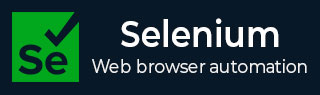
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码生成
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 等待支持
Selenium Webdriver 可以与各种等待机制一起使用,例如显式、隐式和流畅等待,以实现同步并提供等待支持。等待主要应用于测试中,以处理当 Web 元素在 Selenium 测试预期它在页面或 DOM 中可用时不可用的情况。
整个页面加载完毕之前,通常会存在一些延迟时间,并且 Web 元素在网页上完全可用。Selenium Webdriver 中提供的等待机制有助于在元素以正确的状态出现在网页上/消失之前,阻止测试执行。
Selenium Webdriver 中可用的基本等待
Selenium Webdriver 中有多种等待机制可用。它们列在下面:
隐式等待
这是 Selenium 中可用的默认等待。它是一种适用于整个驱动程序会话的全局等待。默认等待时间为 0,这意味着如果找不到元素,则会立即抛出错误。
显式等待
它类似于添加到代码中的循环,该循环轮询网页以使特定场景在退出循环并移动到下一行代码之前变为 true。
流畅等待
这是驱动程序等待特定元素条件为 true 的最长时间。它还确定驱动程序在找到元素或抛出异常之前将进行验证的间隔(轮询间隔)。流畅等待是一种自定义显式等待,它提供选项以自动处理特定异常以及在发生异常时使用自定义消息。FluentWait 类用于向测试添加流畅等待。
语法
Wait wt = new FluentWait(driver) .withTimeout(20, TimeUnit.SECONDS) .pollingEvery(5, TimeUnit.SECONDS) .ignoring(ElementNotInteractableException.class); wt.until(ExpectedConditions.titleIs("Tutorialspoint"));
在上面的示例中,指定了超时和轮询间隔,这意味着驱动程序将等待 20 秒,并在超时时间内以 5 秒的间隔进行轮询,以满足Tutorialspoint浏览器标题条件。如果在该时间范围内未满足该条件,则将抛出异常,否则将执行下一步。
示例 1 - 显式等待
让我们以下面的图像为例,我们首先单击单击我按钮。
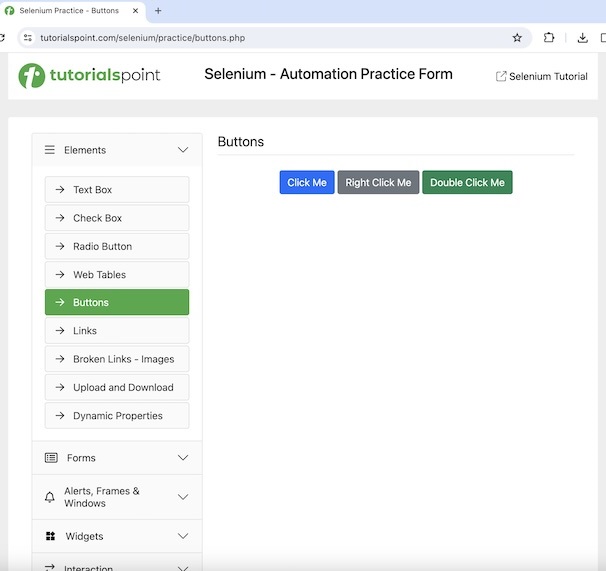
单击“单击我”后,我们将使用显式等待,并等待文本您已完成动态单击出现在网页上。
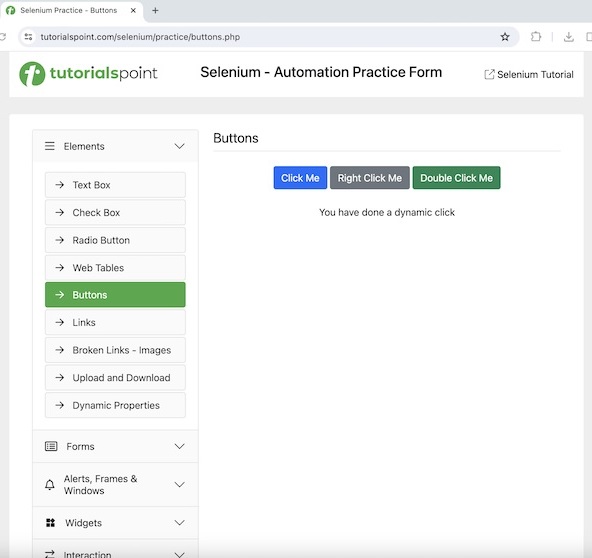
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import java.time.Duration; import java.util.concurrent.TimeUnit; public class ExplicitsWait { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/buttons.php"); // identify button then click on it WebElement l = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/button[1]")); l.click(); // Identify text WebElement e = driver.findElement(By.xpath("//*[@id='welcomeDiv']")); // explicit wait to expected condition for presence of a text WebDriverWait wt = new WebDriverWait(driver, Duration.ofSeconds(2)); wt.until(ExpectedConditions.presenceOfElementLocated(By.xpath("//*[@id='welcomeDiv']"))); // get text System.out.println("Get text after clicking: " + e.getText()); // Quitting browser driver.quit(); } }
输出
Get text after clicking: You have done a dynamic click Process finished with exit code 0
在上面的示例中,单击单击我按钮后获得的文本为您已完成动态单击。
示例 2 - 流畅等待
让我们再举一个下面的页面的例子,我们首先点击颜色更改按钮。
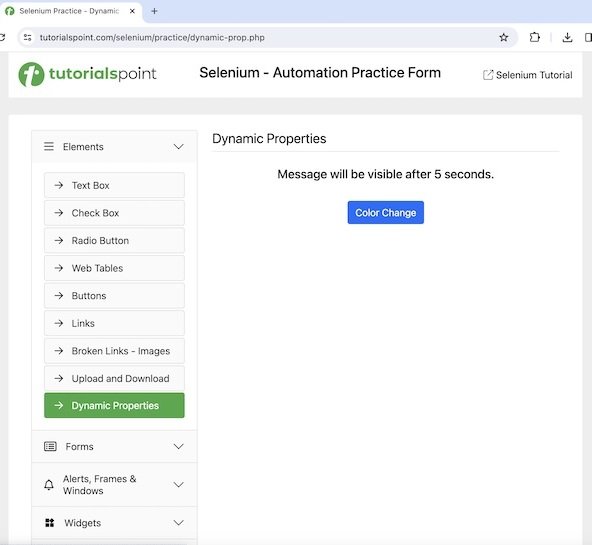
单击颜色更改后,我们将使用流畅等待,并等待按钮5 秒后可见出现在网页上。
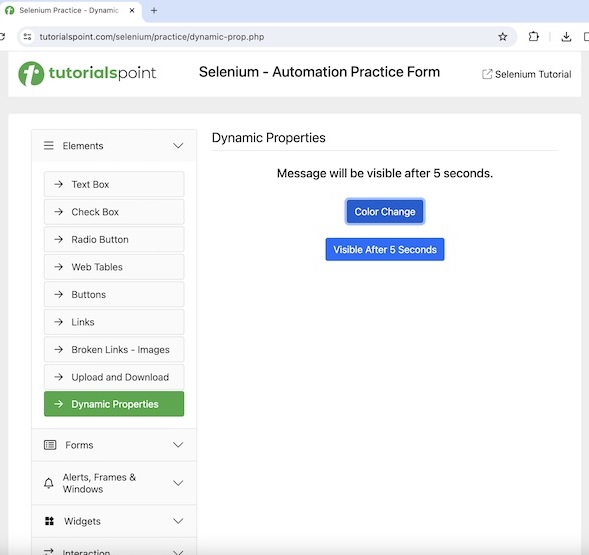
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.FluentWait; import org.openqa.selenium.support.ui.Wait; import java.time.Duration; public class Fluentwts { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/dynamic-prop.php"); // identify button then click WebElement l = driver.findElement(By.xpath("//*[@id='colorChange']")); l.click(); // fluent wait of 6 secs till other button appears Wait<WebDriver> w = new FluentWait<WebDriver>(driver) .withTimeout(Duration.ofSeconds(20)) .pollingEvery(Duration.ofSeconds(6)) .ignoring(NoSuchElementException.class); WebElement m = w.until(ExpectedConditions.visibilityOfElementLocated (By.xpath("//*[@id='visibleAfter']"))); // checking button presence System.out.println("Button appeared: " + m.isDisplayed()); // Quitting browser driver.quit(); } }
输出
Button appeared: true Process finished with exit code 0
在上面的示例中,我们观察到单击颜色更改按钮后获得的按钮为5 秒后可见。
结论
本教程全面介绍了 Selenium Webdriver 等待支持。我们首先介绍了 Selenium Webdriver 中可用的基本等待,并通过示例说明了 Selenium Webdriver 中的显式和流畅等待。这使您能够深入了解 Selenium Webdriver 等待支持。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽视野。