项)和多选(选择多个选项)。在 HTML 术语中,每个下拉框都由名为 select 的标签名标识。此外,其每个选项都由名为 option 的标签名标识。此外,对于多选下拉框,select 标签名还有一个名为 multiple 的属性。
在 HTML 中识别下拉框
右键单击浏览器(例如 Chrome)中的网页,然后单击“检查”按钮。然后,将显示页面的所有 HTML 代码。要检查网页上的单选下拉框,请单击 HTML 代码顶部突出显示的左上方箭头,如下图所示。
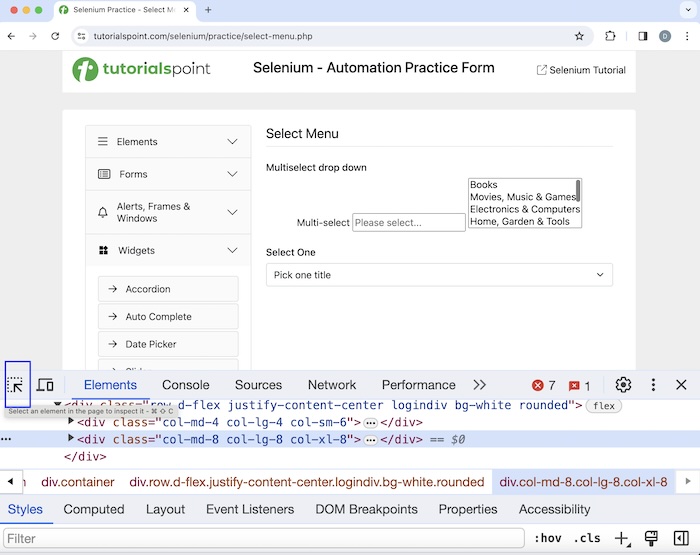
单击并将箭头指向文本选择一个旁边的下拉框后,其 HTML 代码可见,反映了 select 标签名(括在<>中),以及 option 标签名中的选项。
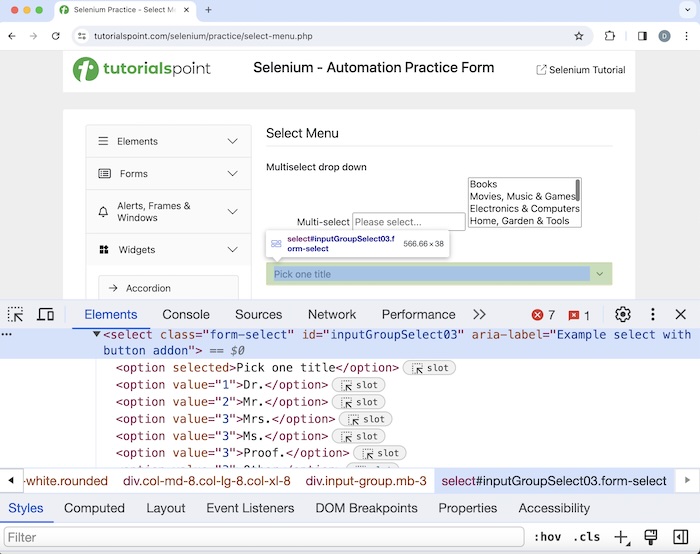
此处,选项选择一个标题具有 selected 属性,反映了它默认被选中的事实。
Selenium WebDriver 中的基本 Select 类方法
Selenium WebDriver 中有多个 Select 类方法。它们列在下面:
getOptions()
返回下拉框中所有选项的列表。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath")) Select s = new Select(e); List<WebElement> l = s.getOptions();
getFirstSelectedOption()
返回下拉框中选定的选项。如果有多个选项被选中,则只返回第一个项目。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); l = s. getFirstSelectedOption();
isMultiple()
返回布尔值,如果下拉框允许选择多个项目,则产生 true 值。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); boolean l = s.isMultiple();
selectByIndex()
下拉框要选择的选项的索引作为参数传递。索引从 0 开始。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.selectByIndex(0);
selectByValue()
下拉框要选择的选项的 value 属性作为参数传递。下拉框中的选项应具有 value 属性,以便可以使用此方法。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.selectByValue("option 1");
selectByVisibleText()
下拉框要选择的选项的可视文本作为参数传递。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.selectByVisibleText("Selenium");
deselectByVisibleText()
下拉框要取消选择的选项的可视文本作为参数传递。它仅适用于多选下拉框。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.deselectByVisibleText("Selenium");
deselectByValue()
下拉框要取消选择的选项的 value 属性作为参数传递。下拉框中的选项应具有 value 属性,以便可以使用此方法。它仅适用于多选下拉框。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.deselectByValue("option 1");
deselectByIndex()
下拉框要取消选择的选项的索引作为参数传递。索引从 0 开始。它仅适用于多选下拉框。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.deselectByIndex(0);
deselectAll()
取消选择下拉框中所有选定的选项。
语法
WebDriver driver = new ChromeDriver(); WebElement e = driver.findElement(By.xpath("value of xpath") Select s = new Select(e); s.deselectAll();
getAllSelectedOptions()
返回下拉框中所有选定的选项。如果有多个选项被选中,则返回 0 或多个选中选项的列表。对于单选下拉框,将返回 1 个选中选项的列表。如果下拉列表的某个选项具有 disabled 属性,则该选项将不会被选中。
示例 1
让我们以以下页面为例,我们将访问文本选择一个下方的下拉框,选择值Dr。
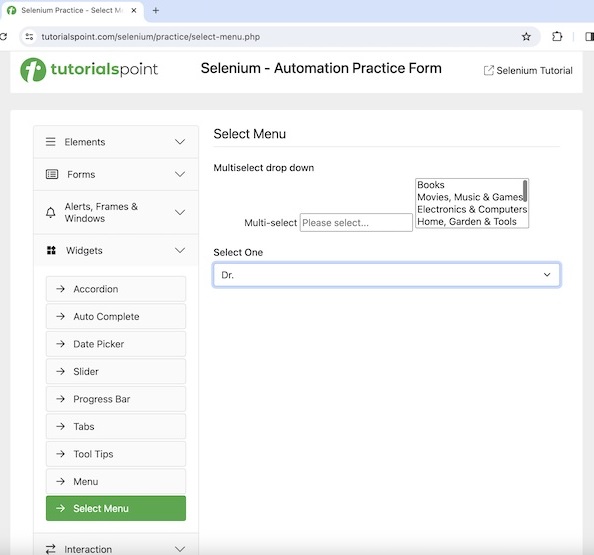
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class DropdownSingle { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://tutorialspoint.com/selenium/practice/select-menu.php"); // identify dropdown then select its options by value WebElement dropdown = driver.findElement(By.xpath("//*[@id='inputGroupSelect03']")); Select select = new Select(dropdown); // get option selected by default WebElement o = select.getFirstSelectedOption(); System.out.println("Option selected by default: " + o.getText()); // select an option by value select.selectByValue("1"); // get selected option List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Option is: " + opt.getText()); } // get all options of dropdown List<WebElement> options =select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // check if multiselect dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for checking is: "+ b); // quitting browser driver.quit(); } }
输出
Option selected by default: Pick one title Selected Option is: Dr. Options are: Pick one title Options are: Dr. Options are: Mr. Options are: Mrs. Options are: Ms. Options are: Proof. Options are: Other Boolean value for checking is: false Process finished with exit code 0
在上面的示例中,我们获得了下拉框中选定的选项,控制台中的消息为 - 选定的选项是:Dr。然后获得下拉框的所有选项,控制台中的消息为 - 选项是:选择一个标题,选项是:Dr.,选项是:Africa,选项是:Mr.,选项是:Mrs.,选项是:Ms,选项是:Proof.,和选项是:Other。
我们还验证了下拉框没有多个选择选项,控制台中的消息为 - 检查的布尔值是:false。我们还检索了下拉框中默认选择的选项,控制台中的消息为 - 默认选择的选项:选择一个标题。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 2
让我们再以以下页面为例,我们将访问文本多选下拉框旁边的多选下拉框,选择值书籍和玩具、儿童和婴儿。
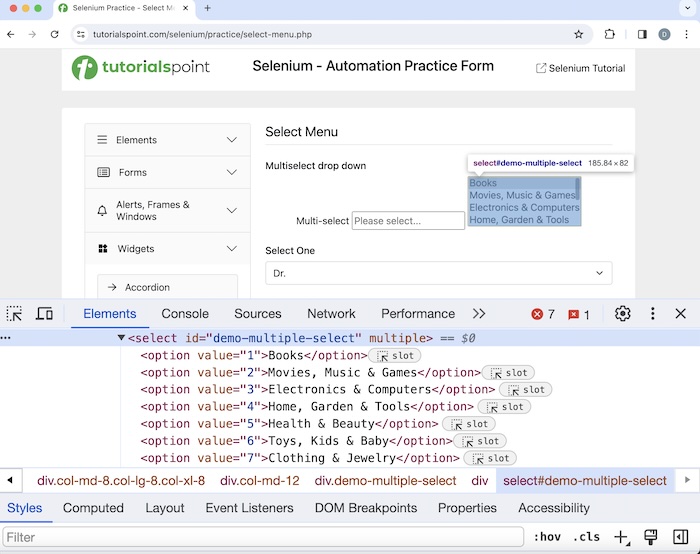
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class DropdownMultiple { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://tutorialspoint.com/selenium/practice/select-menu.php"); // identify multiple dropdown WebElement dropdown = driver.findElement(By.xpath("//*[@id='demo-multiple-select']")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by index select.selectByIndex(5); // select item by visible text select.selectByVisibleText("Books"); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(5); // get first selected option in dropdown after deselecting WebElement e = select.getFirstSelectedOption(); System.out.println("Second selected option is: "+ e.getText()); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> delectedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected: " + delectedOptions.size()); // Closing browser driver.quit(); } }
输出
Options are: Books Options are: Movies, Music & Games Options are: Electronics & Computers Options are: Home, Garden & Tools Options are: Health & Beauty Options are: Toys, Kids & Baby Options are: Clothing & Jewelry Options are: Sports & Outdoors Boolean value for multiple dropdown: true Selected Options are: Books Selected Options are: Toys, Kids & Baby First selected option is: Books Second selected option is: Books No. options selected: 0 Process finished with exit code 0
在上例中,我们在控制台中获取了下拉菜单的所有选项,显示信息为:选项包括:书籍,选项包括:电影,音乐和游戏,选项包括:家居,花园和工具,选项包括:健康和美容,选项包括:玩具,儿童和婴儿,选项包括:服装和珠宝,选项包括:运动和户外。我们还在控制台中验证了该下拉菜单允许多选,显示信息为:用于检查的布尔值为:true。
我们检索到了下拉菜单中已选择的选项,控制台显示信息为:已选择的选项包括:书籍,已选择的选项包括:玩具,儿童和婴儿。我们还获取了第一个已选择的选项,控制台显示信息为:第一个已选择的选项是:书籍。
取消选择第一个已选择的选项书籍后,我们获取了第二个已选择的选项,控制台显示信息为:第二个已选择的选项是:书籍。最后,我们取消选择了下拉菜单中所有已选择的选项,因此在控制台中获取的信息为:已选择选项数量:0。
结论
本教程对Selenium Webdriver下拉框进行了全面讲解。我们从描述HTML中下拉框的识别、Selenium Webdriver中基本的Select类方法以及使用示例开始,说明如何在Selenium Webdriver中使用它们。这将使您深入了解Selenium Webdriver下拉框。建议您多加练习所学内容,并探索其他与Selenium相关的知识,以加深理解并拓宽视野。