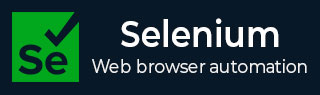
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 手写笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - Safari 选项
Selenium Webdriver 可用于在 Safari 浏览器上运行自动化测试。某些功能和特性仅适用于 Apple 用户的 Safari。Safari 是一款流行的浏览器,由 Apple 设备默认提供。对于 Safari 浏览器版本 10 及更高版本,safaridriver 会自动安装,无需单独安装。
safari 驱动程序默认安装在操作系统上,而 Firefox 和 Chromium 浏览器则不是这样。我们需要进行一些初始设置,在 Safari 浏览器中启用“开发”菜单,然后才能在其上实际运行测试。默认情况下,“开发”菜单不可见。
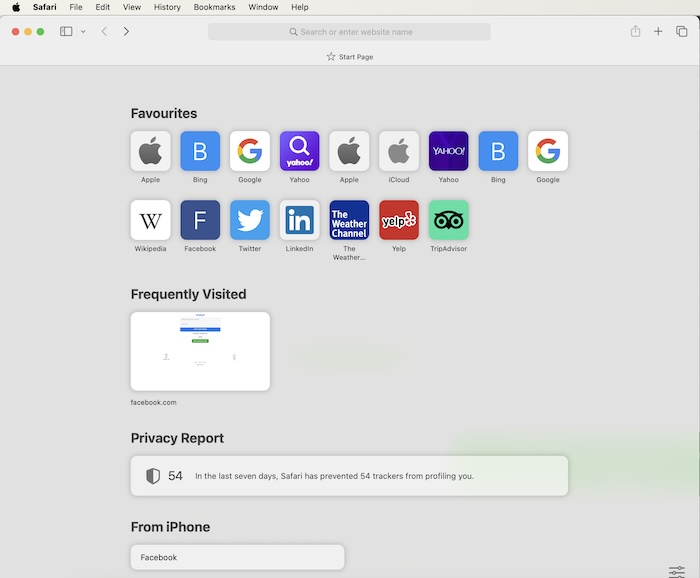
为此,我们需要打开 Safari,然后单击“设置”。接下来,我们需要转到“高级”选项卡,并选中“显示 Web 开发人员功能”选项。
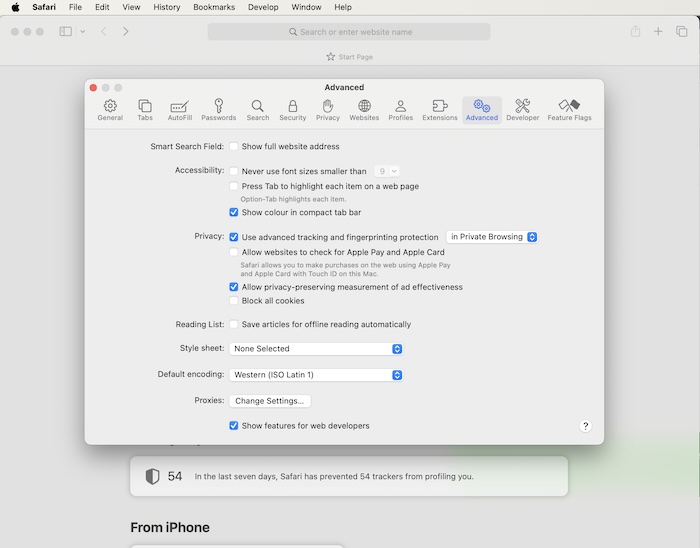
要开始在 Safari 浏览器上运行自动化测试,我们需要从终端运行命令:
safaridriver --enable
示例
让我们以以下页面为例,我们在此启动一个 Safari 驱动程序会话以及 SafariOptions。然后,我们将获取浏览器标题“Selenium Practice - Student Registration Form”和当前 URL Selenium Automation Practice Form。
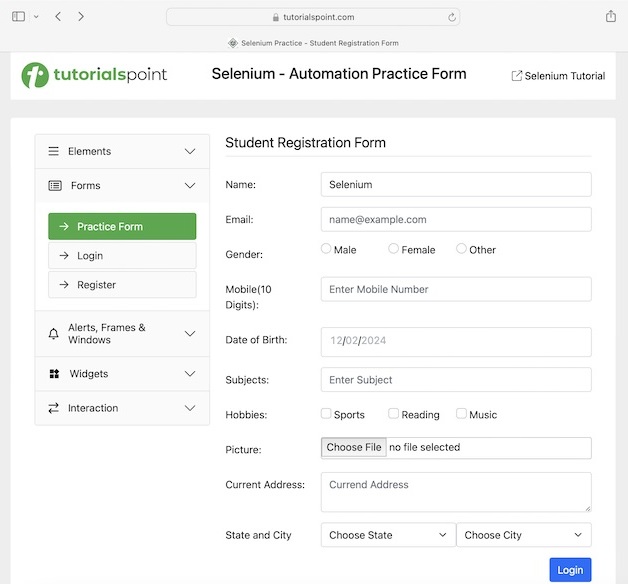
要在 SafariDriver 以及 SafariOptions 类一起使用,我们需要添加导入语句:
import org.openqa.selenium.safari.SafariOptions import org.openqa.selenium.safari.SafariDriver
代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.safari.SafariDriver; import org.openqa.selenium.safari.SafariOptions; import java.util.concurrent.TimeUnit; public class SafariOpts { public static void main(String[] args) throws InterruptedException { // Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // get the page title System.out.println("Browser title is: " + driver.getTitle()); // get the current URL System.out.println("Current URL is: " + driver.getCurrentUrl()); // closing the browser driver.quit(); } }
输出
Browser title is: Selenium Practice - Student Registration Form Current URL is: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php Process finished with exit code 0
在上面的示例中,我们使用 SafariOptions 启动了 Safari 浏览器,然后分别获取了浏览器标题和当前 URL,并在控制台中显示消息 - “浏览器标题为:Selenium Practice - Student Registration Form 和当前 URL 为:” Selenium Automation Practice Form。
Safari 浏览器始终将日志(如果已启用)存储在以下位置 - ~/Library/Logs/com.apple.WebDriver/。与 Chrome、Firefox 等其他浏览器不同,我们无法选择将日志及其级别分别保存到其他位置和值。
示例 - 捕获 Safari 日志
让我们以一个启用 Safari 日志的示例为例。
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.safari.SafariDriver; import org.openqa.selenium.safari.SafariDriverService; import org.openqa.selenium.safari.SafariOptions; import java.util.concurrent.TimeUnit; public class SafariOptsLogs { public static void main(String[] args) throws InterruptedException { // Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // enabling the Safari logs SafariDriverService service = new SafariDriverService.Builder().withLogging(true).build(); // get the page title System.out.println("Logging enabled: " + service); // close the browser driver.quit(); } }
输出
Logging enabled: org.openqa.selenium.safari.SafariDriverService@3eeb318f
示例 - 禁用 Safari 日志
让我们以一个禁用 Safari 日志的示例为例。
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.safari.SafariDriver; import org.openqa.selenium.safari.SafariDriverService; import org.openqa.selenium.safari.SafariOptions; import java.util.concurrent.TimeUnit; public class SafariOptsLogsFalse { public static void main(String[] args) throws InterruptedException { // Using the Safari options SafariOptions opts = new SafariOptions(); WebDriver driver = new SafariDriver(opts); // adding an implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // opening the Safari browser and launch a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // disabling the Safari Logs SafariDriverService service = new SafariDriverService.Builder() .withLogging(false) .build(); // get the page title System.out.println("Launched Browser title is: " + driver.getTitle()); // get the current URL System.out.println("Current URL is: " + driver.getCurrentUrl()); // close the browser driver.quit(); } }
输出
Browser title is: Selenium Practice - Tool Tips Current URL is: https://tutorialspoint.com/selenium/practice/tool-tips.php
结论
本教程全面介绍了 Selenium WebDriver Safari 选项,到此结束。我们从描述 SafariOptions 类开始,并逐步讲解了如何将 SafariOptions 与 Selenium Webdriver 一起使用的示例。这使您深入了解了 Selenium Webdriver 中的 SafariOptions 类。明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。