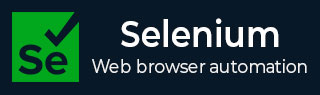
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 颜色支持
Selenium WebDriver可以使用Color类检测网页上web元素的颜色。此外,要检测颜色、背景颜色和边框等特性,可以使用getCssValue()方法。
要获取元素的颜色、背景颜色、边框颜色,需要将它们作为参数传递给getCssValue()方法。它将返回 rgba 格式的值。我们将借助Color类将rgba值转换为十六进制。
识别网页上的元素
启动Chrome浏览器,并在该浏览器上打开一个应用程序。右键单击网页,然后单击“检查”按钮。之后,将显示该页面的整个HTML代码。要识别页面上的元素,请单击HTML代码顶部左侧的向上箭头,如下所示。
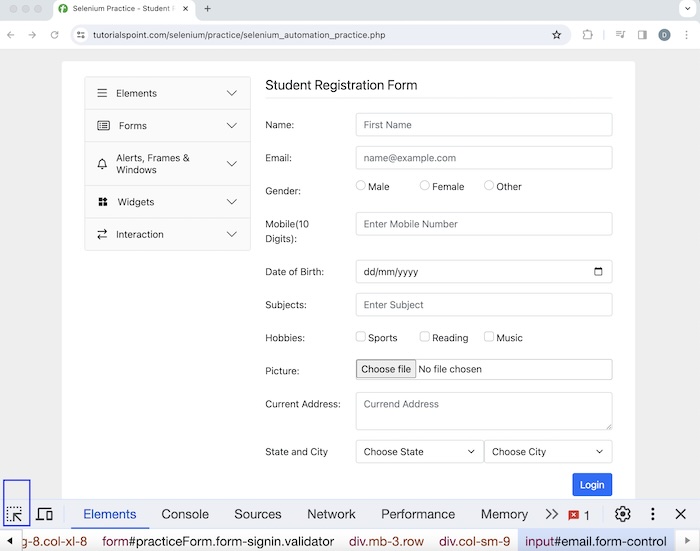
单击并指向元素(突出显示的元素)后,其HTML代码将出现。此外,颜色和背景颜色信息将在样式选项卡中提供。
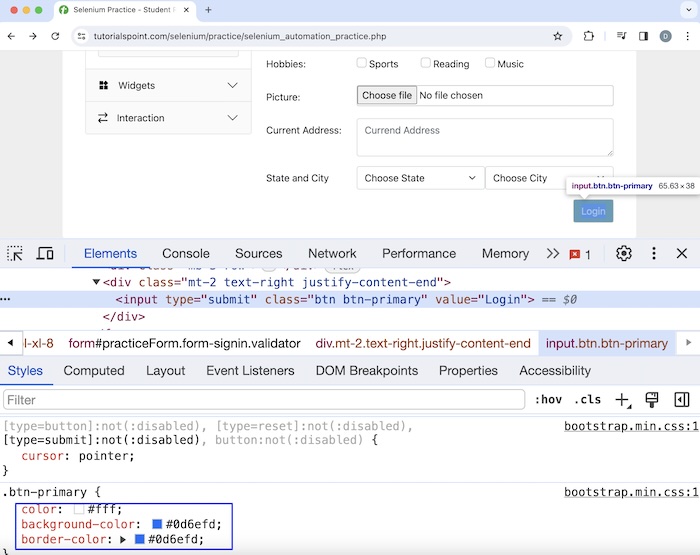
识别元素的背景和颜色
让我们以上面页面上显示的登录按钮为例。在“样式”选项卡中,我们发现其颜色值为#fff,背景颜色为#0d6efd,边框颜色为#0d6efd。让我们使用getCssValue()方法获取该元素的背景颜色和颜色。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.Color; import java.util.concurrent.TimeUnit; public class ColorSupports { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify the element WebElement l = driver.findElement(By.xpath("//*[@id='practiceForm']/div[11]/input")); // get element color in rgba format String s = l.getCssValue("color"); System.out.println("rgba code for color: " + s); // convert rgba to hex using Color class String c = Color.fromString(s).asHex(); System.out.println("Hex format for Element Color is: " + c); // get element background color in rgba format String b = l.getCssValue("background-color"); System.out.println("rgba code for background-color: " + b); // convert rgba to hex using Color class String g = Color.fromString(b).asHex(); System.out.println("Hex format Element Background-Color is: " + g); // Closing browser driver.quit(); } }
输出
rgba code for color: rgba(255, 255, 255, 1) Hex format for Element Color is: #ffffff rgba code for background-color: rgba(13, 110, 253, 1) Hex format Element Background-Color is: #0d6efd Process finished with exit code 0
在上面的例子中,我们以 rgba 格式捕获了按钮的颜色,并在控制台中收到消息 - 颜色的 rgba 代码:rgba(255, 255, 255, 1)。然后将 rgba 格式的颜色转换为十六进制格式,并在控制台中获得消息 - 元素颜色的十六进制格式为:#ffffff。
接下来,我们以 rgba 格式捕获了同一个按钮的背景颜色,并在控制台中收到消息 - 背景颜色的 rgba 代码:rgba(13, 110, 253, 1)。然后将 rgba 格式的背景颜色转换为十六进制格式,并在控制台中获得消息 - 元素背景颜色的十六进制格式为:#0d6efd。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
识别元素的边框颜色
让我们再以前面讨论的同一个登录按钮为例,并使用getCssValue()方法获取边框颜色。
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.Color; import java.util.concurrent.TimeUnit; public class BorderColorSupports { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify the element WebElement l = driver.findElement(By.xpath("//*[@id='practiceForm']/div[11]/input")); // get element border color in rgba format String s = l.getCssValue("border-color"); System.out.println("rgba code for border color: " + s); // convert rgba to hex using Color class String g = Color.fromString(s).asHex(); System.out.println("Hex format for element Border-Color is: " + g); // Closing browser driver.quit(); } }
在pom.xml文件中添加的依赖项:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
输出
rgba code for border color: rgb(13, 110, 253) Hex format for element Border-Color is: #0d6efd
在上面的例子中,我们以 rgba 格式捕获了按钮的边框颜色,并在控制台中收到消息 - 颜色的 rgba 代码:rgba(13, 110, 253)。然后将 rgba 格式的颜色转换为十六进制格式,并在控制台中获得消息 - 元素边框颜色的十六进制格式为:#0d6efd。
结论
本教程对 Selenium WebDriver 颜色支持进行了全面讲解。我们首先介绍了如何在网页上识别元素,然后通过示例演示了如何使用 Selenium WebDriver 识别元素的边框颜色、背景颜色和颜色。这使您能够深入了解 Selenium WebDriver 颜色支持。最好继续练习您所学到的知识,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。