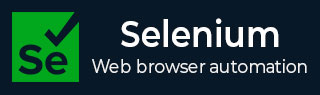
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 杂项概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - Action 类
Selenium Webdriver 可用于执行键盘和鼠标操作。这包括诸如拖放、单击、双击、右键单击、使用控制键、其他键操作等操作。所有这些操作都是通过指定的用户交换 API 执行的,在 Selenium Webdriver 中称为 Actions 类。
Action 类方法
下面列出了 Actions 类中的一些可用方法:
- click() - 此方法用于在鼠标当前位置执行单击。
- build() - 此方法用于创建一个包含所有要执行的操作的动作组合。
- perform() - 此方法用于执行操作,而无需先调用 build()。
- release() - 此方法用于在鼠标当前位置释放鼠标操作。
- release(WebElement e) - 此方法用于在作为参数传递的 webElement e 的中间释放鼠标操作。
- doubleClick(WebElement e) - 此方法用于在作为参数传递的 webElement e 的中间执行双击。
- click(WebElement e) - 此方法用于在作为参数传递的 webElement e 的中间执行单击。
- doubleClick() - 此方法用于在鼠标当前位置执行双击。
- contextClick() - 此方法用于在鼠标当前位置执行右键单击。
- contextClick(WebElement e) - 此方法用于在作为参数传递的 webElement e 的中间执行右键单击。
- moveToElement(WebElement e) - 此方法用于将鼠标移动到作为参数传递的 webElement e 的中间。
- moveToElement(WebElement e, int x-offset, int y-offset) - 此方法用于将鼠标移动到视点中元素的偏移位置。webElement e、x 和 y 偏移值作为参数传递。
- clickAndHold() - 此方法用于在鼠标当前位置执行单击(不释放)。
- clickAndHold(WebElement e) - 此方法用于在作为参数传递的 webElement e 的中间执行单击(不释放)。
- keyDown(CharSequence key) - 此方法用于执行修饰键按下,作为参数传递。
- keyDown(WebElement e, CharSequence key) - 此方法用于在聚焦元素后执行修饰键按下。webElement e 和要按下的键作为参数传递。
- keyUp(CharSequence key) - 此方法用于执行修饰键释放,作为参数传递。
- keyUp(WebElement e, CharSequence key) - 此方法用于在聚焦元素后执行修饰键释放。webElement e 和要释放的键作为参数传递。
- sendKeys(CharSequence key) - 此方法用于将键发送到焦点元素。要发送的键作为参数传递。
- sendKeys(WebElement e, CharSequence key) - 此方法用于将键发送到作为参数传递的 webElement。
请注意,在使用 Actions 类的这些方法时,需要添加导入语句:
import org.openqa.selenium.interactions.Actions in our tests.
示例 1 - 单击、右键单击和双击
让我们以以下页面为例,我们将通过分别单击网页上的单击我、右键单击我和双击我按钮来执行单击、右键单击和双击。
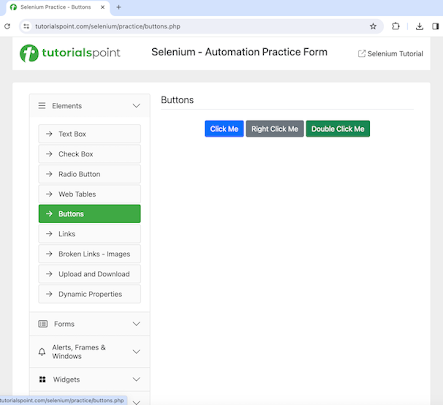
在分别执行单击我、右键单击我和双击我按钮后,我们将在页面上分别收到消息您有一个动态点击和您已双击。
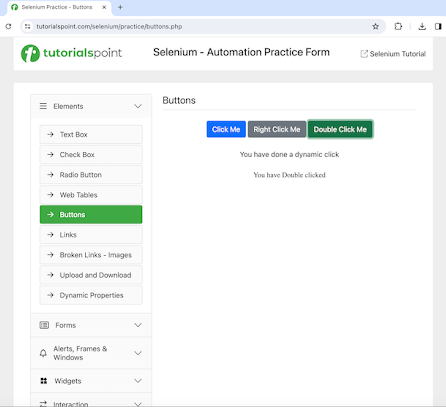
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class ActionsMouse { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/buttons.php"); // identify element with xpath for click WebElement m = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/button[1]")); // object of Actions class to move then click Actions a = new Actions(driver); a.moveToElement(m).click().build().perform(); // get text after click WebElement t = driver.findElement(By.xpath("//*[@id='welcomeDiv']")); System.out.println("Text after click: " + t.getText()); // identify element with xpath for double click WebElement n = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/button[3]")); // double click a.moveToElement(n).doubleClick().build().perform(); // get text after double click WebElement x = driver.findElement(By.xpath("//*[@id='doublec']")); System.out.println("Text after double click: " + x.getText()); // identify element with xpath for right click WebElement y = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/button[2]")); // right click a.moveToElement(y).contextClick().build().perform(); // Closing browser driver.quit(); } }
输出
Text after click: You have done a dynamic click Text after double click: You have Double clicked Process finished with exit code 0
在上面的示例中,我们执行了单击、双击和右键单击,然后在控制台中收到了消息 - 单击后的文本:您已执行动态单击和双击后的文本:您已双击。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 2 - 鼠标悬停
让我们再举一个例子,最初菜单Navbar为黑色。
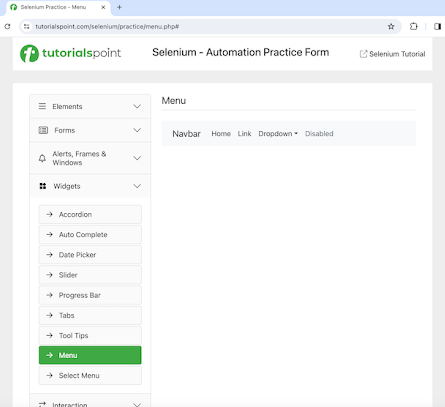
但是,当悬停并按住它时,菜单 Navbar 的颜色从黑色变为绿色,如下面的图像所示。
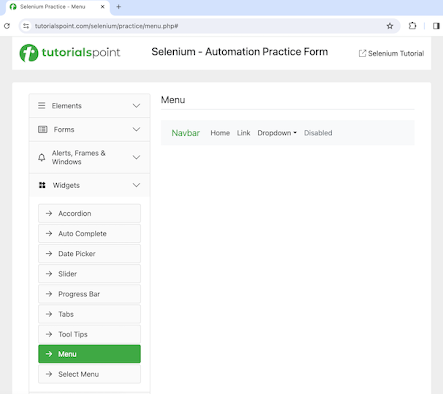
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class ActionsClickandHold { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/menu.php#"); // identify element with xpath for click and hold WebElement m = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/nav/div/a")); // get element color in rgba format String s = m.getCssValue("color"); System.out.println("rgba code for color element: " + s ); // object of Actions class to click and hold Actions a = new Actions(driver); a.clickAndHold(m).build().perform(); // get element color in rgba format String c = m.getCssValue("color"); System.out.println("rgba code for color for element after click and hold: " + c); // Closing browser driver.quit(); } }
输出
rgba code for color element: rgba(51, 51, 51, 1) rgba code for color for element after click and hold: rgba(64, 169, 68, 1) Process finished with exit code 0
示例 3 - 拖放
让我们以以下页面为例,我们将把带有文本将我拖到我的目标的源框拖到带有文本在此处放置的目标框。
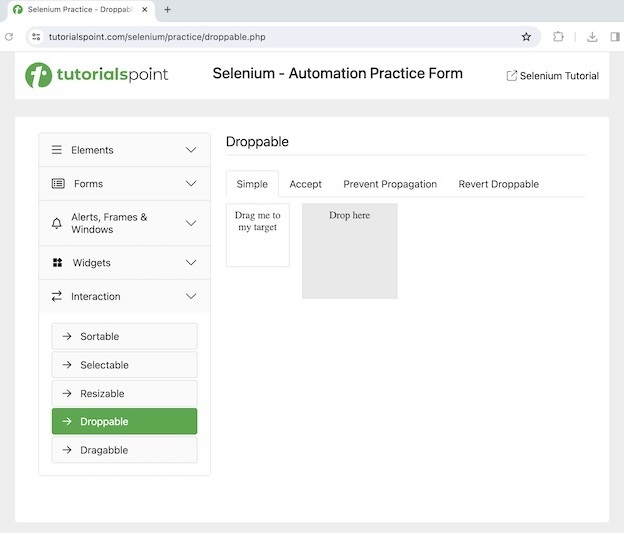
一旦整个操作完成,我们将在网页上获得文本已放置!,如下面的图像中突出显示的那样。
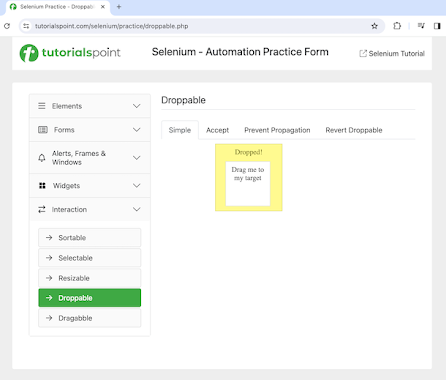
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class DragAndDrp { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // URL launch for accessing drag and drop elements driver.get("https://tutorialspoint.com/selenium/practice/droppable.php"); // identify source and target elements for drag and drop WebElement sourceElement= driver.findElement(By.id("draggable")); WebElement targetElement= driver.findElement(By.id("droppable")); // drag and drop operations without build and perform methods Actions a = new Actions(driver); a.dragAndDrop(sourceElement, targetElement).build().perform(); // identify text after element is dropped WebElement text = driver.findElement(By.xpath("//*[@id='droppable']/p")); System.out.println("Text is : " + text.getText()); // quitting browser after drag and drop operations completed driver.quit(); } }
输出
Text is after dragging: Dropped! Process finished with exit code 0
在上面的示例中,我们首先从源定位器到目标定位器执行了拖放操作,并且还在控制台中收到了消息 - 文本为:已放置!(在本文中,此应用程序中的拖放操作完成后会收到此消息)。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 4 - 键操作
让我们再举一个例子,我们将在输入框中使用 Action 类的 Key Up Key Down 方法输入大写字母的文本SELENIUM。请注意,在将值发送到 sendKeys() 方法时,我们将传递selenium 以及按下 SHIFT 键。因此,我们将在输入框中获得输入的输出为SELENIUM。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class KeyAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // Actions class Actions a = new Actions(driver); // moving to an input box and clicking on it a.moveToElement(e).click(); // key UP and DOWN action for SHIFT a.keyDown(Keys.SHIFT); a.sendKeys("Selenium").keyUp(Keys.SHIFT).build().perform(); // get value entered System.out.println("Text entered: " + e.getAttribute("value")); // Closing browser driver.quit(); } }
输出
Text entered: SELENIUM Process finished with exit code 0
在上面的例子中,我们在输入框中输入了文本selenium 以及按下 SHIFT 键,因此得到了大写的输入文本,并在控制台中显示消息 - Text entered: SELENIUM。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
结论
本教程到此结束,我们全面介绍了 Selenium Webdriver Action 类。我们从描述 Action 类开始,逐步讲解了 Action 类的各种方法,并通过示例说明了如何执行点击、右键点击、双击、鼠标悬停、拖放以及与 Selenium 结合的按键操作。这使您能够深入了解 Action 类。建议您多练习所学内容,并探索其他与 Selenium 相关的知识,以加深理解并拓宽视野。