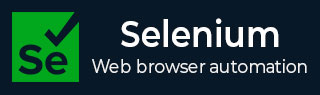
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 浏览器选项
浏览器选项指的是所有浏览器共享的功能和能力。它有助于在任何浏览器上运行自动化测试时修改浏览器的设置和功能。默认情况下,Selenium Webdriver 从一个新的浏览器配置文件开始,没有任何关于 Cookie、历史记录等的预定义设置。
向浏览器添加扩展程序
让我们举一个例子,我们将使用 **ChromeOptions** 类启动带有 Selenium IDE 扩展程序的 Chrome 浏览器。每个 Chrome 扩展程序都应该有一个 .crx 文件。在这里,我们将获取 Selenium IDE Chrome 扩展程序的 .crx 文件,并将其存储在项目中的 Resources 文件夹中。
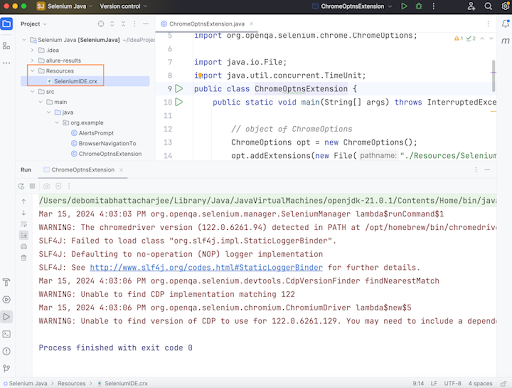
示例
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.io.File; import java.util.concurrent.TimeUnit; public class ChromeOptns { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions class ChromeOptions opt = new ChromeOptions(); // adding .crx extension opt.addExtensions(new File("./Resources/SeleniumIDE.crx")); // Initiate the Chrome Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage with Selenium IDE extension driver.get("https://tutorialspoint.com/selenium/practice/register.php"); } }
输出
Process finished with exit code 0
在这里,我们观察到 Chrome 浏览器启动了 Selenium IDE 扩展程序,并且信息栏显示 **Chrome 正在被自动化测试软件控制**。
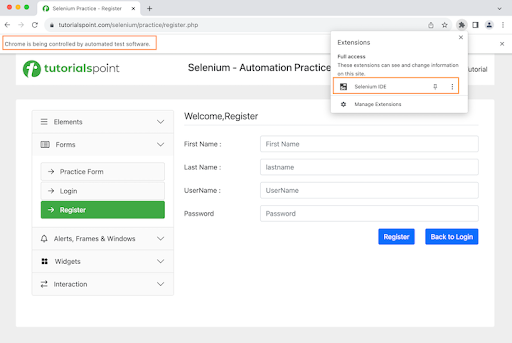
最后,我们收到消息 **进程已完成,退出代码为 0**,表示代码成功执行。
以无头模式打开浏览器
在这个例子中,我们将使用 **EdgeOptions** 类在无头浏览器中启动一个应用程序。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.edge.EdgeDriver; import org.openqa.selenium.edge.EdgeOptions; import java.io.File; import java.util.List; import java.util.concurrent.TimeUnit; public class EdgeHeadless { public static void main(String[] args) throws InterruptedException { // object of EdgeOptions FirefoxOptions opt = new EdgeOptions(); // browser in headless mode opt.addArguments("--headless=new"); // Initiate the Webdriver WebDriver driver = new EdgeDriver(opt); // adding implicit wait of 10 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage with headless mode driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // getting page title System.out.println("Getting the page title: " + driver.getTitle()); // Quit browser driver.quit(); } }
它将显示以下输出:
Getting the page title: Selenium Practice - Student Registration Form
在上面的示例中,我们观察到 Edge 浏览器以 **无头** 模式启动。我们还在控制台中获得了浏览器标题和消息 - **获取页面标题:Selenium 实践 - 学生注册表单**。
在浏览器中创建配置文件
让我们再举一个例子,我们将使用 **FirefoxOptions** 类在浏览器上拥有 Firefox 配置文件。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions; import org.openqa.selenium.firefox.FirefoxProfile; import org.openqa.selenium.firefox.ProfilesIni; import java.util.concurrent.TimeUnit; public class FirefoxProfile { public static void main(String[] args) throws InterruptedException { // object of FirefoxOptions class FirefoxOptions opt = new FirefoxOptions(); // object of ProfilesIni ProfilesIni prof = new ProfilesIni(); // get profile FirefoxProfile p = prof.getProfile("<profileName>"); opt.setProfile(p); // Initiate the Webdriver WebDriver driver = new FirefoxDriver(opt); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // obtain page title System.out.println("Obtain the page title: " + driver.getTitle()); // Quit browser driver.quit(); } }
它将显示以下输出:
Obtain the page title: Selenium Practice - Student Registration Form
在这里,Firefox 浏览器使用创建的配置文件打开。我们还在控制台中获得了浏览器标题和消息 - **获取页面标题:Selenium 实践 - 学生注册表单**。
处理 Edge 中的 SSL 证书错误
为了克服 Edge 浏览器中的 SSL 证书错误,我们将使用 EdgeOptions 和 DesiredCapabilities 类。
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.EdgeDriver; import org.openqa.selenium.firefox.EdgeOptions; import org.openqa.selenium.remote.CapabilityType; import org.openqa.selenium.remote.DesiredCapabilities; import java.util.concurrent.TimeUnit; public class SSLEdge { public static void main(String[] args) throws InterruptedException { // object of Desired Capabilities class DesiredCapabilities dc = new DesiredCapabilities(); dc.setCapability(CapabilityType.ACCEPT_INSECURE_CERTS, true); // object Edge Options class EdgeOptionsOptions opt = new EdgeOptions(); opt.merge(dc); // Initiate the Webdriver along with options WebDriver driver = new EdgeDriver(opt); // adding implicit wait of 5 secs driver.manage().timeouts().implicitlyWait(55, TimeUnit.SECONDS); // launch application with SSL certificate error driver.get("https://expired.badssl.com"); // get browser title System.out.println("Get Browser title in Edge: " + driver.getTitle()); // quit the browser driver.quit(); } }
它将显示以下输出:
Get Browser title in Edge: Privacy error
在这里,我们摆脱了 Edge 中的 SSL 证书错误并启动了应用程序。然后在控制台中获得了浏览器标题和消息 - **Edge 中的浏览器标题:隐私错误**。
页面加载策略
浏览器中有多种页面加载策略可用,分别是 NORMAL(浏览器中的默认页面加载设置)、EAGER 和 NONE。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; public class ChromePageLoadNormal { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // setting pageloadStrategy to normal opt.setPageLoadStrategy(PageLoadStrategy.NORMAL); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/register.php"); // quit browser driver.quit(); } }
它将显示以下输出:
Process finished with exit code 0
在上面的示例中,我们设置了浏览器选项,使页面加载策略设置为 NORMAL。如果需要将页面加载策略设置为 EAGER,则需要进行以下代码更改:
// object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // setting pageloadStrategy to EAGER opt.setPageLoadStrategy(PageLoadStrategy.EAGER); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt);
如果需要将页面加载策略设置为 NONE,则需要进行以下代码更改:
// object of ChromeOptions ChromeOptions opt = new ChromeOptions(); // setting pageloadStrategy to NONE opt.setPageLoadStrategy(PageLoadStrategy.NONE); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt);
结论
本教程全面介绍了 Selenium Webdriver 浏览器选项。我们已经通过示例演示了如何向浏览器添加配置文件和扩展程序,如何在无头模式下启动浏览器,如何处理 SSL 证书错误以及如何使用浏览器选项和 Selenium Webdriver 进行页面加载策略。这使您能够深入了解 Selenium Webdriver 中的浏览器选项。明智的做法是不断练习您所学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并拓宽您的视野。