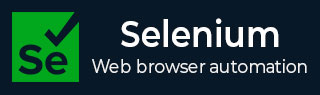
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码生成
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态网页表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览器上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 动态网页表格
Selenium WebDriver 可用于处理动态网页表格(行数和列数不相等的表格)。每个表格在 HTML 中都由标签名 table 标识。此外,表格中的每一行都具有标签名为 tr,列的标签名为 td。列标题由 th 标签名标识。
在网页上识别动态网页表格
右键单击在浏览器(例如 Chrome)中打开的网页,然后单击“检查”按钮。这将显示整个页面的完整 HTML 代码。要检查下面页面上的网页表格,请单击下面突出显示的左上方箭头。

单击箭头并将其指向表格(如下图所示)后,其 HTML 代码将可用,反映表格标签名以及表格行、列和标题的 tr、td 和 th 标签名。

thead 标签下的 th 标签名用于表格中的列标题。
<thead> <tr> <th scope="col">First Name</th> <th scope="col">Last Name</th> <th scope="col">Age</th> <th scope="col">Email</th> <th scope="col">Salary</th> <th scope="col">Department</th> <th scope="col">Action</th> </tr> </thead>

tbody 标签下的 td 标签名用于表格中的单元格值。

示例 1
让我们以上面表格为例,我们将使用该表格的定位器 xpath 获取具有 td 标签名的表格的所有单元格值。
语法
Webdriver driver = new ChromeDriver(); // Locate the table element WebElement table1 = driver.findElement(By.xpath("value of xpath")); // Find all rows in the table then store in list List<WebElement> r = table1.findElements(By.xpath(".//tr")); // Looping through rows and get cell values for (WebElement rw : r) { List<WebElement> cell = rw.findElements(By.xpath(".//td")); for (WebElement c : cell) { String value = c.getText(); } }
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; public class HandlingWebTable { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Open the webpage to identify table driver.get("https://tutorialspoint.com/selenium/practice/webtables.php"); // Locate the table element WebElement table1 = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/div[2]/table")); // Find all rows in the table List<WebElement> r = table1.findElements(By.xpath(".//tr")); // Looping through rows and get cell values for (WebElement rw : r) { List<WebElement> cell = rw.findElements(By.xpath(".//td")); for (WebElement c : cell) { String value = c.getText(); System.out.println("Cells values: " + value); } } // Closing browser driver.quit(); } }
输出
Cells values: Cierra Cells values: Vega Cells values: 39 Cells values: cierra@example.com Cells values: 10000 Cells values: Insurance Cells values: Cells values: Alden Cells values: Cantrell Cells values: 45 Cells values: alden@example.com Cells values: 12000 Cells values: Compliance Cells values: Cells values: Kierra Cells values: Gentry Cells values: 29 Cells values: kierra@example.com Cells values: 2000 Cells values: Legal Cells values: Cells values: Alden Cells values: Cantrell Cells values: 45 Cells values: alden@example.com Cells values: 12000 Cells values: Compliance Cells values: Cells values: Kierra Cells values: Gentry Cells values: 29 Cells values: kierra@example.com Cells values: 2000 Cells values: Legal Cells values: Process finished with exit code 0
在上面的示例中,我们捕获了表格中的所有单元格值。
最后,收到消息Process finished with exit code 0,表示代码成功执行。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 2
让我们以同一个表格为例,我们将使用该表格的定位器 xpath 获取具有 th 标签名的表格的所有单元格标题。
语法
获取表格中所有单元格标题的语法。
Webdriver driver = new ChromeDriver(); WebElement table1 = driver.findElement(By.xpath("value of xpath")); List<WebElement> r = table1.findElements(By.xpath(".//tr")); // Looping through rows and get cell values for (WebElement rw : r) { List<WebElement> cell = rw.findElements(By.xpath(".//th")); for (WebElement c : cell) { String value = c.getText(); } }
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.List; import java.util.concurrent.TimeUnit; public class HandlingWebTable { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Open the webpage driver.get("https://tutorialspoint.com/selenium/practice/webtables.php"); // Locate the table element WebElement table1 = driver..findElement (By.xpath ("/html/body/main/div/div/div[2]/form/div[2]/table")); // Find all rows in the table List<WebElement> r = table1.findElements(By.xpath(".//tr")); // Looping through rows and get headers for (WebElement rw : r) { List<WebElement> cell = rw.findElements(By.xpath(".//th")); for (WebElement c : cell) { String value = c.getText(); System.out.println("Table headers: " + value); } } // Closing browser driver.quit(); } }
输出
Table headers: First Name Table headers: Last Name Table headers: Age Table headers: Email Table headers: Salary Table headers: Department Table headers: Action Process finished with exit code 0
在上面的示例中,我们捕获了表格中的所有表头。
结论
本教程总结了 Selenium WebDriver 动态网页表格的全面介绍。我们从描述如何在 HTML 中识别动态网页表格开始,并通过示例说明如何在 Selenium WebDriver 中处理动态网页表格。这使您能够深入了解 Selenium WebDriver 动态网页表格。最好继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽视野。