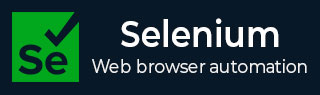
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 基于关键字的框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Kotlin Selenium 教程
Selenium 可以与多种语言一起使用,例如 Java、Python、Kotlin、JavaScript、Ruby 等。Selenium 广泛用于 Web 自动化测试。Selenium 是一款开源且可移植的自动化软件测试工具,用于测试 Web 应用程序。它能够跨不同浏览器和操作系统运行。Selenium 不仅仅是一个工具,而是一套工具,可以帮助测试人员更有效地自动化基于 Web 的应用程序。
使用 Kotlin 设置 Selenium
步骤 1 - 使用以下链接在我们的系统中安装 Maven:https://maven.apache.org/download.cgi。
要更详细地了解如何设置 Maven,请参阅以下链接:Maven 环境。
通过运行以下命令确认已安装的Maven版本:
mvn –version
它将显示以下输出:
Apache Maven 3.9.6 (bc0240f3c744dd6b6ec2920b3cd08dcc295161ae) Maven home: /opt/homebrew/Cellar/maven/3.9.6/libexec Java version: 21.0.1, vendor: Homebrew, runtime: /opt/homebrew/Cellar/openjdk/21.0.1/libexec/openjdk.jdk/Contents/Home Default locale: en_IN, platform encoding: UTF-8 OS name: "mac os x", version: "14.0", arch: "aarch64", family: "mac"
执行的命令的输出表明系统中安装的 Maven 版本为 Apache Maven 3.9.6。
步骤 2 - 从以下链接下载并安装代码编辑器 IntelliJ,以编写和运行 Selenium 测试:https://www.jetbrains.com/idea/。
要更详细地了解如何设置 IntelliJ,请参阅以下链接:Selenium IntelliJ。
步骤 3 - 启动 IntelliJ 并单击“新建项目”按钮。

步骤 4 - 输入项目名称、位置,然后选择 Kotlin 作为语言,Maven 作为构建系统。

步骤 5 - 从“构建”菜单构建项目。

步骤 6 - 从以下链接添加 Selenium Maven 依赖项:https://mvnrepository.com/artifact/。
步骤 7 - 保存包含所有依赖项的 pom.xml 并更新 maven 项目。
步骤 8 - 在 Maven 项目 SeleniumKotlin 中,右键单击 test 文件夹中的 kotlin 文件夹,然后创建一个包,例如 TestCases。
步骤 9 - 右键单击 TestCases 包,然后选择“新建”菜单,然后单击“Kotlin 类/文件”菜单。

步骤 10 - 在“新建 Kotlin 类/文件”字段中输入文件名,例如 MyTest,然后按 Enter 键。

步骤 11 - 在 MyTest.kt 文件中添加以下代码。
package TestCases import org.openqa.selenium.WebDriver import org.openqa.selenium.edge.EdgeDriver import java.time.Duration import org.testng.annotations.Test class MyTest { @Test fun launchBrow() { // Initiate Webdriver val driver: WebDriver = EdgeDriver(); // adding implicit wait of 15 seconds driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(15)); // URL launch driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // get browser title after browser launch System.out.println("Browser title: " + driver.title); } }
在 pom.xml 文件中添加的整体依赖项:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumKotlin</artifactId> <version>1.0-SNAPSHOT</version> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <kotlin.code.style>official</kotlin.code.style> <kotlin.compiler.jvmTarget>1.8</kotlin.compiler.jvmTarget> </properties> <repositories> <repository> <id>mavenCentral</id> <url>https://repo1.maven.org/maven2/</url> </repository> </repositories> <build> <sourceDirectory>src/main/kotlin</sourceDirectory> <testSourceDirectory>src/test/kotlin</testSourceDirectory> <plugins> <plugin> <groupId>org.jetbrains.kotlin</groupId> <artifactId>kotlin-maven-plugin</artifactId> <version>1.9.23</version> <executions> <execution> <id>compile</id> <phase>compile</phase> <goals> <goal>compile</goal> </goals> </execution> <execution> <id>test-compile</id> <phase>test-compile</phase> <goals> <goal>test-compile</goal> </goals> </execution> </executions> </plugin> <plugin> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.2</version> </plugin> <plugin> <artifactId>maven-failsafe-plugin</artifactId> <version>2.22.2</version> </plugin> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>exec-maven-plugin</artifactId> <version>1.6.0</version> <configuration> <mainClass>MainKt</mainClass> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>org.jetbrains.kotlin</groupId> <artifactId>kotlin-test-junit5</artifactId> <version>1.9.23</version> <scope>test</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.19.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.testng/testng --> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>5.10.0</version> <scope>test</scope> </dependency> <dependency> <groupId>org.jetbrains.kotlin</groupId> <artifactId>kotlin-stdlib</artifactId> <version>1.9.23</version> </dependency> </dependencies> </project>
在此示例中遵循的项目结构:

步骤 12 - 右键单击并选择“运行‘MyRun’”选项。等待运行完成。
它将显示以下输出:
Browser title: Selenium Practice - Student Registration Form =============================================== Default Suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
在上面的示例中,我们首先启动了 Edge 浏览器并启动了一个应用程序,然后检索了浏览器标题,并在控制台中收到了消息 - 浏览器标题:Selenium 实践 - 学生注册表单。
控制台中的结果显示已运行的总测试数:1,因为只有一个方法带有 @Test 注释 - launchBrow()。
最后,收到了消息通过:1和进程已完成,退出代码为 0,表明代码已成功执行。
使用 Selenium Kotlin 识别元素并检查其功能
导航到网页后,我们必须与页面上可用的 Web 元素进行交互,例如单击链接/按钮、在编辑框中输入文本等,以完成我们的自动化测试用例。
为此,我们的首要任务应该是识别元素。我们可以使用链接的链接文本进行识别,并使用方法 findElement(By.linkText("<链接文本的值>"))。这样,应该返回具有匹配链接文本值的第一个元素。
如果找不到具有匹配链接文本值的元素,则应抛出 NoSuchElementException。
让我们看看上面图片中讨论的链接的 html 代码:

<a href="javascript:void(0)" id="moved" onclick="shide('move')">Moved</a>
上面图片中突出显示的链接 - 已移动 的链接文本值为 Moved。让我们在识别它之后单击它。单击“已移动”链接后,文本 - 链接已返回状态 301 和状态文本“永久移动” 将出现在网页上。最后,我们将退出浏览器。

示例
package TestCases import org.openqa.selenium.By import org.openqa.selenium.WebDriver import org.openqa.selenium.edge.EdgeDriver import java.time.Duration import org.testng.annotations.Test class MyTest { @Test fun accessLnkGetText() { // Initiate Webdriver val driver: WebDriver = EdgeDriver(); // adding implicit wait of 15 seconds driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(15)); // URL launch driver.get("https://tutorialspoint.com/selenium/practice/links.php"); // identify link then click val lnk = driver.findElement(By.linkText("Moved")); lnk.click(); // identify text the get text val txt = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[3]")); System.out.println("Text Obtained is: " + txt.text); // quit browser driver.quit() } }
它将显示以下输出:
Text Obtained is: Link has responded with status 301 and status text Moved Permanently =============================================== Default Suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
在上面的示例中,我们首先启动了 Edge 浏览器并启动了一个应用程序,然后单击了该链接。单击链接后,我们获得了文本,并在控制台中收到了消息 - 获得的文本是:链接已返回状态 301 和状态文本“永久移动”。
控制台中的结果显示已运行的总测试数:1,因为只有一个方法带有 @Test 注释 - accessLnkGetText()。
最后,收到了消息通过:1和进程已完成,退出代码为 0,表明代码已成功执行。
这总结了我们对 Selenium - Kotlin 教程的全面讲解。我们首先介绍了如何使用 Kotlin 设置 Selenium,如何使用 Selenium Kotlin 启动浏览器和退出会话,以及如何识别元素并使用 Selenium Kotlin 检查其功能。
这使您掌握了 Selenium - Kotlin 教程的深入知识。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。