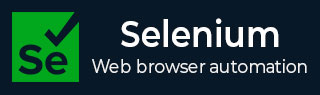
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 复制和粘贴
在使用 Selenium WebDriver 开发的测试脚本中,可以自动化复制和粘贴操作。在测试应用程序时,我们经常会从一个编辑框中复制文本,然后将其粘贴到另一个编辑框中。
可以使用 Selenium 中的Keys类执行复制和粘贴操作。用于复制和粘贴的键可以使用Ctrl + C和Ctrl + V分别实现。要按下的这些键作为参数发送到sendKeys()方法。
现在让我们讨论一下在网页上执行复制和粘贴操作的元素的识别,如下图所示。首先,我们需要右键单击网页,然后在 Chrome 浏览器中单击“检查”按钮。然后,整个页面的相应 HTML 代码将可见。为了检查该网页上的源元素和目标元素,我们需要单击可见 HTML 代码顶部的左上箭头,如下所示。
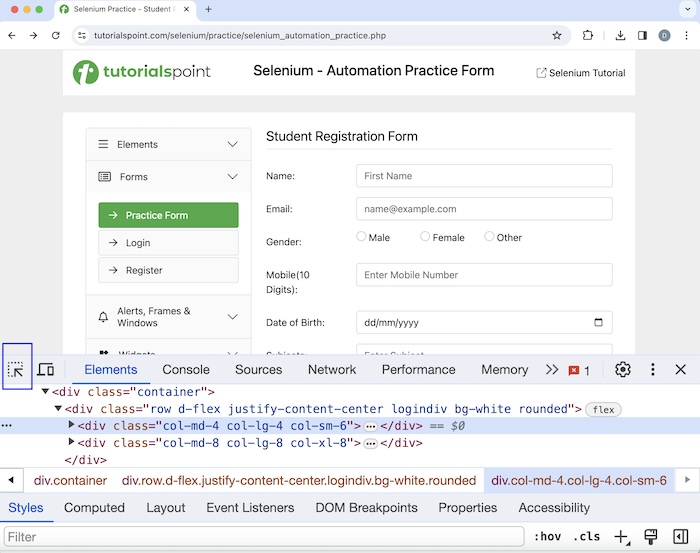
让我们以以下页面为例,我们首先在第一个输入框(突出显示)中输入文本 -Selenium(在姓名旁边),然后将相同的文本复制并粘贴到另一个输入框(突出显示)中(在电子邮件旁边)。
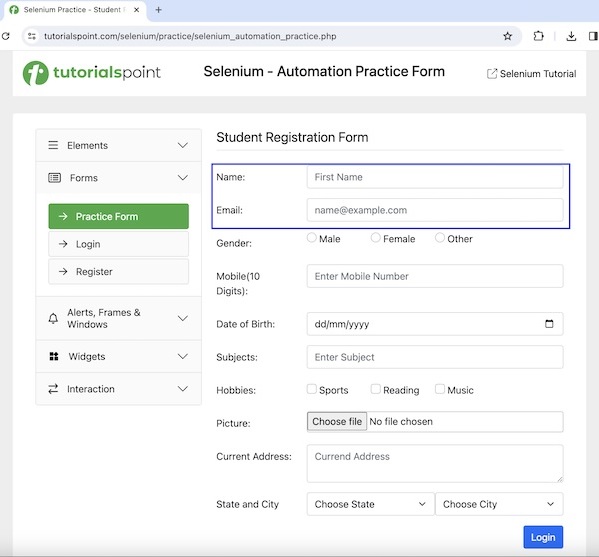
语法
如果您使用的是 MAC 机器,则语法为:
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Selenium"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // copy text from first input box then paste to second input box e.sendKeys(Keys.COMMAND, "a"); e.sendKeys(Keys.COMMAND, "c"); // paste to second input box s.sendKeys(Keys.COMMAND, "v");
如果您使用的是 Windows 机器,则语法为:
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Selenium"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // copy text from first input box then paste to second input box e.sendKeys(Keys.CONTROL, "a"); e.sendKeys(Keys.CONTROL, "c"); // paste to second input box s.sendKeys(Keys.CONTROL, "v");
示例
在 CopyAndPaste.java 类文件中实现代码。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CopyAndPaste { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter some text e.sendKeys("Selenium"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='email']")); // copy text from first input box then paste to second input box e.sendKeys(Keys.COMMAND, "a"); e.sendKeys(Keys.COMMAND, "c"); // paste to second input box s.sendKeys(Keys.COMMAND, "v"); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
输出
Value copied and pasted: Selenium Process finished with exit code 0
在上面的示例中,我们首先在第一个输入框中输入了文本Selenium,然后将文本复制并粘贴到第二个输入框中,并在控制台中获得了输入文本作为消息 -已复制并粘贴的值:Selenium。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
让我们再举一个例子,我们再次首先在一个输入框中输入文本,然后使用Actions类的keyUp()、keyDown()和sendKeys()方法将相同的文本复制并粘贴到另一个输入框中。所有这些方法都将要按下的键作为参数。
语法
如果您使用的是 MAC 机器,则语法为:
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Selenium"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // Actions class methods to select text Actions a = new Actions(driver); a.keyDown(Keys.COMMAND); a.sendKeys("a"); a.keyUp(Keys.COMMAND); a.build().perform(); // Actions class methods to copy text a.keyDown(Keys.COMMAND); a.sendKeys("c"); a.keyUp(Keys.COMMAND); a.build().perform(); // Action class methods to tab and reach to the next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(Keys.COMMAND); a.sendKeys("v"); a.keyUp(Keys.COMMAND); a.build().perform();
如果您使用的是 Windows 机器,则语法为:
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Selenium"); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // Actions class methods to select text Actions a = new Actions(driver); a.keyDown(Keys.CONTROL); a.sendKeys("a"); a.keyUp(Keys.CONTROL); a.build().perform(); // Actions class methods to copy text a.keyDown(Keys.CONTROL); a.sendKeys("c"); a.keyUp(Keys.CONTROL); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(Keys.CONTROL); a.sendKeys("v"); a.keyUp(Keys.CONTROL); a.build().perform();
示例
在 CopyAndPasteActions.java 类文件中实现代码。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class CopyAndPasteAct { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter some text e.sendKeys("Selenium"); // Actions class methods to select text Actions a = new Actions(driver); a.keyDown(Keys.COMMAND); a.sendKeys("a"); a.keyUp(Keys.COMMAND); a.build().perform(); // Actions class methods to copy text a.keyDown(Keys.COMMAND); a.sendKeys("c"); a.keyUp(Keys.COMMAND); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(Keys.COMMAND); a.sendKeys("v"); a.keyUp(Keys.COMMAND); a.build().perform(); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='email']")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
输出
Value copied and pasted: Selenium Process finished with exit code 0
在上面的示例中,我们首先在第一个输入框中输入了文本Selenium,然后将文本复制并粘贴到第二个输入框中,并在控制台中获得了输入文本作为消息 -已复制并粘贴的值:Selenium。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
因此,在本教程中,我们讨论了如何使用 Selenium WebDriver 处理复制和粘贴操作。