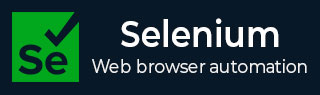
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - Excel 数据文件
Selenium Webdriver 可用于与 Excel 数据文件交互。在自动化测试中,通常需要通过 Excel 文件为测试用例提供大量数据。这样做是为了验证特定场景或创建数据驱动框架。
Java 提供了大量的类和方法,可以使用 Apache POI 库对 Excel 文件执行读写数据操作。Apache POI API 是一组免费且开源的Java 库。
什么是 Apache POI?
Apache POI 用于处理 Microsoft 文件。它可以用于对各种格式的文件(包括 Excel)执行读取、写入、更新和其他操作。Java 不能直接用于处理 Excel 文件,因此 Apache POI(第三方 API)与 Java 一起使用,以创建需要从 Excel 中读取数据的 Selenium 测试。
可以使用 Apache POI 的 Workbook 接口处理 Excel 工作簿。此接口利用 WorkBookFactory 类来生成特定的工作簿。HSSFWorkbook 类(实现 Workbook 接口)具有有助于对具有 .xls 格式的Microsoft Excel 文件执行读写操作的方法。XSSFWorkbook 类(实现 Workbook 接口)具有有助于对具有 .xlsx 或 .xls 格式的 Microsoft Excel 和 OpenOffice XML 文件执行读写操作的方法。
类似地,可以使用 Apache POI 的 Sheet 接口处理 Excel 工作表。HSSFSheet 类(实现 Sheet 接口)具有在 HSSFWorkbook 工作簿(具有 .xls 格式的 Microsoft Excel 文件)中创建工作表的方法。XSSFSheet 类(实现 Sheet 接口)具有在 XSSFWorkbook 工作簿(具有 .xlsx 或 .xls 格式的 Microsoft Excel 和 OpenOffice XML 文件)中创建工作表的方法。
可以使用 Apache POI 的 Row 接口处理 Excel 行。HSSFRow 类(实现 Row 接口)具有表示 HSSFSheet 中行的的方法。XSSFRow 类(实现 Row 接口)具有表示 XSSFSheet 中行的的方法。
可以使用 Apache POI 的 Cell 接口处理 Excel 单元格。HSSFCell 类(实现 Row 接口)具有处理 HSSFRow 中单元格的方法。XSSFCell 类(实现 Row 接口)具有处理 XSSFRow 中单元格的方法。
如何安装 Apache POI?
步骤 1 - 从链接 Apache POI Common 将 Apache POI Common 依赖项添加到 pom.xml 文件。
步骤 2 - 从链接 Apache POI API Based 将基于 OPC 和 OOXML 架构的 Apache POI API 依赖项添加到 pom.xml 文件。
步骤 3 - 保存包含所有依赖项的 pom.xml 并更新Maven 项目。
读取 Excel 中的所有值
让我们以名为 Details.xlsx 的 Excel 文件为例,我们将读取整个 Excel 文件并检索其所有值。
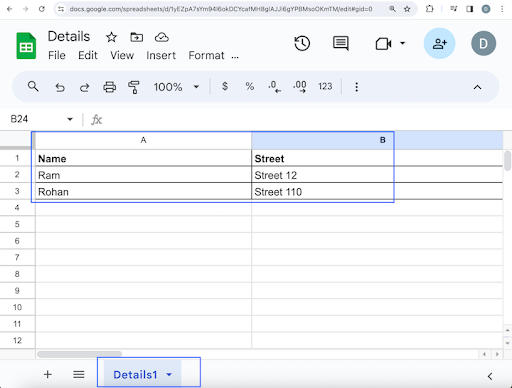
请注意:Details.xlsx 文件放置在项目下的 Resources 文件夹中,如下图所示。
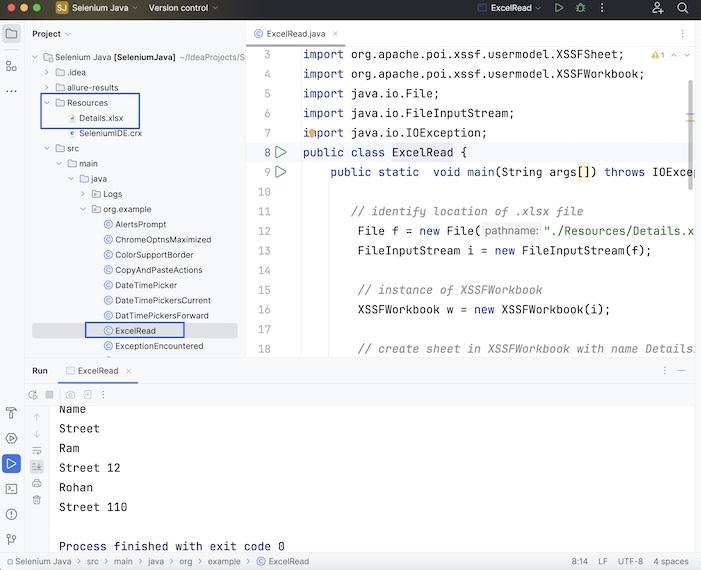
示例
package org.example; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; import java.io.File; import java.io.FileInputStream; import java.io.IOException; public class ExcelRead { public static void main(String args[]) throws IOException { // identify location of .xlsx file File f = new File("./Resources/Details.xlsx"); FileInputStream i = new FileInputStream(f); // instance of XSSFWorkbook XSSFWorkbook w = new XSSFWorkbook(i); // create sheet in XSSFWorkbook with name Details1 XSSFSheet s = w .getSheet("Details1"); // handle total rows in XSSFSheet int r = s.getLastRowNum() - s.getFirstRowNum(); // loop through rows for(int k = 0; k <= r ; k++){ // get cells in each row int c = s.getRow(k).getLastCellNum(); for(int j = 0; j < c; j++){ // get cell values System.out.println(s.getRow(k).getCell(j).getStringCellValue()); } } } }
添加到 pom.xml 的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>5.2.5</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>5.2.5</version> </dependency> </dependencies> </project>
输出
Name Street Ram Street 12 Rohan Street 110 Process finished with exit code 0
在上面的示例中,我们读取了整个 Excel 文件,并在控制台中获得了其所有值。
最后,收到消息 Process finished with exit code 0,表示代码已成功执行。
读取和写入 Excel 中的值
让我们以名为 DetailsStudent.xlsx 的 Excel 文件为例,我们将从该 Excel 文件中读取值,并将这些数据输入到下面的注册页面中。成功后,我们将文本 Test Case: Pass 写入单元格(同一行和 E 列)。如果未成功完成,我们将文本 Test Case: Fail 写入同一单元格。
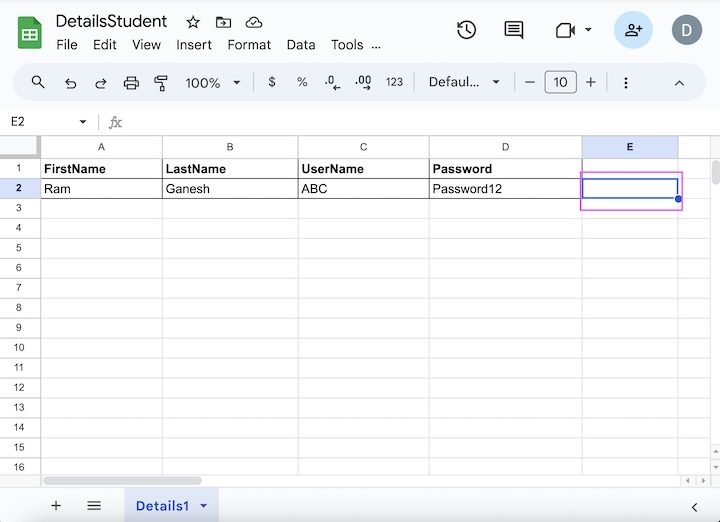
下图显示了我们将从 DetailsStudent.xlsx 文件中在 Full Name:、Last Name:、Username: 和 Password 字段中输入数据的注册页面。
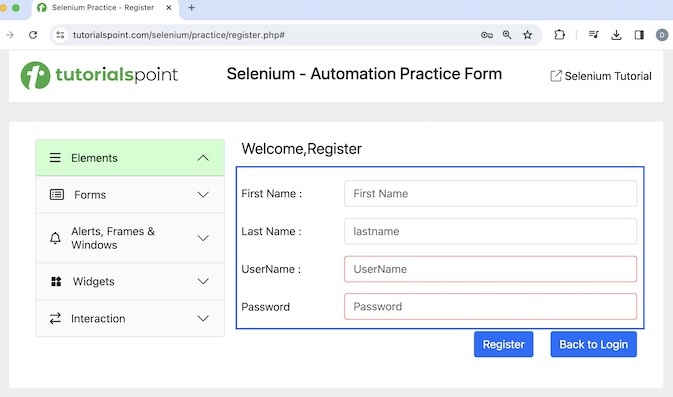
请注意 - DetailsStudent.xlsx Excel 文件放置在项目下的 Resources 文件夹中,如下图所示。
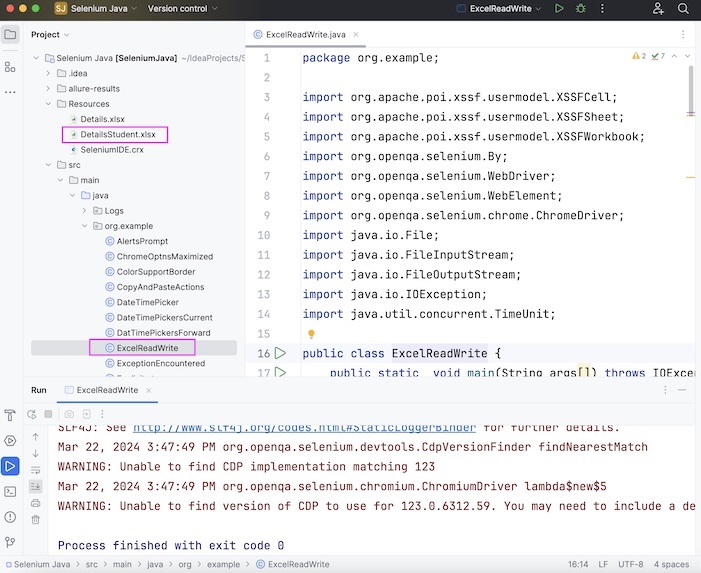
示例
package org.example; import org.apache.poi.xssf.usermodel.XSSFCell; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.concurrent.TimeUnit; public class ExcelReadWrite { public static void main(String args[]) throws IOException { // identify location of .xlsx file File f = new File("./Resources/DetailsStudent.xlsx"); FileInputStream i = new FileInputStream(f); // instance of XSSFWorkbook XSSFWorkbook w = new XSSFWorkbook(i); // create sheet in XSSFWorkbook with name Details1 XSSFSheet s = w .getSheet("Details1"); // handle total rows in XSSFSheet int r = s.getLastRowNum() - s.getFirstRowNum(); // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); // Opening the webpage where we will identify elements driver.get("https://tutorialspoint.com/selenium/practice/register.php#"); //Identify elements for registration WebElement fname = driver.findElement(By.xpath("//*[@id='firstname']")); WebElement lname = driver.findElement(By.xpath("//*[@id='lastname']")); WebElement uname = driver.findElement(By.xpath("//*[@id='username']")); WebElement pass = driver.findElement(By.xpath("//*[@id='password']")); WebElement btn = driver.findElement(By.xpath("//*[@id='signupForm']/div[5]/input")); // loop through rows, read and enter values in form for(int j = 1; j <= r; j++) { fname.sendKeys(s.getRow(j).getCell(0).getStringCellValue()); lname.sendKeys(s.getRow(j).getCell(1).getStringCellValue()); uname.sendKeys(s.getRow(j).getCell(2).getStringCellValue()); pass.sendKeys(s.getRow(j).getCell(3).getStringCellValue()); // submit registration form btn.click(); // verify form submitted WebElement fname1 = driver.findElement(By.xpath("//*[@id='firstname']")); String value = fname1.getAttribute("value"); // create cell at Column 4 to write values in excel XSSFCell c = s.getRow(j).createCell(4); // write results in excel if (value.isEmpty()) { c.setCellValue("Test Case: PASS"); } else { c.setCellValue("Test Case: FAIL"); } // complete writing value in excel FileOutputStream o = new FileOutputStream("./Resources/DetailsStudent.xlsx"); w.write(o); } // closing workbook object w.close(); // Quitting browser driver.quit(); } }
添加到 pom.xml 的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>5.2.5</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>5.2.5</version> </dependency> </dependencies> </project>
输出
Process finished with exit code 0
在上面的示例中,我们读取了整个 Excel 文件,并在第五列的单元格中写入值 Test Case: Pass。
最后,收到消息 Process finished with exit code 0,表示代码已成功执行。
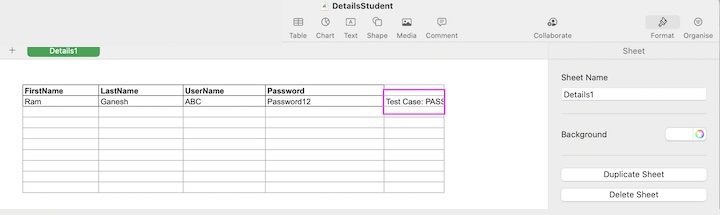
如上图所示,测试运行后,在与Excel中注册数据相同的Excel文件**DetailsStudent.xlsx**的第5列中写入**测试用例:通过**。
结论
本教程全面介绍了Selenium Webdriver Excel数据文件。我们首先介绍了什么是Apache POI,如何安装Apache POI,并通过示例演示了如何在Selenium Webdriver的帮助下使用Apache POI读取和写入Excel中的值。这将使您深入了解Selenium Webdriver中的Excel数据文件。建议您多练习所学内容,并探索与Selenium相关的其他内容,以加深理解并拓宽视野。