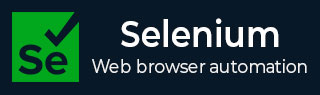
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览器上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 基于关键字的框架
- Selenium - 数据驱动的框架
- Selenium - 混合驱动的框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 浏览器导航
Selenium Webdriver 提供多种方法来实现浏览器导航,包括启动、后退、前进浏览器历史记录以及刷新浏览器。
基本的浏览器导航命令
下面讨论一些浏览器导航方法:
driver.navigate().to("应用程序 URL")
此方法导航到应用程序 URL。
语法
driver.navigate().to("https://tutorialspoint.com/index.htm");
driver.get("应用程序 URL")
此方法启动浏览器以打开应用程序。
语法
driver.get("https://tutorialspoint.com/index.htm");
driver.navigate().back()
此方法根据浏览器历史记录上下文跳转到上一页。
语法
driver.navigate().back();
driver.navigate().forward()
此方法根据浏览器历史记录上下文跳转到下一页。
语法
driver.navigate().forward();
driver.navigate().refresh()
此方法重新加载网页。
语法
driver.navigate().refresh();
示例 1
让我们来看一个例子,我们首先启动一个浏览器标题为Selenium Practice - Student Registration Form的应用程序。

然后,我们将点击登录链接,点击后,我们将导航到另一个浏览器标题为Selenium Practice - Login的页面。

接下来,我们将返回浏览器历史记录,并将浏览器标题获取为Selenium Practice - Student Registration Form。
最后,我们将前进到浏览器历史记录,并将浏览器标题获取为Selenium Practice - Login。我们还将刷新浏览器,并将浏览器标题获取为Selenium Practice - Login。
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class BrowNavigation { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Getting browser title after launch System.out.println("Getting browser title after launch: " + driver.getTitle()); // identify the link then click WebElement l = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a")); l.click(); // Getting browser title after clicking link System.out.println("Getting browser title after clicking link: " + driver.getTitle()); // navigate back to browser history driver.navigate().back(); // Getting browser title after navigating back System.out.println("Getting browser title after navigating back: " + driver.getTitle()); // navigate forward to browser history driver.navigate().forward(); // Getting browser title after navigating forward System.out.println("Getting browser title after navigating forward: " + driver.getTitle()); // refresh browser driver.navigate().refresh(); // Getting browser title after browser refresh System.out.println("Getting browser title after browser refresh: " + driver.getTitle()); // Closing browser driver.quit(); } }
输出
Getting browser title after launch: Selenium Practice - Student Registration Form Getting browser title after clicking link: Selenium Practice - Login Getting browser title after navigating back: Selenium Practice - Student Registration Form Getting browser title after navigating forward: Selenium Practice - Login Getting browser title after browser refresh: Selenium Practice - Login
在上面的示例中,我们在打开的浏览器中启动了一个 URL,并在控制台中获得了浏览器标题消息 - 启动后获取浏览器标题:Selenium Practice - Student Registration Form。然后我们点击了登录链接,并收到了导航页面的浏览器标题,控制台消息为 - 点击链接后获取浏览器标题:Selenium Practice - Login。
接下来,我们在浏览器历史记录中后退,并获得了浏览器标题,控制台消息为 - 导航后退后获取浏览器标题:Selenium Practice - Student Registration Form。再次,我们在浏览器历史记录中前进,并检索了浏览器标题,控制台消息为 - 导航前进后获取浏览器标题:Selenium Practice - Login。最后,我们刷新了浏览器并获得了它的标题,控制台消息为 - 浏览器刷新后获取浏览器标题:Selenium Practice - Login。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 2
让我们来看一个例子,我们首先启动一个 URL 为 - 的应用程序:
https://tutorialspoint.com/selenium/practice/login.php.

然后,我们将点击注册链接,点击后,我们将导航到另一个 URL 为 - 的页面:
https://tutorialspoint.com/selenium/practice/register.php.

接下来,我们将返回浏览器历史记录,并将 URL 获取为 -
https://tutorialspoint.com/selenium/practice/login.php.
最后,我们将前进到浏览器历史记录,并将 URL 获取为 -
https://tutorialspoint.com/selenium/practice/register.php.
我们还将刷新浏览器,刷新后我们将获得 URL 为
https://tutorialspoint.com/selenium/practice/register.php.
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class BrowNavigationTo { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.navigate().to("https://tutorialspoint.com/selenium/practice/login.php"); // Getting browser title after launch System.out.println("Getting current URL after launch: " + driver.getCurrentUrl()); // identify a link then click WebElement l = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[3]/a")); l.click(); // Getting browser title after clicking link System.out.println("Getting current URL after clicking link: " + driver.getCurrentUrl()); // navigate back to browser history driver.navigate().back(); // Getting browser title after navigating back System.out.println ("Getting current URL after navigating back: " + driver.getCurrentUrl()); // navigate forward to browser history driver.navigate().forward(); // Getting browser title after navigating forward System.out.println("Getting current URL after navigating forward: " + driver.getCurrentUrl()); // refresh browser driver.navigate().refresh(); // Getting browser title after browser refresh System.out.println("Getting current URL after browser refresh: " + driver.getCurrentUrl()); // Closing browser driver.quit(); } }
输出
Getting current URL after launch: https://tutorialspoint.com/selenium/practice/login.php Getting current URL after clicking link: https://tutorialspoint.com/selenium/practice/register.php Getting current URL after navigating back: https://tutorialspoint.com/selenium/practice/login.php Getting current URL after navigating forward: https://tutorialspoint.com/selenium/practice/register.php Getting current URL after browser refresh: https://tutorialspoint.com/selenium/practice/register.php
在上面的示例中,我们在打开的浏览器中启动了一个 URL,并在控制台中获得了当前 URL 消息 - 启动后获取当前 URL: https://tutorialspoint.com/selenium/practice/login.php。
然后我们点击了注册链接,并在控制台中收到了导航后的当前 URL 消息 - 点击链接后获取当前 URL: https://tutorialspoint.com/selenium/practice/register.php。
接下来,我们在浏览器历史记录中后退,并在控制台中获得了导航后的当前 URL 消息 - 导航后退后获取当前 URL: https://tutorialspoint.com/selenium/practice/login.php。
再次,我们在浏览器历史记录中前进,并在控制台中收到了导航后的当前 URL 消息 - 导航前进后获取当前 URL: https://tutorialspoint.com/selenium/practice/register.php。
最后,我们刷新了浏览器,并在控制台中收到了当前 URL 消息 - 浏览器刷新后获取当前 URL: https://tutorialspoint.com/selenium/practice/register.php。
因此,在本教程中,我们讨论了如何使用 Selenium Webdriver 执行浏览器导航。